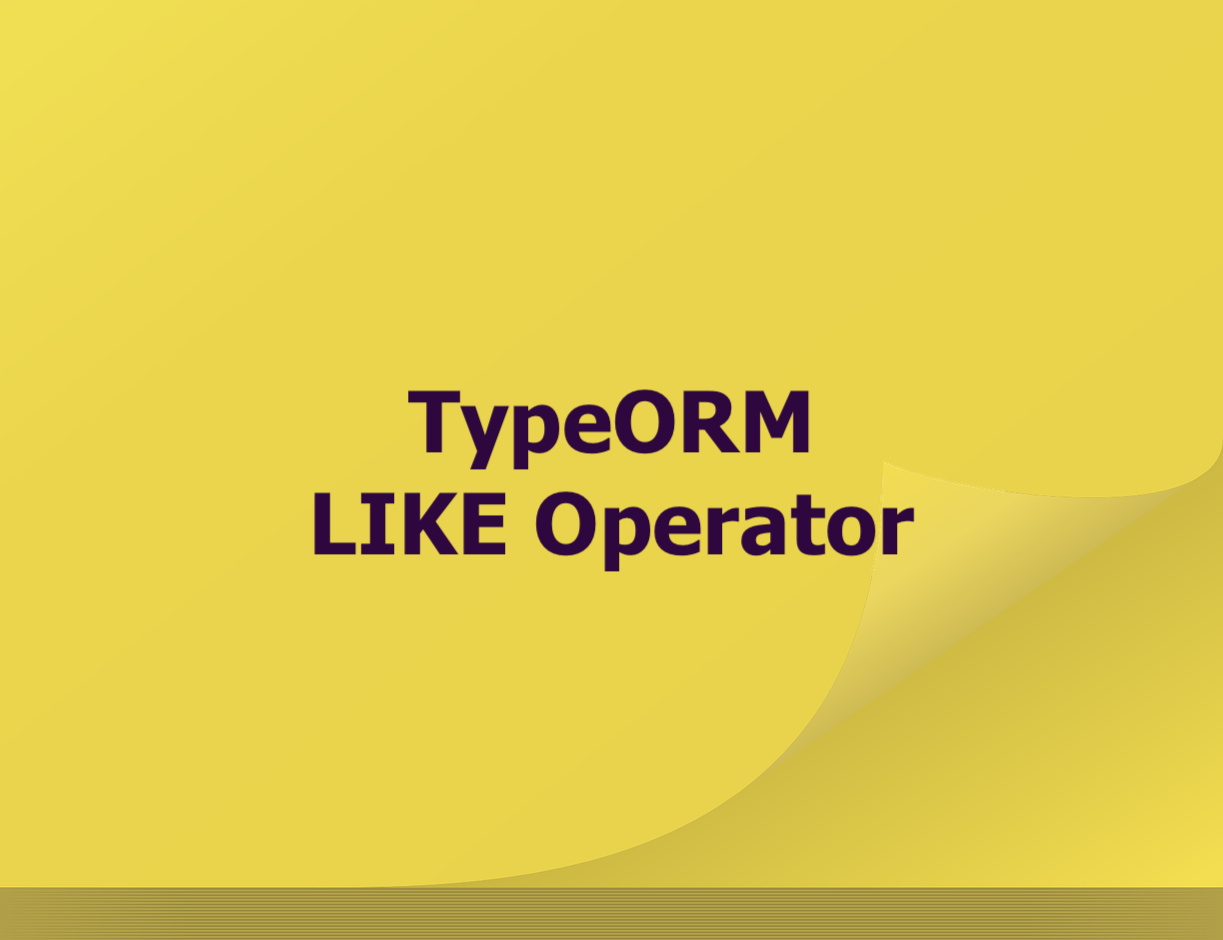
In TypeORM, the Like operator (with the percent sign %) is used to search for a specified pattern in a column. This concise article walks you through 2 examples of selecting data with the Like operator in 2 different ways:
- Using the find() method
- Using a query builder
A quick note about the Like keyword and the percent sign:
- Like ‘kindacode.com%’: Finds any values that start with “kindacode.com”
- Like ‘%kindacode.com’: Finds any values that end with “kindacode.com”
- Like ‘%kindacode.com%’: Finds any values that contain “kindacode.com” (in any position)
We’ll work with a sample entity named Post (that stores blog posts in three columns: id, title, and body):
import { Entity, Column, PrimaryGeneratedColumn, Unique } from 'typeorm';
@Entity()
@Unique(['title'])
export class Post {
@PrimaryGeneratedColumn()
id: number;
@Column()
title: string;
@Column()
body: string;
}
Using the find() method
This example selects all blog posts whose titles contain the word: ‘kindacode.com’.
import { Like } from 'typeorm';
/*...*/
const postRepository = myDataSource.getRepository(Post);
const posts = await postRepository.find({
where: { title: Like('%kindacode.com%') },
});
console.log(posts);
Using a query builder
This code snippet does the same thing as the preceding one, but in a distinguishable manner (you don’t have to import { Like } from ‘typeorm’):
const postRepository = myDataSource.getRepository(Post);
const posts = await postRepository
.createQueryBuilder('post')
.where('post.title LIKE :title', { title: '%kindacode.com%' })
.getMany();
console.log(posts);
That’s it. Further reading:
- TypeORM Upsert: Update If Exists, Create If Not Exists
- TypeORM: Find Rows Where Column Value is IN an Array
- How to Store JSON Object with TypeORM
- TypeORM: How to Limit Query Execution Time
- TypeORM: 2 Ways to Exclude a Column from being Selected
You can also check out our Javascript category page, TypeScript category page, Node.js category page, and React category page for the latest tutorials and examples.