ORM stands for Object-Relational Mapping, which maps between Object Model and a Relational Database like MySQL, PostgreSQL, MS SQL, SQLite, etc.
ODM is the abbreviation for Object Data Model that maps between an Object Model and a Document Database (NoSQL) like MongoDB, Amazon DynamoDB, etc.
ORMs and ODMs are powerful tools that can help us a lot when building and maintaining backend applications that use databases. This article covers the best and most-used open-source ORM and ODM libraries for Node.js. The main criteria here are the stars they get from GitHub’s users and the number of weekly installs on npmjs. Without any further ado, let’s explore the list.
Table of Contents
TypeORM
- GitHub stars: 33k+
- NPM weekly downloads: 1.5m – 2m
- License: MIT
- Written in: TypeScript
- Links: GitHub repo | NPM page | Official website
- Supported databases: MySQL, MariaDB, Postgres, CockroachDB, SQLite, Microsoft SQL Server, Oracle, SAP Hana, sql.js, and MongoDB NoSQL database
TypeORM, as its name implies, is a big and comprehensive ORM library that can run not only in Node.js but also in many other platforms, including Ionic, React Native, Browser, Cordova, Expo, and Electron. It works fine with the latest versions of modern JavaScript and provides many extra features that help you to develop any kind of application that uses databases.
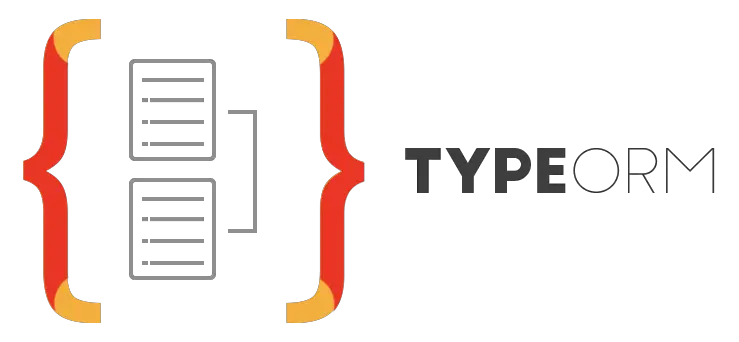
Key features:
- Supports both DataMapper and ActiveRecord
- Supports both SQL and NoSQL databases
- Transactions
- Migrations and automatic migrations generation
- Connection pooling
- Replication
- Using multiple database connections
- Working with multiple databases types
- Cross-database and cross-schema queries
- Pagination for queries using joins
Installing:
npm i typeorm
Example (with TypeScript):
import { Entity, PrimaryGeneratedColumn, Column } from "typeorm";
@Entity()
export class Item {
@PrimaryGeneratedColumn()
id: number;
@Column()
name: string;
@Column()
price: number;
}
Mongoose (ODM)
- GitHub stars: 26.5k+
- NPM weekly downloads: 2.2m – 2.7m
- License: MIT
- Written in: Javascript
- Links: GitHub repo | NPM page | Official website
- Supported databases: MongoDB
Mongoose has come to life for more than a decade. It is currently the most popular MongoDB object modeling tool in the Node.js world. The library is regularly updated and improved to take advantage of the new features of the latest versions of MongoDB. It is also well documented, and there are hundreds of plugins made by the community to extend the core API.
