This practical article walks you through a few examples of how to use ImageBackground in React Native.
Example 1: Full-screen image background
import React from "react";
import { View, Text, ImageBackground, StyleSheet } from "react-native";
const bgImage = {
uri: "https://www.kindacode.com/wp-content/uploads/2022/03/blue-sky.jpeg",
};
const App = () => {
return (
<ImageBackground source={bgImage} style={styles.background}>
<View style={styles.container}>
<Text style={styles.text}>Good morning!</Text>
</View>
</ImageBackground>
);
};
// styling
const styles = StyleSheet.create({
background: {
flex: 1,
},
container: {
flex: 1,
justifyContent: "center",
alignItems: "center",
},
text: {
fontSize: 30,
},
});
export default App;
Result:
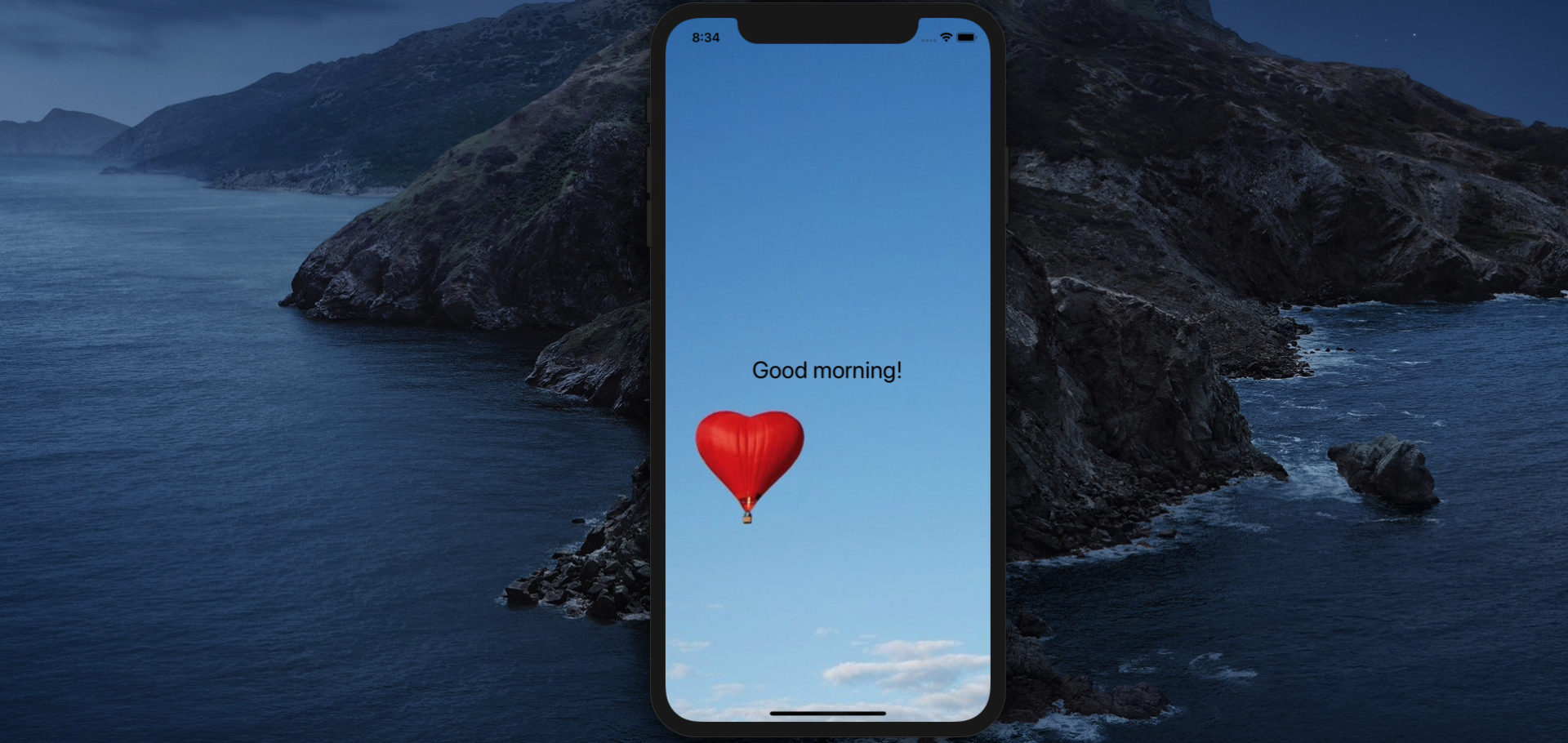
Example 2: Using resizeMode
The resizeMode property determines how to resize the image when the frame doesn’t match the raw image dimensions.
import React from 'react';
import {View, Text, ImageBackground, StyleSheet} from 'react-native';
const bgImage = {
uri:
'https://www.kindacode.com/wp-content/uploads/2022/03/green.jpeg',
};
const App = () => {
return (
<ImageBackground
source={bgImage}
style={styles.background}
resizeMode="contain">
<View style={styles.container}>
<Text style={styles.text}>Good morning!</Text>
</View>
</ImageBackground>
);
};
// styling
const styles = StyleSheet.create({
background: {
margin: 60,
width: 300,
height: 300,
},
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
text: {
fontSize: 30,
fontWeight: 'bold',
color: '#fff',
},
});
export default App;
What you should see when running your code:

Example 3: Rounded Corners Area With imageBackground
We can create a rounded-corner background image by using borderRadius like so:
import React from 'react';
import {View, Text, ImageBackground, StyleSheet} from 'react-native';
const bgImage = {
uri:
'https://www.kindacode.com/wp-content/uploads/2022/03/green.jpeg',
};
const App = () => {
return (
<ImageBackground
source={bgImage}
style={styles.background}
// add this line
imageStyle={{borderRadius: 200}}
resizeMode="contain">
<View style={styles.container}>
<Text style={styles.text}>Good morning!</Text>
</View>
</ImageBackground>
);
};
// styling
const styles = StyleSheet.create({
background: {
margin: 60,
width: 300,
height: 300,
},
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
text: {
fontSize: 30,
fontWeight: 'bold',
color: '#fff',
},
});
export default App;
Result:

The above examples are quite simple but from here, you’re pretty good to go. We used some images from Pixabay, under the Pixabay License.
Final Words
We’ve examined a couple of examples about using the BackgroundImage component to create a more attractive user interface. If you’d like to learn more new and exciting stuff about modern React Native, take a look at the following articles:
- How to Get the Window Width & Height in React Native
- How to set a gradient background in React Native
- How to create circle images in React Native
- Top 4 open-source React Native UI libraries
- Implementing a Date Time picker in React Native
You can also check our React topic page and React Native topic page for the latest tutorials and examples.