Node.js helps us build fast and high-performance backends for websites and mobile apps of all sizes. This job is made easier and more convenient thanks to the help of open-source frameworks.
In this article, we will walk through a list of the best Node.js frameworks for building APIs in 2024 (RESTful and GraphQL).
Express
- GitHub stars: 63.4k+
- NPM weekly downloads: 30m – 35m
- Written in: pure Javascript
- License: MIT
- Links: Official website | GitHub repo | NPM page
Express is one of the oldest, most stable Node.js frameworks. It first debuted in 2010, which was shortly after the arrival of Node.js.
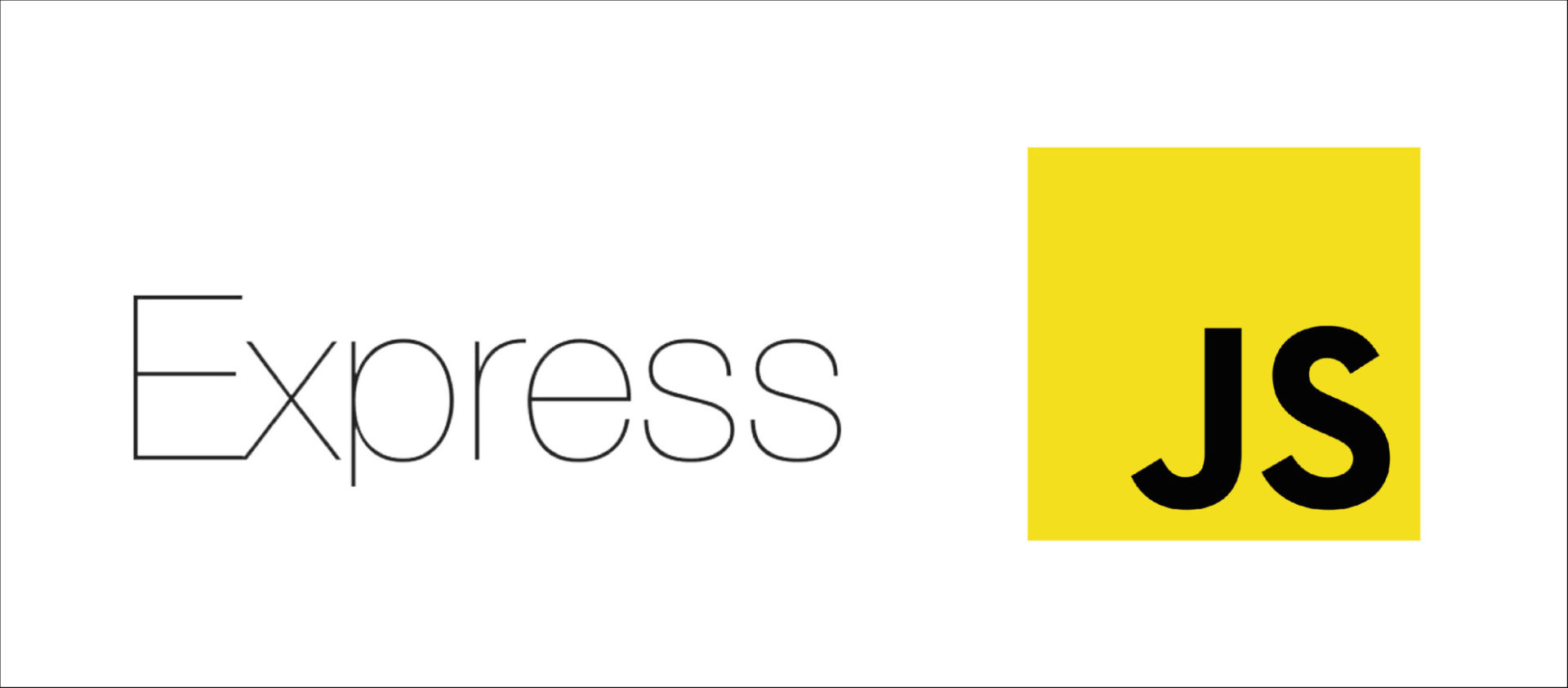
Express is lightweight, minimal, and flexible. It provides a thin layer of fundamental web application features, a myriad of HTTP utility methods, and middleware for quickly creating REST API. You can also easily implement GraphQL by using the express-graphql library.
A minimal Express API that runs on port 3000:
const express = require('express')
const app = express()
app.get('/', (req, res) => {
res.send('Hello World')
})
app.listen(3000)
Fastify
- GitHub stars: 30.3k+
- NPM weekly downloads: 1.8m – 2m
- Written in: Javascript and Typescript
- License: MIT
- Links: Official website | GitHub repo | NPM page
Fastify, as its name suggests, is a framework that focuses on performance and speed as well as saving resources for the server when it is up and running.

Like Express, Fastify is quite lightweight, highly flexible, and fully extensible via its hooks, plugins, and decorators. As far as we know, this is one of the fastest web frameworks these days, with the ability to serve up to 76+ thousands of requests per second.