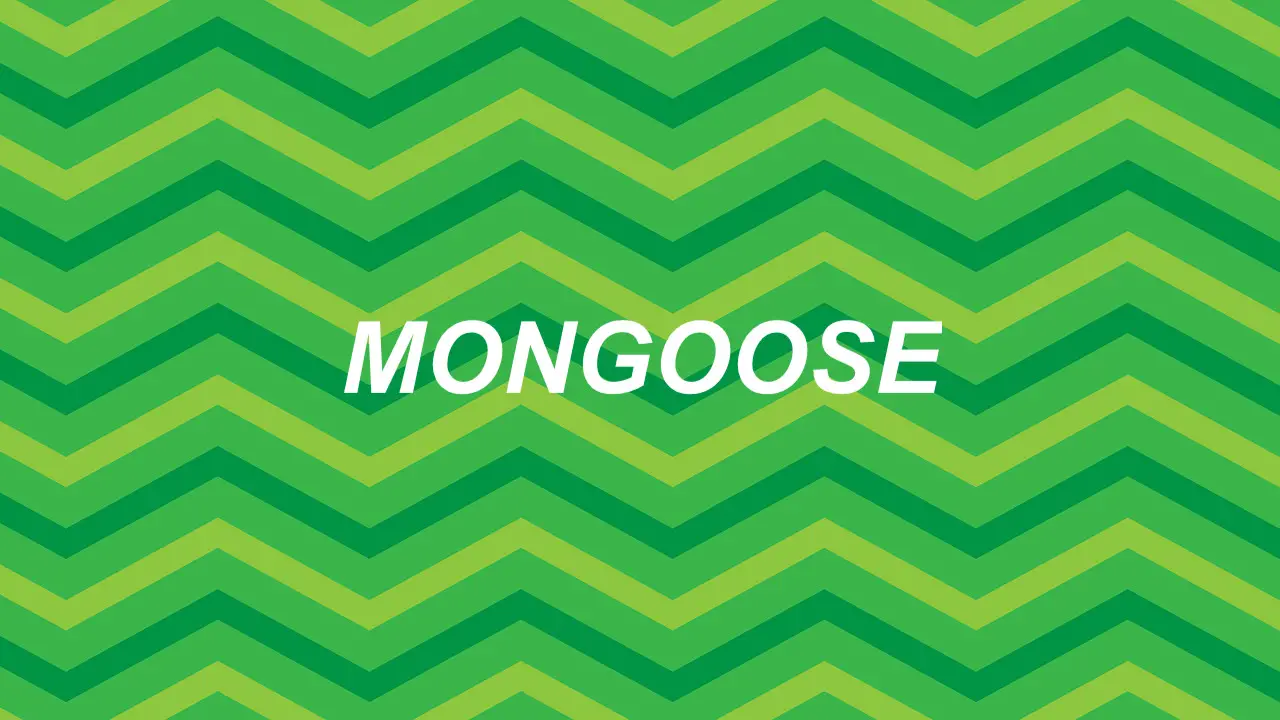
Installing:
npm i mongoose
Example:
const mongoose = require('mongoose');
mongoose.connect('mongodb://localhost:27017/my_shop');
const Cat = mongoose.model('Product', { name: String, price: Number });
const book = new Product({ name: 'Unknown Novel', price: 10.99 });
book.save().then(() => console.log('Done'));
Prisma
- GitHub stars: 36k+
- NPM weekly downloads: 2m – 2.5m
- License: Apache-2.0
- Written in: TypeScript, Javascript
- Links: GitHub repo | NPM page | Official website
- Supported databases: PostgreSQL, MySQL, SQL Server, SQLite, and MongoDB
Prisma is a young, modern, and rapid-growing Node.js ORM. The provides an intuitive and declarative way to define data models and relations that are very friendly and easy to read.
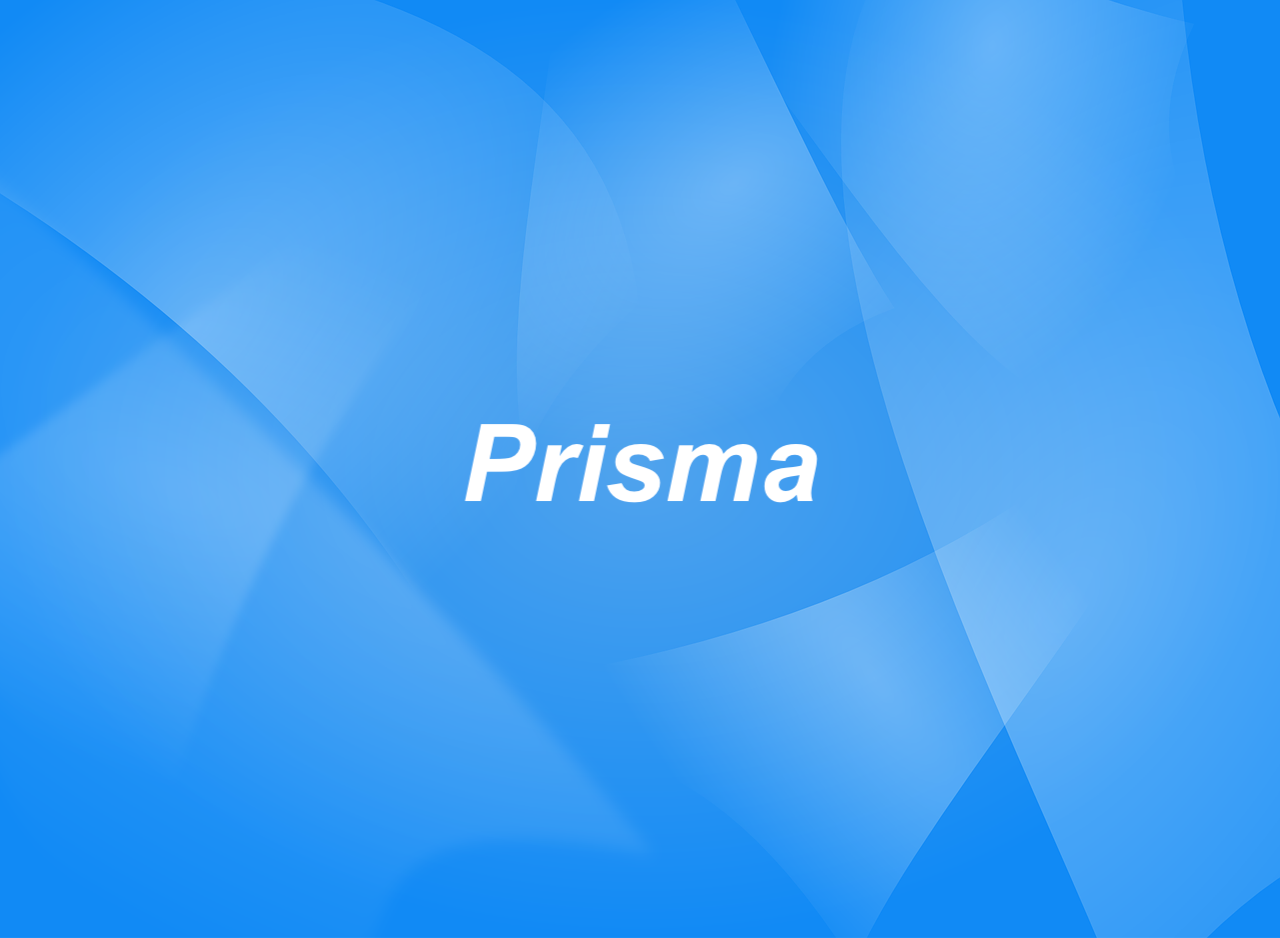
Installing Prisma CLI:
npm i prisma
Example of defining a model with Prisma:
model User {
id Int @id @default(autoincrement())
createdAt DateTime @default(now())
email String @unique
name String?
}
Sequelize
- GitHub stars: 29k+
- NPM weekly downloads: 1.6m – 2m
- License: MIT
- Written in: Javascript, TypeScript
- Links: GitHub repo | NPM page | Official website
- Supported databases: Postgres, MySQL, MariaDB, SQLite, MS SQL Server
Like Mongoose, Sequelize is not young anymore since it was born more than 10 years ago. However, while Mongoose only supports MongoDB, Sequelize only works with SQL databases.
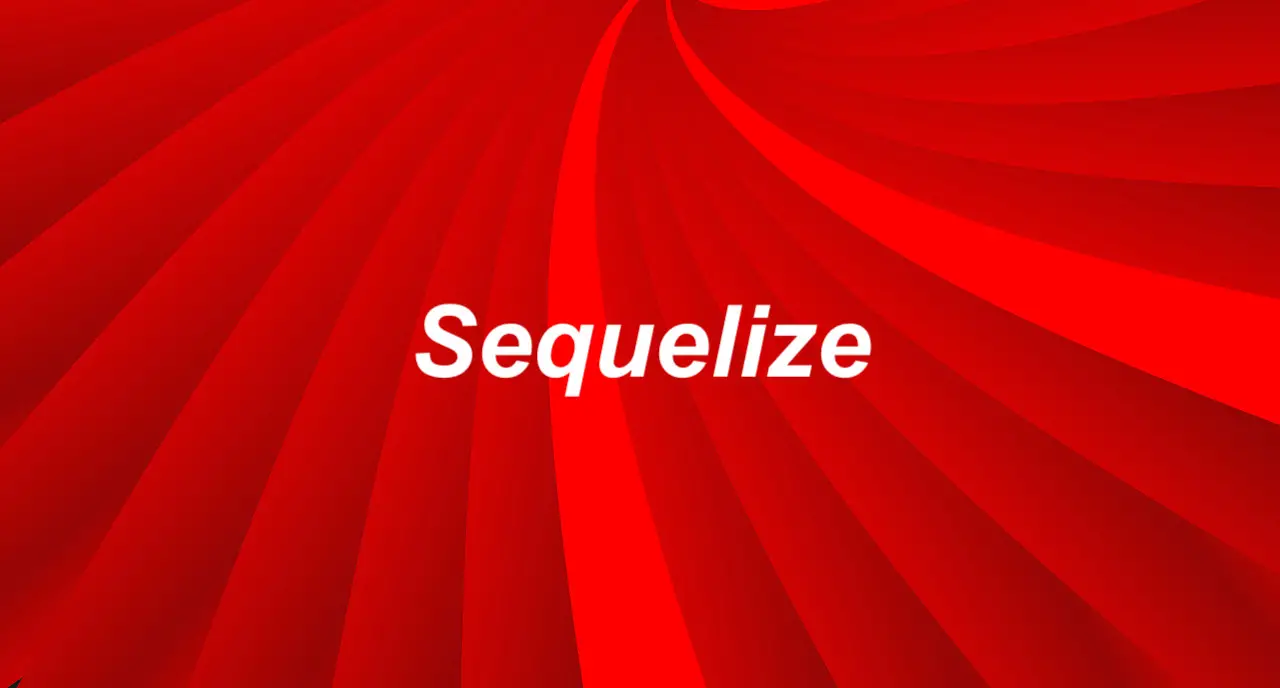
Installing:
npm i sequelize
The example below defines a User model:
