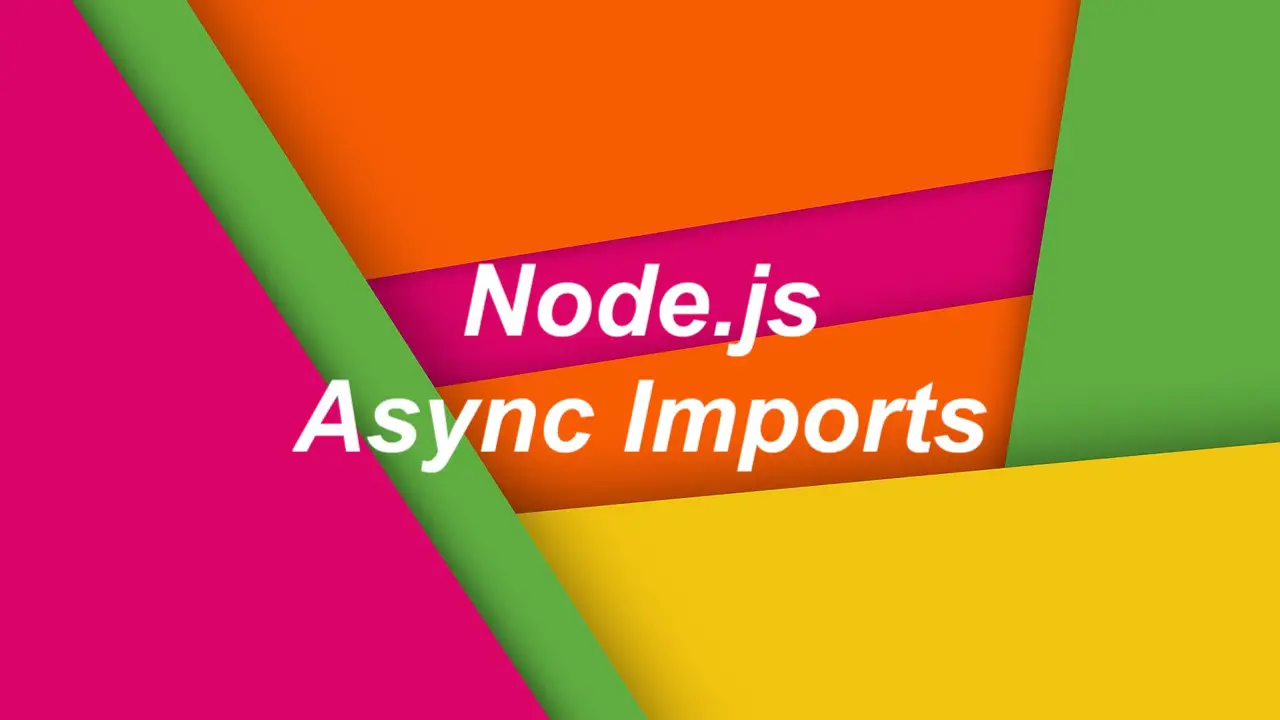
Overview
Nowadays, many modern Node.js applications use ES modules (that go with the import keyword) instead of CommonJS modules (that go with the require keyword). When working with ES modules, there might be scenarios where you want to import modules based on certain conditions. How do you construct a module identifier at runtime?
if(condition){
// import module A
} else {
// import module B
}
The solution here is to use import() as a function that takes a module identifier as an argument and it will return a promise that resolves to a module object. You may get a little confused about this. Don’t worry, the two examples below will make things much clearer.
A Quick Note
In order to use ES modules in Node.js at this time, you can follow one of the approaches below:
- Adding “type”: “module” to your package.json file.
- Saving you Javascript file with the .mjs extension instead of .js.
- Using TypeScript as your development language.
Example 1: Using Async/Await
1. Initialize a new npm project then create three empty files: index.js, module-a.js, and module-b.js:
.
├── index.js
├── module-a.js
├── module-b.js
└── package.json
2. Declaring “type”: “module” in the package.json file, like this:
{
"name": "node_kindacode",
"type": "module",
// Other stuff here
}
3. index.js:
// The condition will be passed when you run the "node index.js [condition]" command
const condition = process.argv[2];
const moduleName = `./module-${condition}.js`;
const run = async () => {
const importedModule = await import(moduleName);
importedModule.logger();
}
run();
4. module-a.js:
export const logger = () => {
console.log('This is module A');
}
5. module-b.js:
export const logger = () => {
console.log('This is module B');
}
6. Run:
node index.js a
Console output:
This is module A
When run:
node index.js b
You will get:
This is module b
Example 2: Using Promise & Then
Clone the entire project from the first example and replace the code in index.js with the following:
// The condition will be passed when you run the "node index.js [condition]" command
const condition = process.argv[2];
const moduleName = `./module-${condition}.js`;
import(moduleName).then(importedModule => importedModule.logger());
Now re-perform the 6th in the previous example to see what happens.
Conclusion
You’ve learned how to dynamically import an ES module. If you’d like to explore more new and interesting things in the modern Node.js world, take a look at the following articles:
- Node.js: How to Use “Import” and “Require” in the Same File
- How to Install Node.js on Ubuntu
- Node.js: Reading and Parsing Excel (XLSX) Files
- Node.js: How to Ping a Remote Server/ Website
- Best open-source ORM and ODM libraries for Node.js
- Node.js + Express + TypeScript: req.query type
You can also check out our Node.js category page for the latest tutorials and examples.