The example below shows you how to programmatically generate images from text in Node.js by using the canvas library.
Table of Contents
Example
Here’s the image we are going to “draw” with code:
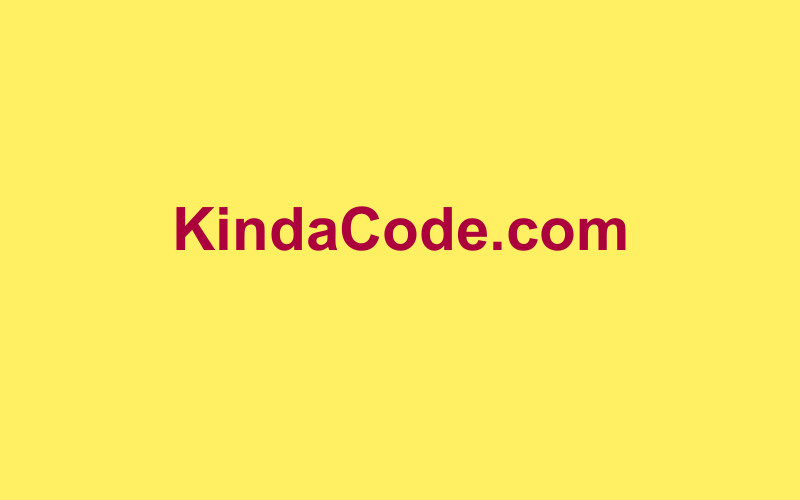
The code
1. Install the library:
npm i canvas
2. The complete code with explanations:
const fs = require("fs");
const { createCanvas } = require("canvas");
// width and height
const width = 800;
const height = 500;
const canvas = createCanvas(width, height);
const context = canvas.getContext("2d");
// Background color
context.fillStyle = "#ffef62";
context.fillRect(0, 0, width, height);
# Set text styles
context.font = "bold 60px Arial";
context.textAlign = "center";
// Set text color
context.fillStyle = "#ab003c";
// Write "KindaCode.com"
context.fillText("KindaCode.com", width / 2, height / 2);
const buffer = canvas.toBuffer("image/png");
fs.writeFileSync("output.png", buffer);
Final Words
We’ve walked through an elegant approach to creating images from text in Node.js. This is very helpful in many real-world use cases such as captcha stuff, online logo maker, etc. If you’d like to explore more new and exciting things about the awesome Javascript runtime, take a look at the following articles:
- Express + TypeScript: Extending Request and Response objects
- Node + Mongoose + TypeScript: Defining Schemas and Models
- Node.js: Reading content from PDF and CSV files
- Using Axios to download images and videos in Node.js
- Node.js: Reading and Parsing Excel (XLSX) Files
You can also check out our Node.js category page for the latest tutorials and examples.