const { Sequelize, DataTypes } = require('sequelize');
const sequelize = new Sequelize('sqlite::memory:');
const User = sequelize.define('User', {
// Model attributes
firstName: {
type: DataTypes.STRING,
allowNull: false
},
lastName: {
type: DataTypes.STRING
}
}, {
// Other options
});
Objection
- GitHub stars: 7.2k+
- NPM weekly downloads: 110k – 150k
- License: MIT
- Written in: Javascript, TypeScript
- Links: GitHub repo | NPM page
- Supported databases: All popular SQL databases (MySQL, Postgres, SQLite3, etc)
Objection.js is a lightweight ORM for Node.js that strongly focuses on making things easier and more enjoyable. It provides an SQL query builder that comes along with a powerful set of tools for working with relations.
Key features:
- Simple and fun CRUD operations
- Defines models with ease
- Easy-to-use transactions
- Supports TypeScript out of the box
- Optional JSON schema validation
- Storing a complex document as a single row
Installing:
npm i objection
Example:
const { Model } = require('objection');
class Person extends Model {
static get tableName() {
return 'persons';
}
static get jsonSchema() {
return {
type: 'object',
required: ['firstName', 'lastName'],
properties: {
id: { type: 'integer' },
parentId: { type: ['integer', 'null'] },
firstName: { type: 'string', minLength: 1, maxLength: 255 },
lastName: { type: 'string', minLength: 1, maxLength: 255 },
age: { type: 'number' },
address: {
type: 'object',
properties: {
street: { type: 'string' },
city: { type: 'string' },
