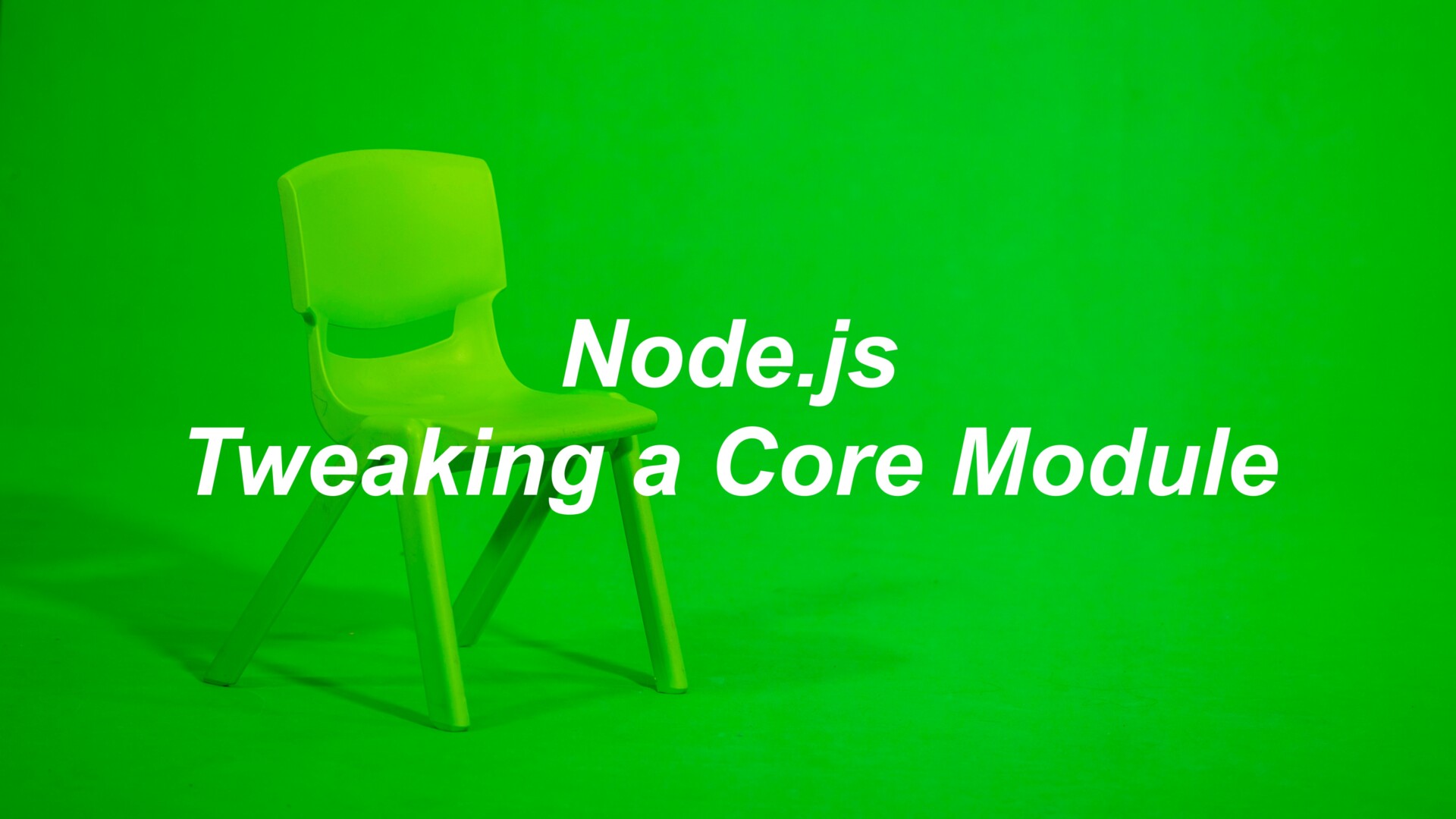
The example below shows you how to change the behavior of a Node.js core module by modifying or extending it. What we are going to do should not appear in serious products but might be somehow helpful for learning and testing purposes.
In this example, we will make some changes to fs/promises.
const fsPromises = require("fs/promises");
// Mutating an existing functionality
fsPromises.readFile = (fakePath) => {
return "This is the fake data";
};
// Extending the module
fsPromises.sayHello = () => {
console.log('Hello there!')
}
const run = async () => {
fsPromises.sayHello();
const data = await fsPromises.readFile("a-mock-file");
console.log(data);
};
run();
Run the code and you should get this output in your console:
Hello there!
This is the fake data
Further reading:
- How to Install Node.js on Ubuntu 21.04 and 21.10
- Best open-source ORM and ODM libraries for Node.js
- 6 best Node.js frameworks to build backend APIs
- How to Generate Slugs from Titles in Node.js
- How to Delete a File or Directory in Node.js
- Node.js: Get domain, hostname, and protocol from a URL
You can also check out our Node.js category page for the latest tutorials and examples.