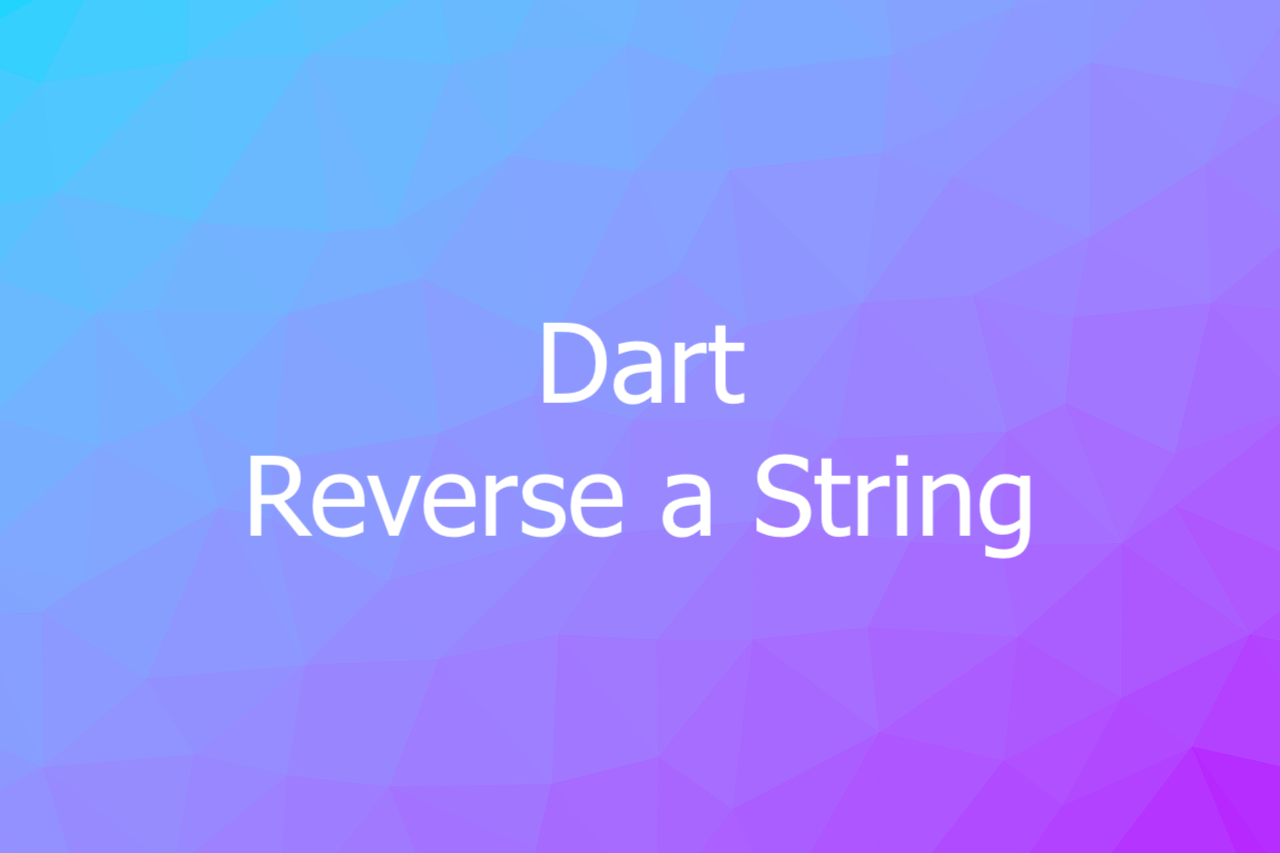
The three examples below show you three different ways to reverse a given string in Dart (e.g. turn ABC to CBA).
Turn the String into an Array
Example:
// KindaCode.com
// main.dart
// Define a reusable function
String reverseString(String input) {
final output = input.split('').reversed.join('');
return output;
}
void main() {
// Try it
print(reverseString('ABCDEFGH'));
print(reverseString('The busy dog jumps over the lazy dog'));
}
Output:
HGFEDCBA
god yzal eht revo spmuj god ysub ehT
Using StringBuffer
Example:
// Define a reusable function
String reverseString(String input) {
var buffer = StringBuffer();
for (var i = input.length - 1; i >= 0; --i) {
buffer.write(input[i]);
}
return buffer.toString();
}
void main() {
// Try it
print(reverseString('123456789'));
print(reverseString('Welcome to KindaCode.com'));
}
Output:
987654321
moc.edoCadniK ot emocleW
Using Char Code Technique
Example:
// Define a reusable function
String reverseString(String input) {
String reversedString = String.fromCharCodes(input.codeUnits.reversed);
return reversedString;
}
void main() {
// Test it
print(reverseString('banana'));
print(reverseString('Welcome to KindaCode.com'));
}
Output:
ananab
moc.edoCadniK ot emocleW
Futher reading:
- How to Subtract two Dates in Flutter & Dart
- Dart & Flutter: Convert a Duration to HH:mm:ss format
- Flutter & Dart: How to Check if a String is Null/Empty
- Flutter: Floating Action Button examples (basic & advanced)
- Flutter: Programmatically Check Soft Keyboard Visibility
- Dart: Checking if a Map contains a given Key/Value
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.