The example below shows you how to change the text of a button after it gets clicked in React. We’ll use the useState hook to get the job done.
Table of Contents
App Preview
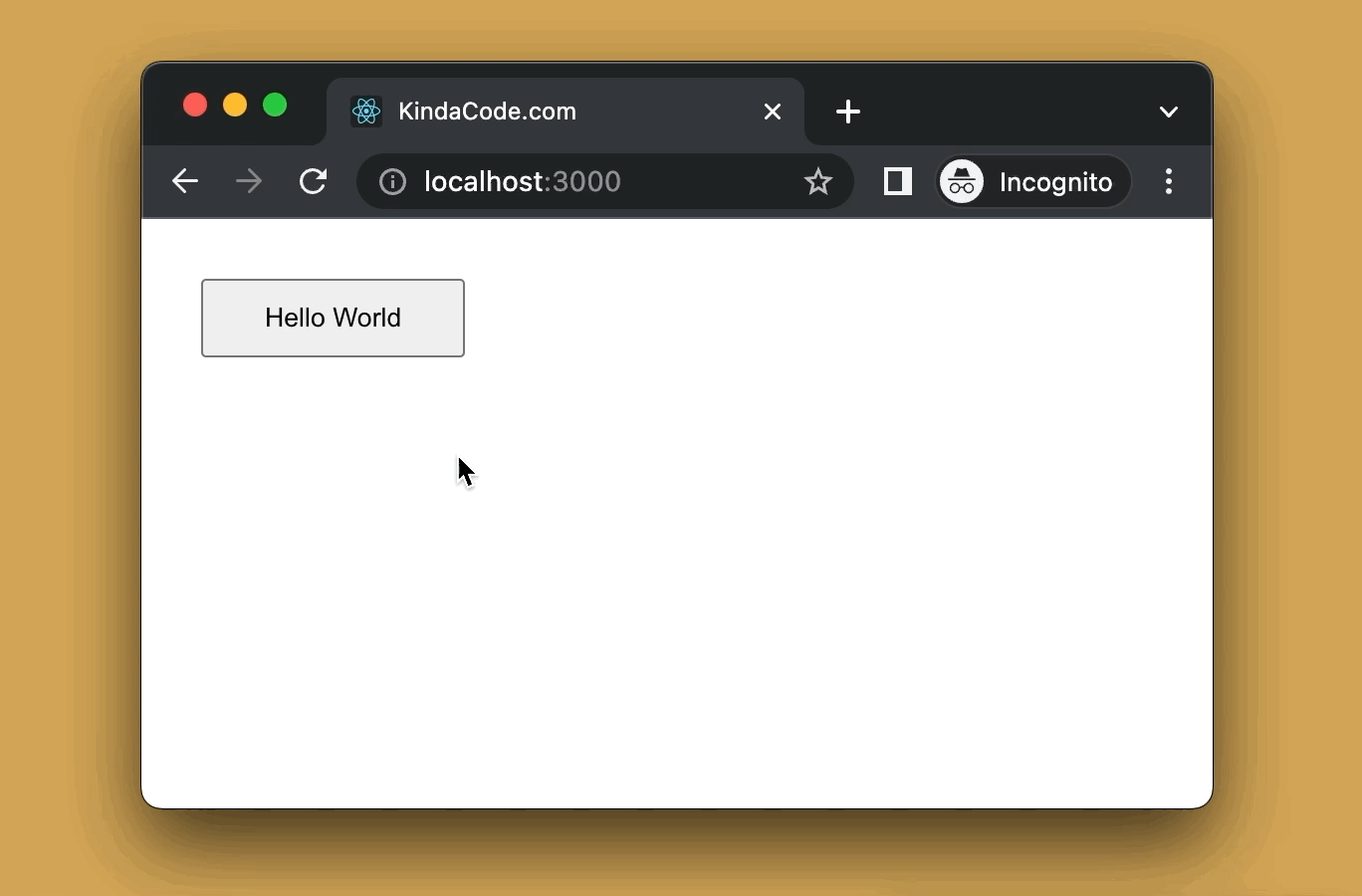
The Code
// KindaCode.com
// src/App.js
import { useState } from 'react';
function App() {
// Button Text
const [buttonText, setButtonText] = useState('Hello World');
// This function will be called when the button is clicked
const handleClick = () => {
setButtonText('Goodbye, World!');
};
return (
<div style={{ padding: 30 }}>
<button onClick={handleClick} style={{ padding: '10px 30px' }}>
{/* Button Text */}
{buttonText}
</button>
</div>
);
}
export default App;
Explanation
1. Store the button text in a state variable so that we can update it programmatically:
const [buttonText, setButtonText] = useState('Hello World');
2. Set a handler function for the onClick event:
<button onClick={handleClick}>{buttonText}</button>
3. Call the state updater function to set new button text:
const handleClick = () => {
setButtonText('Goodbye, World!');
};
That’s it. Further reading:
- React: How to Disable a Button after One Click
- React + TypeScript: Using setTimeout() with Hooks
- React: How to Check Internet Connection (Online/Offline)
- React: Create a Fullscreen Search Overlay from Scratch
- React: How to Create a Responsive Navbar from Scratch
- React + TypeScript: Multiple Dynamic Checkboxes
You can also check our React category page and React Native category page for the latest tutorials and examples.