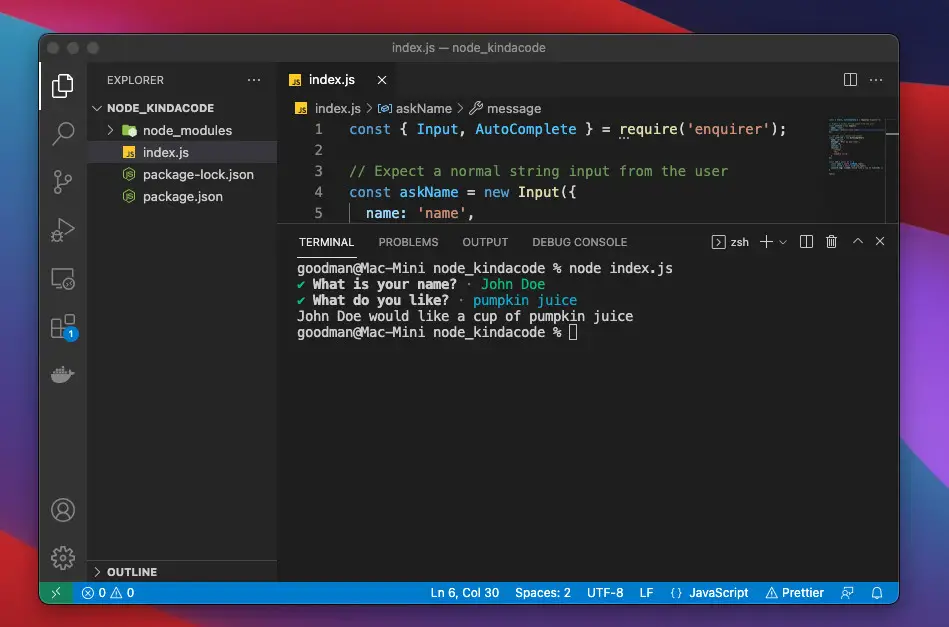
This article walks you through a couple of different ways to get user input from the console in a Node.js program. The first approach is to do things from scratch with only built-in features of Node.js. The second one is to archive the goal quickly by using a third-party library. Without any further ado, let’s get our hands dirty.
Using self-written code
In the following example, we’ll use readline, a standard module of Node.js core.
Example Preview
The Code
// CommonJS require
const readline = require('readline')
// if you use ES Modules, use this:
// import readline from 'readline'
const inquirer = readline.createInterface({
input: process.stdin,
output: process.stdout
});
inquirer.question("What is your name ?", name => {
inquirer.question("How old are you?", age => {
console.log(`${name} is ${age} years old`);
inquirer.close();
});
});
inquirer.on("close", function() {
console.log("Good bye!");
process.exit(0);
});
Using a 3rd library
There are many great packages for inquiring the user through the command line. The most popular ones are Inquirer.js, Enquirer, Prompts, Prompt (without an “s”). The example below will make use of Enquirer (which has more than 13 million weekly downloads via npm) to create a beautiful, stylish, and intuitive prompt.
Example Preview
In this example, you’ll see how to create a multi-choice question with Enquirer. The use can use the Up Arrow and Down Arrow keys to select an answer.
The Code
Installing:
npm i enquirer
The full code in index.js:
// CommonJS
const { Input, AutoComplete } = require('enquirer');
// ES Modules
// import { Input, AutoComplete } from 'enquirer';
// Expect a normal string input from the user
const askName = new Input({
name: 'name',
message: 'What is your name?'
});
// Let the user choose one answer
const askDrink = new AutoComplete({
name: 'drink',
message: 'What do you like?',
limit: 10,
initial: 2,
choices: [
'coffee',
'tea',
'pumpkin juice',
]
});
const run = async () => {
const name = await askName.run();
const drink = await askDrink.run();
console.log(`${name} would like a cup of ${drink}`);
}
run();
Conclusion
You’ve learned a few techniques to prompt the user for their input through the console in Node.js. If you’d like to explore more new and awesome things about the Javascript runtime, then take a look at the following articles:
- Node.js: Use Async Imports (Dynamic Imports) with ES Modules
- Node.js: Generate Images from Text Using Canvas
- Node.js: Reading and Parsing Excel (XLSX) Files
- Best open-source ORM and ODM libraries for Node.js
- 7 Best Open-Source HTTP Request Libraries for Node.js
- Node.js: Get domain, hostname, and protocol from a URL
You can also check out our Node.js category page for the latest tutorials and examples.