Sample usage:
import Fastify from 'fastify'
const fastify = Fastify({
logger: true
})
fastify.get('/', async (request, reply) => {
reply.type('application/json').code(200)
return { hello: 'world' }
})
fastify.listen({ port: 3000 }, (err, address) => {
if (err) throw err
})
NestJS
- GitHub stars: 64k+
- NPM weekly downloads: 3.1m – 4m
- Written in: Typescript
- License: MIT
- Links: Official website | GitHub repo | NPM page
Nest is a progressive, modern Node.js framework that is growing rapidly, and more and more large businesses are choosing it for their projects.

Nest aims to provide an application architecture out of the box which allows for the effortless creation of highly testable, scalable, loosely coupled, and easily maintainable applications. The architecture is heavily inspired by Angular.
Defining REST API with Nest is simple. If you want to implement GraphQL, just use the built-in @nestjs/graphql module.
Koa
- GitHub stars: 35k+
- NPM weekly downloads: 1.6m – 2m
- Written in: pure Javascript
- License: MIT
- Links: Official website | GitHub repo | NPM page
Koa is a new web framework designed by the team behind Express, which aims to be a smaller, more expressive, and more robust foundation for web applications and APIs.
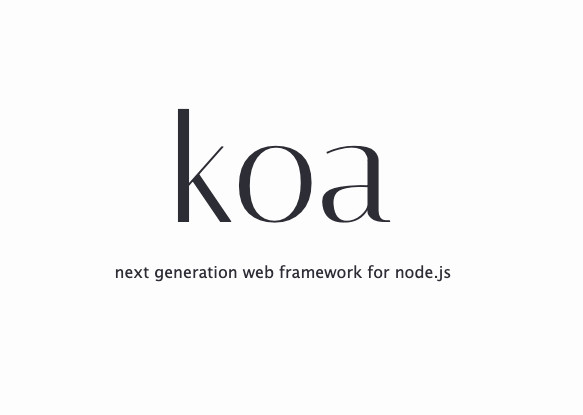
By leveraging async functions, Koa allows you to ditch callbacks and greatly increase error handling. Koa does not bundle any middleware within its core, and it provides an elegant suite of methods that make writing servers fast and enjoyable.
Connect
- GitHub stars: 9.8k+
- NPM weekly downloads: 6.8m – 8m
- Written in: Javascript
- License: MIT
- Links: GitHub repo | NPM page
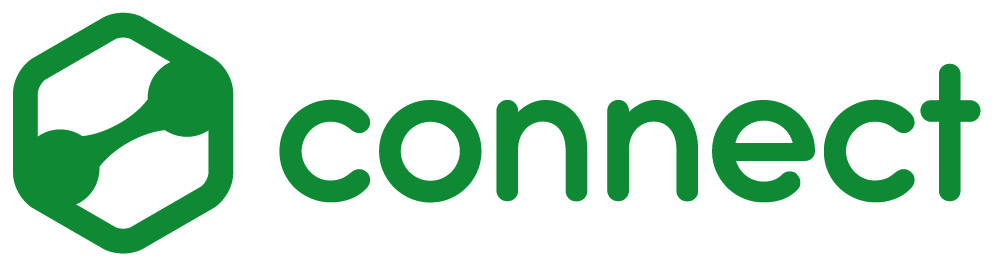
Connect is a lightweight HTTP server framework for Node.js whose unpacked size is only around 95 kB. It is simple and glues with various middleware to handle incoming requests. There are a very rich number of plugins to extend Connect’s behaviors, such as:
You can install connect and some plugins by running:
npm i connect compression cookie-session body-parser
And use them together like this:
const connect = require('connect');
const http = require('http');
const app = connect();
// gzip outgoing response
const compression = require('compression');
app.use(compression());
// session stuff
const cookieSession = require('cookie-session');
app.use(cookieSession({
keys: ['kitty', 'puppy']
}));
// using body-parser
var bodyParser = require('body-parser');
app.use(bodyParser.urlencoded({extended: false}));
// respond to all requests
app.use(function(req, res){
res.end('Hello from Connect!\n');
});
// Listen to incoming requests on port 3000
http.createServer(app).listen(3000);
LoopBack
- GitHub stars: 4.7k+ (the previous version gained 13.5k)
- NPM weekly downloads: 20k – 40k
- Written in: Javascript and Typescript
- License: MIT
- Links: Official website | GitHub repo | NPM page
LoopBack is a highly extensible Node.js and TypeScript framework
for building APIs and microservices.
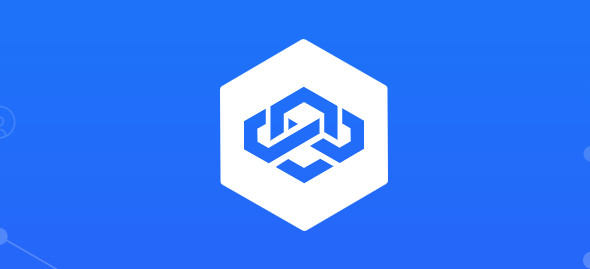
LoopBack 4 is the latest version of this awesome framework. It comes with a new, improved programming model with Dependency Injection and new concepts such as Components, Mixins, Repositories, etc., making this the most extensible version yet.