zipCode: { type: 'string' },
},
},
},
}
}
}
module.exports = {
Person,
};
Bookshelf
- GitHub stars: 6.3k+
- NPM weekly downloads: 80k – 120k
- License: MIT
- Written in: Javascript
- Links: GitHub repo | NPM page | Official website
- Supports PostgreSQL, MySQL, and SQLite3
Bookshelf.js is an open-source ORM for Node.js with both promise-based and old-fashioned callback interfaces. It is super neat and concise, with a total unpacked size of only around 300 kB. The foundation that Bookshelf was built on is Knex.js, a famous SQL query builder.
To use Bookshelf, you have to install it and Knex:
npm install knex bookshelf mysql
// You can replace mysql with pg or sqlite3
Bookshelf.js can be used with a bunch of plugins to add new features to the core library. You can see the full list here.
Wrapping Up
We’ve walked through a list of the best ORMs and ODMs for Node.js these days. Which one do you love most and will appear in your next project?
Node.js is awesome, and there are many things to learn about it. If you’d like to explore more about this Javascript runtime, then take a look at the following articles:
- Node.js: 2 Ways to Colorize Console Log Output
- 4 Ways to Convert Object into Query String in Node.js
- Node.js: Get domain, hostname, and protocol from a URL
- Top best Node.js Open Source Headless CMS
- Best Node.js frameworks to build backend APIs
- Best Open-Source HTTP Request Libraries for Node.js
You can also check out our Node.js category page for the latest tutorials and examples.