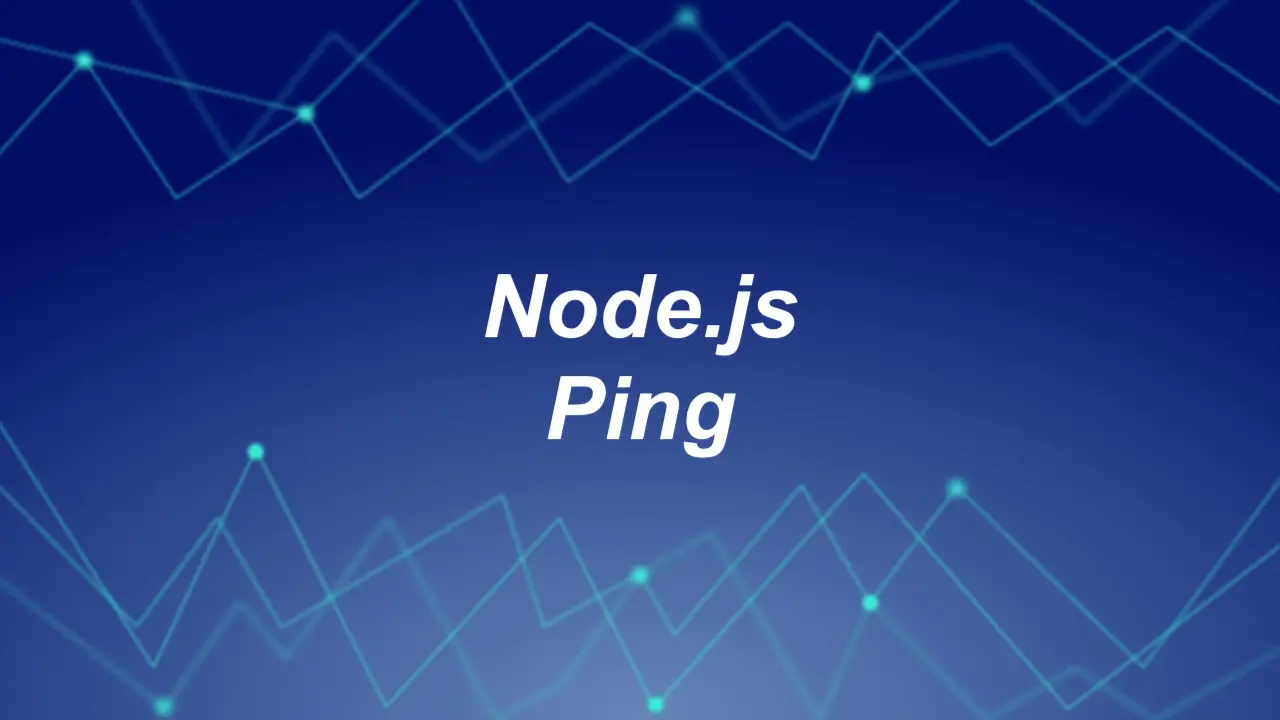
This article shows you a couple of different ways to programmatically ping a remote server or a website from within your Node.js application (to get some network information like latency, IP address, …). In the first approach, we will solve the task ourselves by writing code from scratch. In the second one, we will get the job done quickly by using a third-party package.
Table of Contents
Using self-written code
The strategy here is to perform the ping command provided by the operating system (Linux, macOS, Windows) in our Node.js app. Fortunately, we can do that easily with the help of the child_process module of Node.js.
Example:
// kindacode.com
// this example uses ES modules (import) instead of CommonJS (require)
import util from 'util'
import { createRequire } from "module";
const require = createRequire(import.meta.url);
const exec = util.promisify(require('child_process').exec);
const ping = async (host) => {
const {stdout, stderr} = await exec(`ping -c 5 ${host}`);
console.log(stdout);
console.log(stderr);
}
ping('www.kindacode.com');
Output:
PING www.kindacode.com (104.21.33.69): 56 data bytes
64 bytes from 104.21.33.69: icmp_seq=0 ttl=59 time=38.733 ms
64 bytes from 104.21.33.69: icmp_seq=1 ttl=59 time=37.775 ms
64 bytes from 104.21.33.69: icmp_seq=2 ttl=59 time=137.648 ms
64 bytes from 104.21.33.69: icmp_seq=3 ttl=59 time=42.290 ms
64 bytes from 104.21.33.69: icmp_seq=4 ttl=59 time=34.817 ms
--- www.kindacode.com ping statistics ---
5 packets transmitted, 5 packets received, 0.0% packet loss
round-trip min/avg/max/stddev = 34.817/58.253/137.648/39.769 ms
The output you get might be different from mine (depending on your location and network).
Using a 3rd library
Even though ping stuff is not a big challenge in Node.js, many developers prefer to use a pre-made library. A great one for this case is ping (you can freely use it under the MIT License).
Installing:
npm i ping
Example:
// kindacode.com
// this example uses ES Modules (import) instead of CommonJS (require)
import ping from 'ping'
(async function () {
const result = await ping.promise.probe('www.kindacode.com', {
timeout: 10,
extra: ["-i", "2"],
});
console.log(result);
})();
Output:
{
inputHost: 'www.kindacode.com',
host: 'www.kindacode.com',
alive: true,
output: 'PING www.kindacode.com (104.21.33.69): 56 data bytes\n' +
'64 bytes from 104.21.33.69: icmp_seq=0 ttl=59 time=33.207 ms\n' +
'\n' +
'--- www.kindacode.com ping statistics ---\n' +
'1 packets transmitted, 1 packets received, 0.0% packet loss\n' +
'round-trip min/avg/max/stddev = 33.207/33.207/33.207/0.000 ms\n',
time: 33.207,
times: [ 33.207 ],
min: '33.207',
max: '33.207',
avg: '33.207',
stddev: '0.000',
packetLoss: '0.000',
numeric_host: '104.21.33.69'
}
Conclusion
We’ve walked through more than one technique to ping a remote host with some lines of code. If you’d like to explore more new and exciting things in the modern Node.js world, take a look at the following articles:
- Best open-source ORM and ODM libraries for Node.js
- 7 Best Open-Source HTTP Request Libraries for Node.js
- Express + TypeScript: Extending Request and Response objects
- Node + Mongoose + TypeScript: Defining Schemas and Models
- Node.js: How to Use “Import” and “Require” in the Same File
- How to Resize Images using Node.js
You can also check out our Node.js category page or PHP category page for the latest tutorials and examples.