If you want to translate from REST to GraphQL with LoopBack, the official recommended approach in the docs is to install OpenAPI-to-GraphQL:
npm i -g openapi-to-graphql-cli
AdonisJS
- GitHub stars: 15.1k (Adonis core)
- NPM weekly downloads: 40k – 60k
- Written in: Typescript
- License: MIT
- Links: Official website | GitHub repo | NPM page
Adonis is a Node.js framework that focuses on developer ergonomics, stability, and confidence. It takes care of much of the web development hassles, offering you a clean and stable API to build Web apps and microservices.
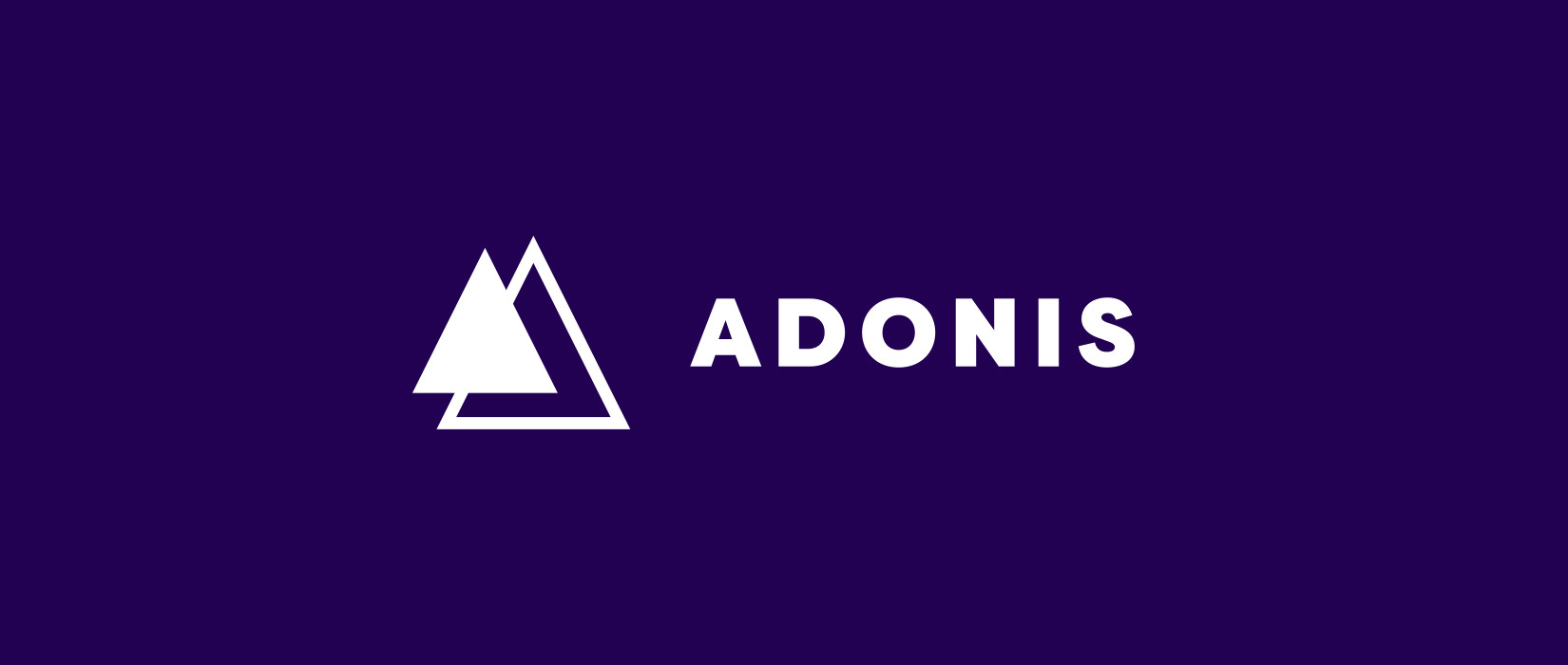
Conclusion
We have come across the best Node.js frameworks for building APIs in 2024. You don’t have to understand all of these, but knowing that they exist and knowing their characteristics will help you to have more approaches and strategies when building and developing the Node.js backend for new projects.
If you would like to explore more interesting things related to Node.js, take a look at the following articles:
- Top 5 best Node.js Open Source Headless CMS
- Node.js: Get domain, hostname, and protocol from a URL
- Node.js: Reading content from PDF and CSV files
- How to get all Links from a Webpage using Node.js and Cheerio
- Node.js + Express + TypeScript: req.query type
- Node.js: Listing Files in a Folder
You can also check out our Node.js category page for the latest tutorials and examples.
wat?
d