
In Node.js, you can compress a file using the Gzip format by using streams. We can get the job done with just a few lines of code and there is no need to install any third-party packages.
Example:
// Kindacode.com Example
const { createReadStream, createWriteStream } = require("fs");
const { createGzip } = require("zlib");
// Create a gzip function for reusable purpose
const compressFile = (filePath) => {
const stream = createReadStream(filePath);
stream
.pipe(createGzip())
.pipe(createWriteStream(`${filePath}.gz`))
.on("finish", () =>
console.log(`Successfully compressed the file at ${filePath}`)
);
};
// You can compress text files, images, SQL files, and many other file types.
compressFile("./kindacode.txt");
compressFile('./kindacode.jpeg');
Don’t forget to use your own file paths when running the preceding code snippet.
My screenshot:
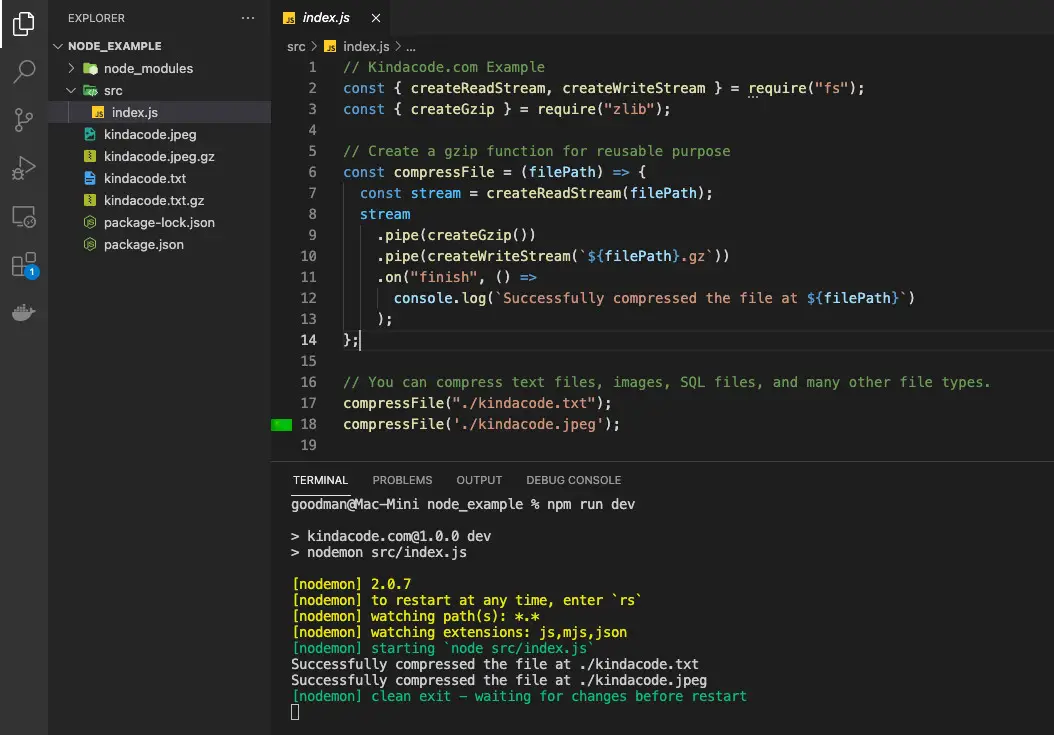
You can improve the code above by adding error handling so that you can use it for production with confidence.
Further reading:
- Using Axios to download images and videos in Node.js
- 4 Ways to Read JSON Files in Node.js
- Node.js: Using __dirname and __filename with ES Modules
- Node.js: Get File Name and Extension from Path/URL
- Node.js: Get domain, hostname, and protocol from a URL
You can also check out our Node.js category page for the latest tutorials and examples.