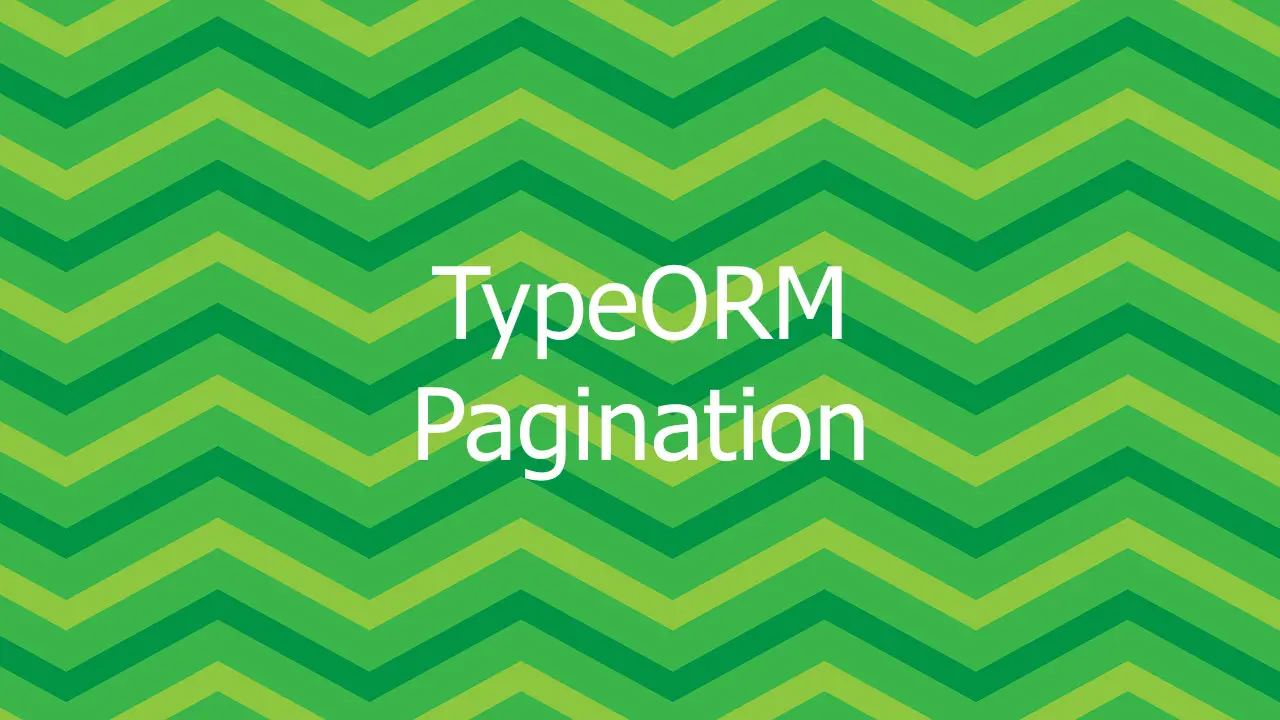
This short and straight-to-the-point article shows you how to paginate data in TypeORM.
Using Find(), FindAndCount() methods
If you use a find method, you can implement pagination with the skip and take options:
- skip: offset from where entities should be taken
- take: limit the number of entities that should be taken
Example:
const userRepository = dataSource.getRepository(User);
const results = await userRepository.findAndCount({
order: {
id: 'DESC'
}
skip: 0,
take: 10
})
Note that 0 and 10 here are sample numbers only. In real-world applications, they are transmitted from the frontend via HTTP requests.
Using QueryBuilder
If you prefer QueryBuilder to find methods, you can paginate with skip() and take() methods, like this:
const users = await dataSource
.getRepository(User)
.createQueryBuilder("user")
.orderBy("user.id", "DESC")
.take(10)
.skip(0)
.getMany()
Further reading:
- TypeORM: Selecting Rows with Null Values
- TypeORM: Entity with Decimal Data Type
- TypeORM: How to Limit Query Execution Time
- TypeORM: Adding Fields with Nullable/Default Data
- PostgreSQL: How to Rename a Column of a Table
- TypeORM: Adding created_at and updated_at columns
You can also check out our database topic page for the latest tutorials and examples.