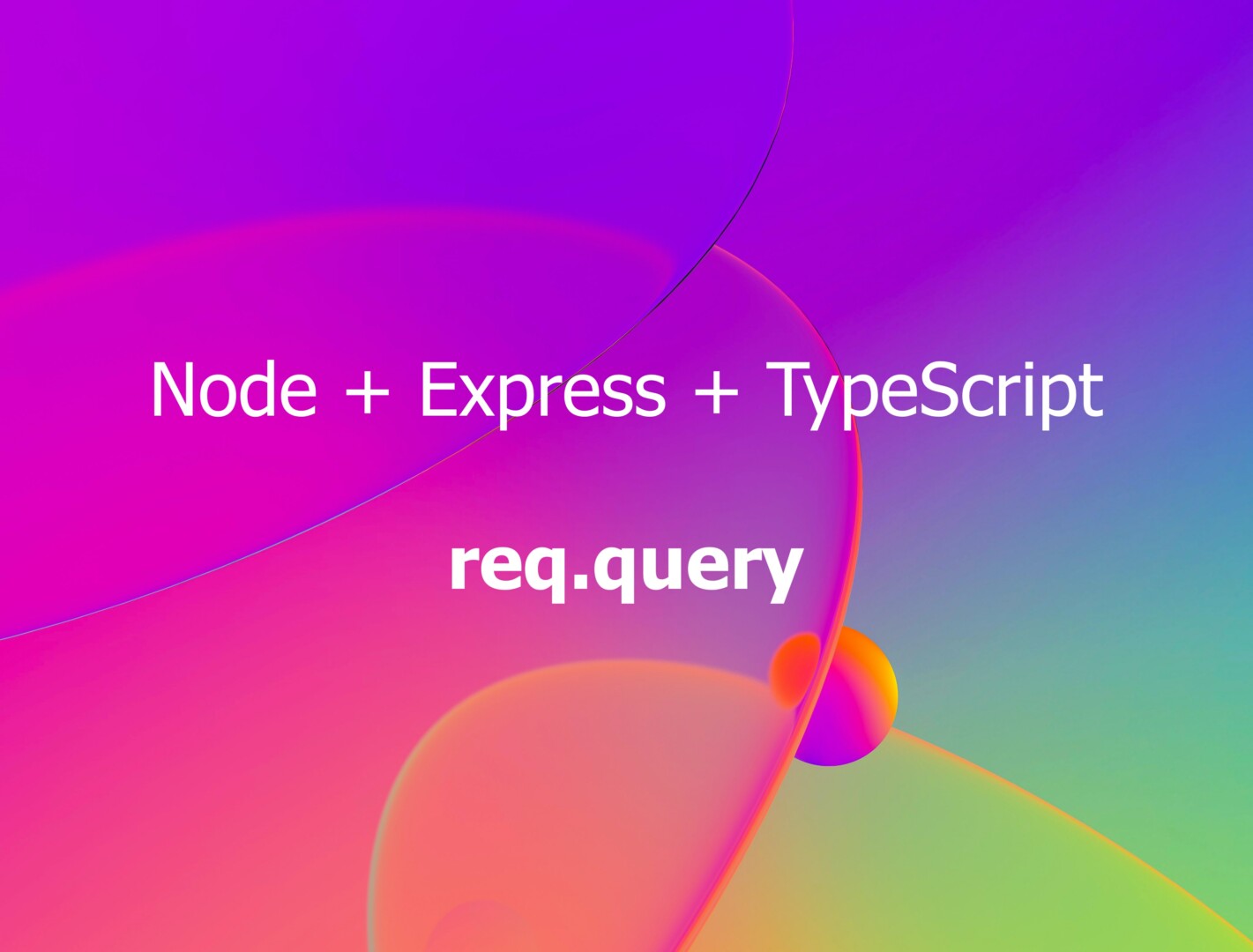
When working with Node and Express.js, there might be cases where you need to access req.query, an object that contains the property for each query string parameter in the route. For example, given a route like so:
http://localhost:3000?foo=abc&bar=def&something=123
Then req.query will look like this:
{ foo: 'abc', bar: 'def', something: '123' }
If you write code with TypeScript, you may wonder what is the proper type for req.query? There is more than one solution to this question.
Solution 1
The Request interface (which can be imported from express) is a generic which accepts additional definitions:
interface Request<
P = core.ParamsDictionary,
ResBody = any,
ReqBody = any,
ReqQuery = core.Query,
Locals extends Record<string, any> = Record<string, any>
> extends core.Request<P, ResBody, ReqBody, ReqQuery, Locals> {}
Therefore, we can do as follows:
import { Request, Response, NextFunction } from 'express';
// interfaces
interface ReqParams {
/* Add something as needed */
}
interface ReqBody {
/* Add something as needed */
}
interface Resbody {
/* Add something as needed */
}
interface ReqQuery {
foo: string;
bar: string;
something?: string;
}
// controller
export const getThings = (
req: Request<ReqParams, ReqBody, Resbody, ReqQuery>,
res: Response,
next: NextFunction
) => {
const { foo, bar, something } = req.query;
console.log(foo, bar, something);
res.status(200).json({ message: 'Welcome to KindaCode.com!' });
};
Solution 2
You can also use type casting like this:
import { Request, Response, NextFunction } from 'express';
// controller
export const getThings = (req: Request, res: Response, next: NextFunction) => {
const foo: string = req.query.foo as string;
const bar:string = req.query.bar as string;
const something: string = req.query.something as string;
console.log(foo, bar, something);
res.status(200).json({ message: 'Welcome to KindaCode.com!' });
};
That’s it. Further reading:
- How to Use Subqueries in TypeORM
- Node + Mongoose + TypeScript: Defining Schemas and Models
- TypeScript: Using Variables to Set Object Keys
- Nodemon: Automatically Restart a Node.js App on Crash
- Node.js: Using __dirname and __filename with ES Modules
- Best open-source ORM and ODM libraries for Node.js
You can also check out our Node.js category page for the latest tutorials and examples.