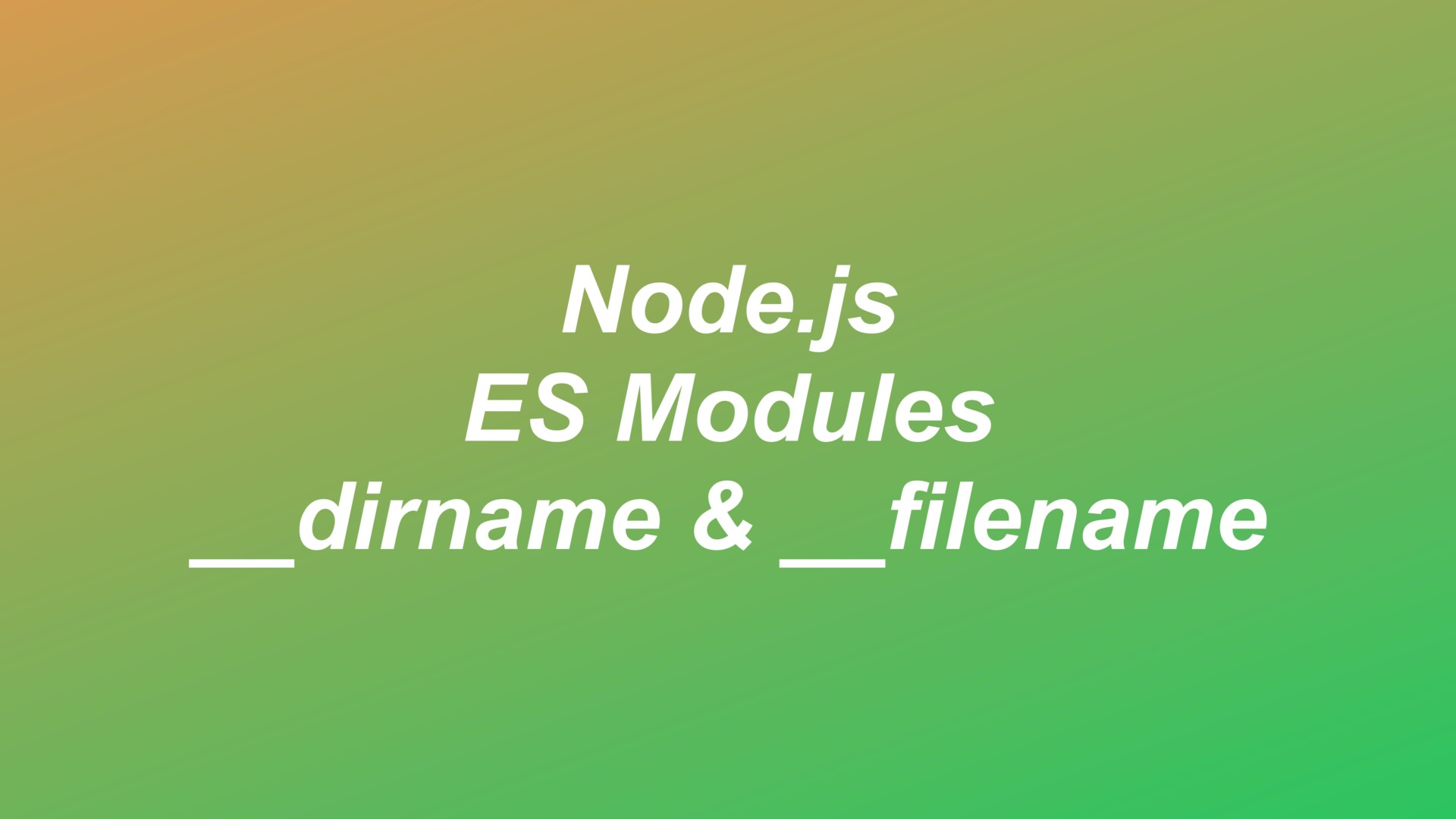
If you’re building a Node.js application with ES modules instead of CommonJS modules (“import” instead of “require”, in simpler terms), you have to do something before writing __filename and __direname in your code.
Table of Contents
The Problem
In Node.js:
- __filename: Returns the absolute path to the current file
- __dirname: Returns the absolute path to the parrent folder
Those things are not ready to be used if you’re working with ES modules and you will encounter the following errors:
ReferenceError: __dirname is not defined in ES module scope
And:
ReferenceError: __filename is not defined in ES module scope
Don’t panic. The solution is only a few lines of code.
The Solution
Here’s how we solve the problem:
import { fileURLToPath } from 'url'
import { dirname } from 'path'
const __filename = fileURLToPath(import.meta.url)
const __dirname = dirname(__filename)
console.log(__filename);
console.log(__dirname);
When executing the preceding code, you will see an output similar to this (your paths won’t be exactly the same as mine, of course):
/Users/goodman/Desktop/Dev/node/node_kindacode/src/index.js
/Users/goodman/Desktop/Dev/node/node_kindacode/src
Conclusion
You’ve learned how to use __dirname and __filename when working with ES6 modules in Node.js. If you’d like to explore more modern and exciting stuff about the Javascript runtime, take a look at the following articles:
- How to Install Node.js on Ubuntu 21.04 and 21.10
- Node.js: Generate Images from Text Using Canvas
- Node.js: Reading and Parsing Excel (XLSX) Files
- 7 Best Open-Source HTTP Request Libraries for Node.js
- Node.js: Get domain, hostname, and protocol from a URL
- Using Docker Compose with Node.js and MongoDB
You can also check out our Node.js category page for the latest tutorials and examples.