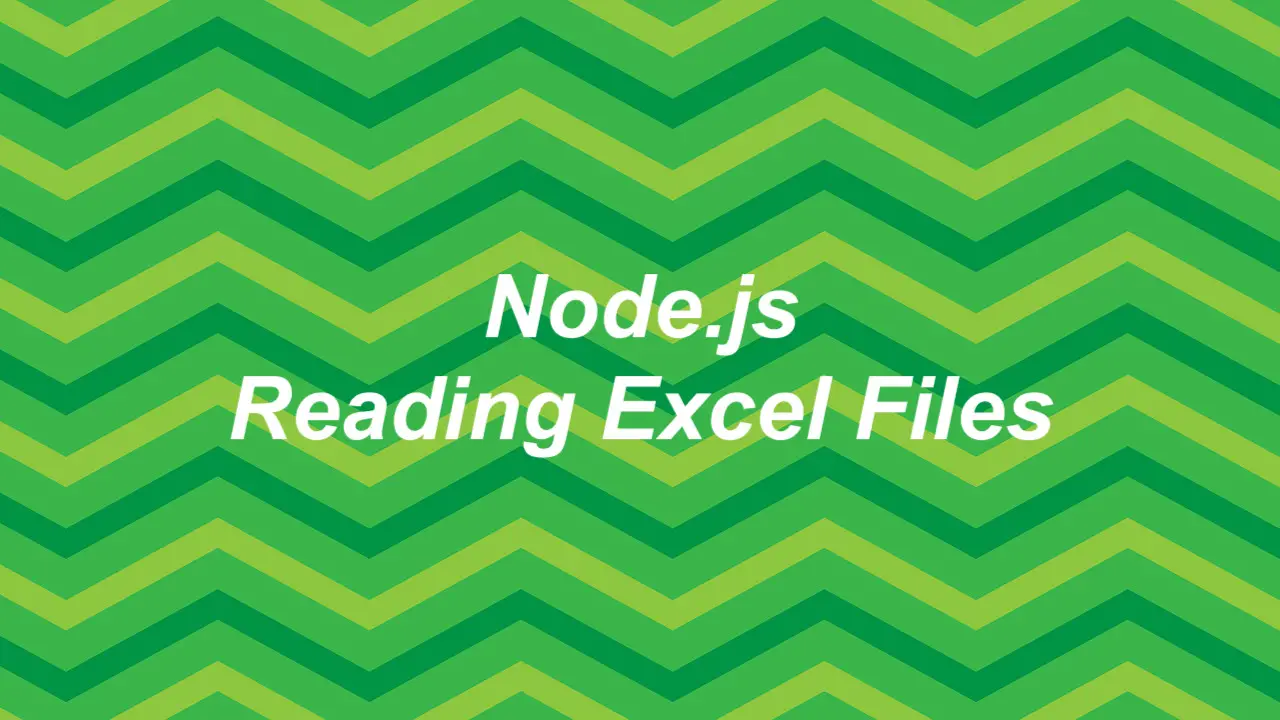
This article shows you how to read and extract content from an Excel (.xlsx) file by using Node.js. Without any further ado, let’s get our hands dirty and start coding.
Getting Things Ready
We are going to work with a simple Excel file that contains some information as follows:
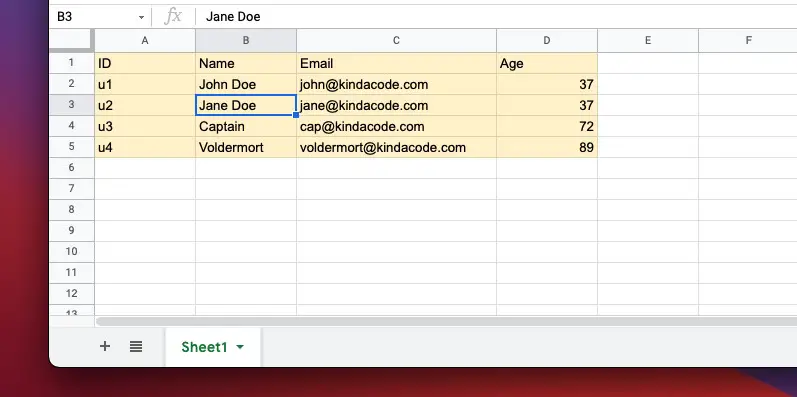
You can download the file from the link below to your computer:
https://www.kindacode.com/wp-content/uploads/2021/11/KindaCode.com-Example.xlsx.zip
Writing Code
There are many great libraries that can help us easily read Excel files, such as xlsx (SheetJS), exceljs (ExcelJS), node-xlsx (Node XLSX). In the sample project below, we will use xlsx. It’s super popular and supports TypeScript out-of-the-box.
1. Create a new folder named example (the name doesn’t matter and is totally up to you), then initialize a new Node.js project by running:
npm init
2. In your project directory, create a subfolder called src, then add an empty index.js file. Copy the Excel file you’ve downloaded before into the src folder.
Here’s the file structure:
.
├── node_modules
├── package-lock.json
├── package.json
└── src
├── KindaCode.com Example.xlsx
└── index.js
3. Installing the xlsx package:
npm i xlsx
4. Below are the code snippets for index.js. There are 2 different code snippets. The first one uses the CommonJS syntax (with require), while the second one uses the ES Modules syntax (with import). Choose the one that fits your need.
CommonJS:
const path = require("path");
const xlsx = require("xlsx");
const filePath = path.resolve(__dirname, "Kindacode.com Example.xlsx");
const workbook = xlsx.readFile(filePath);
const sheetNames = workbook.SheetNames;
// Get the data of "Sheet1"
const data = xlsx.utils.sheet_to_json(workbook.Sheets[sheetNames[0]])
/// Do what you need with the received data
data.map(person => {
console.log(`${person.Name} is ${person.Age} years old`);
})
ES Modules:
// import with ES6 Modules syntax
import path from 'path';
import xlsx from 'xlsx';
import { fileURLToPath } from 'url'
import { dirname } from 'path'
const __filename = fileURLToPath(import.meta.url)
const __dirname = dirname(__filename)
const filePath = path.resolve(__dirname, "Kindacode.com Example.xlsx");
const workbook = xlsx.readFile(filePath);
const sheetNames = workbook.SheetNames;
// Get the data of "Sheet1"
const data = xlsx.utils.sheet_to_json(workbook.Sheets[sheetNames[0]])
/// Do what you need with the received data
data.map(person => {
console.log(`${person.Name} is ${person.Age} years old`);
})
5. Test our project:
node src/index.js
Output:
John Doe is 37 years old
Jane Doe is 37 years old
Captain is 72 years old
Voldermort is 89 years old
Conclusion
We’ve written a simple Node.js program to retrieve the data from a sheet of an Excel workbook. If you’d like to explore more new and interesting things about modern Node.js, take a look at the following articles:
- Node.js: How to Ping a Remote Server/ Website
- Best open-source ORM and ODM libraries for Node.js
- 7 Best Open-Source HTTP Request Libraries for Node.js
- Node.js: Executing a Piece of Code after a Delay
- Express + TypeScript: Extending Request and Response objects
You can also check out our Node.js category page for the latest tutorials and examples.