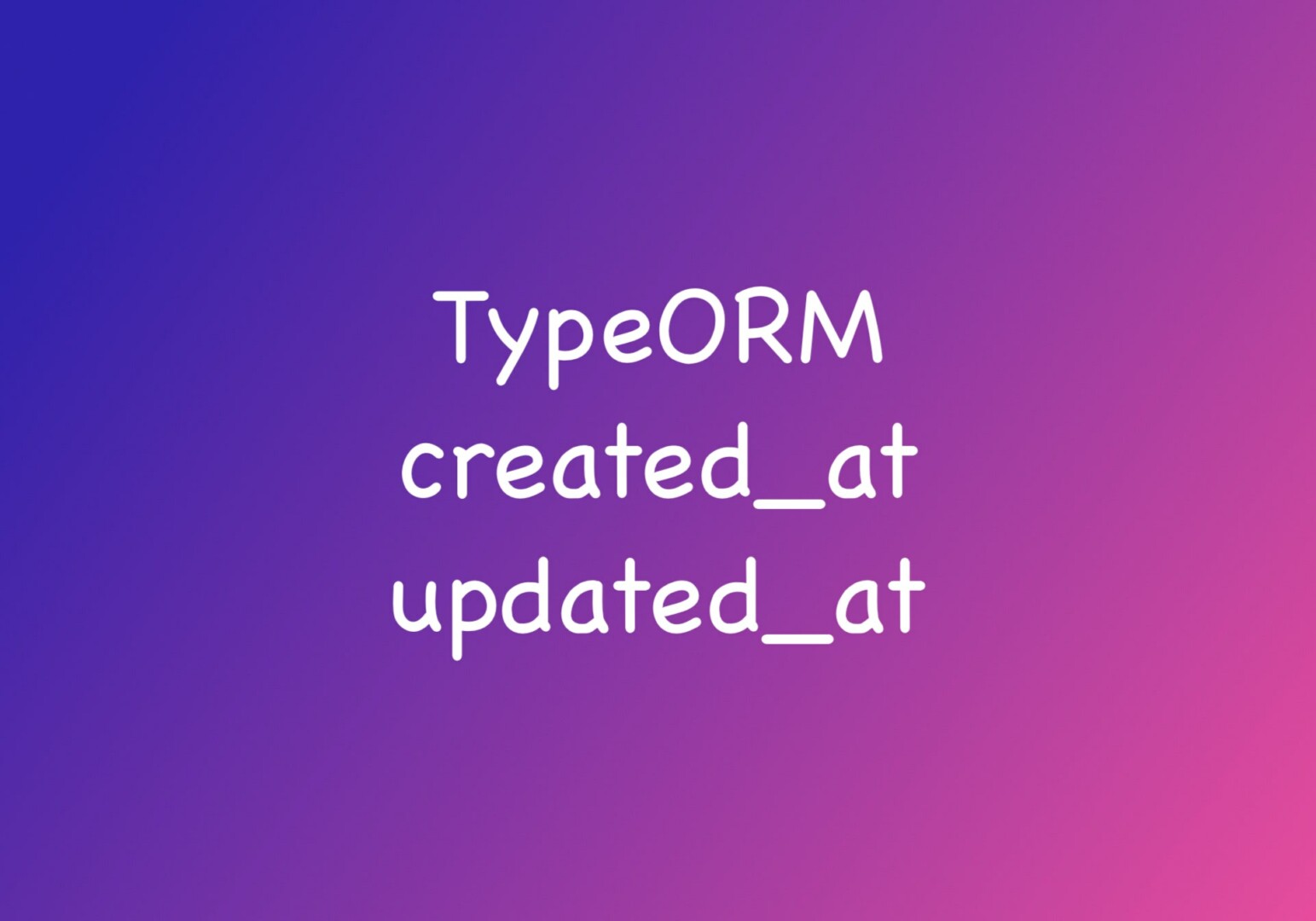
In TypeORM, you can add a created_at column and a updated_at column by making use of the CreateDateColumn and UpdateDateColumn decorators, respectively. These columns will be automatically initialized and updated to the current date and time (that is, the current timestamp).
Example:
import {
Entity,
PrimaryGeneratedColumn,
Column,
CreateDateColumn,
UpdateDateColumn,
} from 'typeorm'
@Entity()
export class Product {
@PrimaryGeneratedColumn()
id: number;
@Column()
name: string;
@CreateDateColumn()
created_at: Date;
@UpdateDateColumn()
updated_at: Date;
}
If you don’t want to use underscore (snake case) properties in your entity class but want your columns’ names are in underscore form, you can do like so:
import {
Entity,
PrimaryGeneratedColumn,
Column,
CreateDateColumn,
UpdateDateColumn,
} from 'typeorm'
@Entity()
export class Product {
@PrimaryGeneratedColumn()
id: number;
@Column()
name: string;
@CreateDateColumn({name: 'created_at'})
createdAt: Date;
@UpdateDateColumn({name: 'updated_at'})
updatedAt: Date;
}
Now the createdAt and updatedAt properties are all camelCase and the code looks a little bit nicer.
Reference: TypeORM special columns (typeorm.io).
Further reading:
- Unsigned (non-negative) Integer in PostgreSQL
- Best open-source ORM and ODM libraries for Node.js
- 2 Ways to Get the Size of a Database in PostgreSQL
- Top 4 best Node.js Open Source Headless CMS
- Node + Mongoose + TypeScript: Defining Schemas and Models
You can also check out our Node.js category page for the latest tutorials and examples.