
If you want to store some kind of flexible data with TypeORM, you can save them as JSON. You can specify the column type as simple-json. This helps you store and load Javascript objects with ease.
Let’s examine a concrete example for more clarity.
1. Define an entity called Product:
// Product entity
import {
Entity,
PrimaryGeneratedColumn,
Column,
CreateDateColumn,
} from 'typeorm';
@Entity({name: 'products'})
export class Product {
@PrimaryGeneratedColumn()
id: number;
@Column()
name: string;
// Column with JSON data type
@Column('simple-json', {nullable: true})
otherInfo: {
description: string;
freeShipping: boolean;
weight: number;
color: string;
};
@Column({default: 0})
price: number
}
2. Add a new product to the database:
const productRepository = dataSource.getRepository(Product);
const product = new Product();
product.name = 'KindaCode.com';
// JSON stuff here
product.otherInfo = {
description: 'This is a description',
freeShipping: true,
weight: 1,
color: 'red',
};
product.price = 100;
await productRepository.save(product);
When viewing your table products in your database, you’ll see this (put your attention on the otherInfo column):
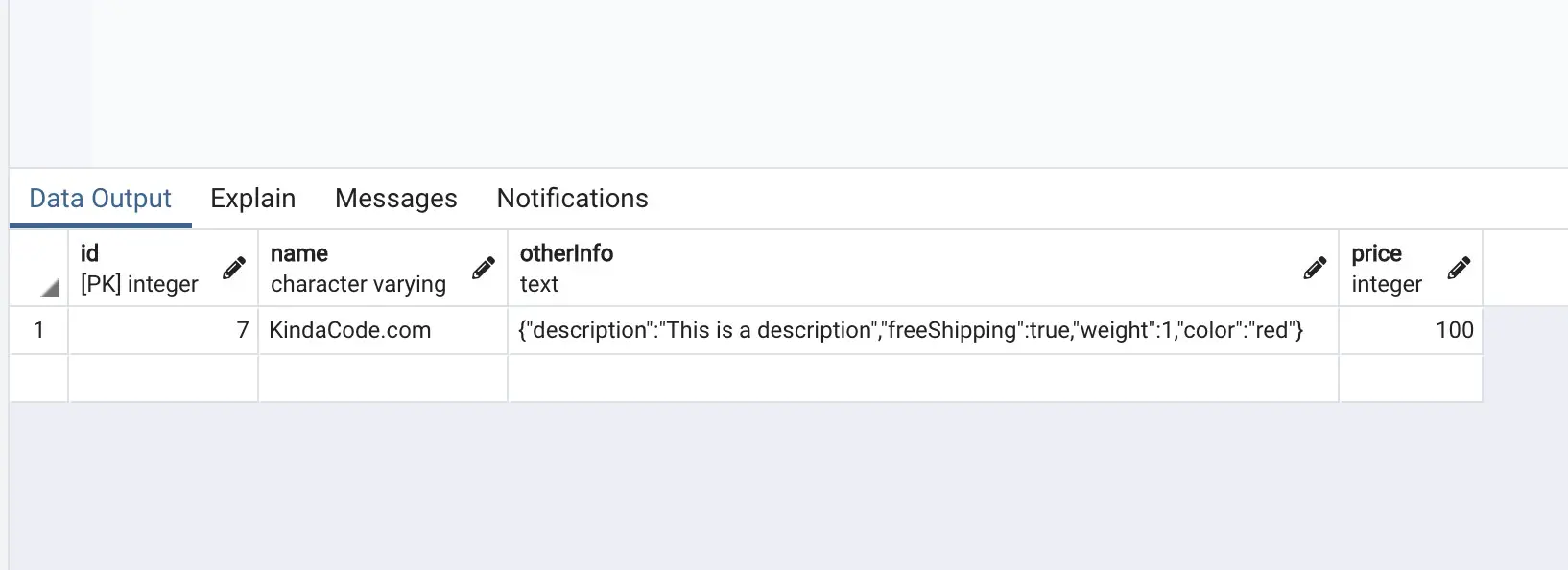
That’s it. Further reading:
- TypeORM: Add Columns with Array Data Type
- Pagination in TypeORM (Find Options & QueryBuilder)
- TypeORM: Entity with Decimal Data Type
- 2 Ways to View the Structure of a Table in PostgreSQL
- Using ENUM Type in TypeORM
You can also check out our database topic page for the latest tutorials and examples.
Thank you