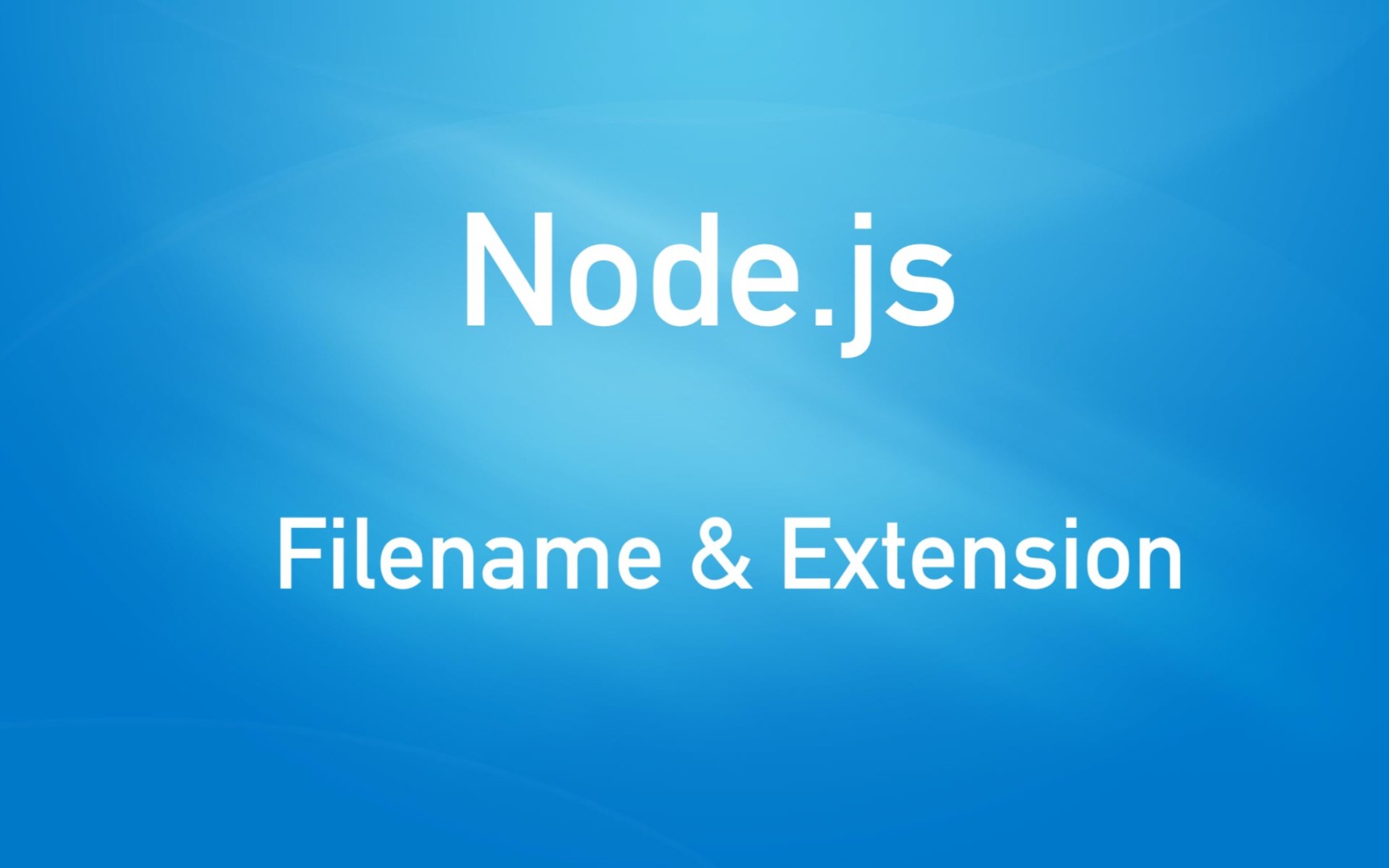
The path module (one of the core modules of Node.js) gives us some methods to simply retrieve the name and extension of a file from a path or web URL:
- path.basename(path: string, extension?: string): Returns the file name with the extension if extension is not passed. On the other hand, If extension is given, the method returns the file name without the extension.
- path.extname(path): Return the file extension.
- path.wind32.basename(path: string, extension?: string): Used to deal with Windows-style paths which contain double-backslashes
Table of Contents
Example
The code:
const path = require('path');
// File path
SAMPLE_PATH = '/Users/goodman/Desktop/dev/node_kindacode/package.json';
const name1 = path.basename(SAMPLE_PATH);
const ext1 = path.extname(SAMPLE_PATH);
const nameWithoutExt1 = path.basename(name1, ext1);
console.log(
'Filename:',
name1,
'Extension:',
ext1,
'Name without extenions:',
nameWithoutExt1
);
// Web URL
SAMPLE_URL =
'https://www.kindacode.com/wp-content/uploads/2021/04/nodejs-file.jpg';
const name2 = path.basename(SAMPLE_URL);
const ext2 = path.extname(SAMPLE_URL);
const nameWithoutExt2 = path.basename(name2, ext2);
console.log(
'Filename:',
name2,
'Extension:',
ext2,
'Name without extenions:',
nameWithoutExt2
);
Output:
Filename: package.json Extension: .json Name without extenions: package
Filename: nodejs-file.jpg Extension: .jpg Name without extenions: nodejs-file
path.win32.basename() use cases
Note: If your Node.js program only runs on Windows then you don’t need to care about path.win32.basename(). This is for macOS/Linux users or people who want to achieve consistent results when working with file paths on any operating system including Windows.
Using the path.basename() method might return an unwanted result if your Node.js program is running on macOS (or Ubuntu) and the input path is Windows-style with double-backslashes like this:
"C:\\Personal\\MyFolder\\MyFile.jpg";
In this case, we need to use path.win32.basename() instead to ensure you get the correct result everywhere:
Example:
const path = require('path');
const FILE_PATH = "C:\\Personal\\MyFolder\\MyFile.jpg";
// Do NOT use this
console.log('Unwanted result:', path.basename(FILE_PATH));
// Use this
console.log('Correct result:', path.win32.basename(FILE_PATH));
The output on my machine (macOS):
Unwanted result: C:\Personal\MyFolder\MyFile.jpg
Correct result: MyFile.jpg
Final Words
You’ve learned how to retrieve the name and extension of a file from a given path or URL. Continue learning more interesting stuff about Node.js by taking a look at the following articles:
- Best Node.js Open Source Headless CMS
- Node + Mongoose + TypeScript: Defining Schemas and Models
- Best Node.js frameworks to build backend APIs
- Using Axios to download images and videos in Node.js
- How to get all Links from a Webpage using Node.js and Cheerio
You can also check out our Node.js category page for the latest tutorials and examples.