In Flutter, you can render an image from an Int8List of bytes by using the Image.memory constructor like so:
Image.memory(Uint8List.fromList(myBytes));
If what you have are byte buffers, use this:
Image.memory(Uint8List.fromList(myBuffer.asInt8List()));
Example
This example does the following things:
- Reading byte data from a given URL of an image (you can change this step to suit your situation, such as fetch byte data from a file or from a database)
- Using Image.memory to render the image from byte data
The purpose of this example is to demonstrate what I mentioned earlier. In reality, the tiny app we’re going to build doesn’t make lots of sense because we can directly show an image with its URL instead of the detour of getting its bytes from the URL and then using this byte list to display the image.
Screenshot:
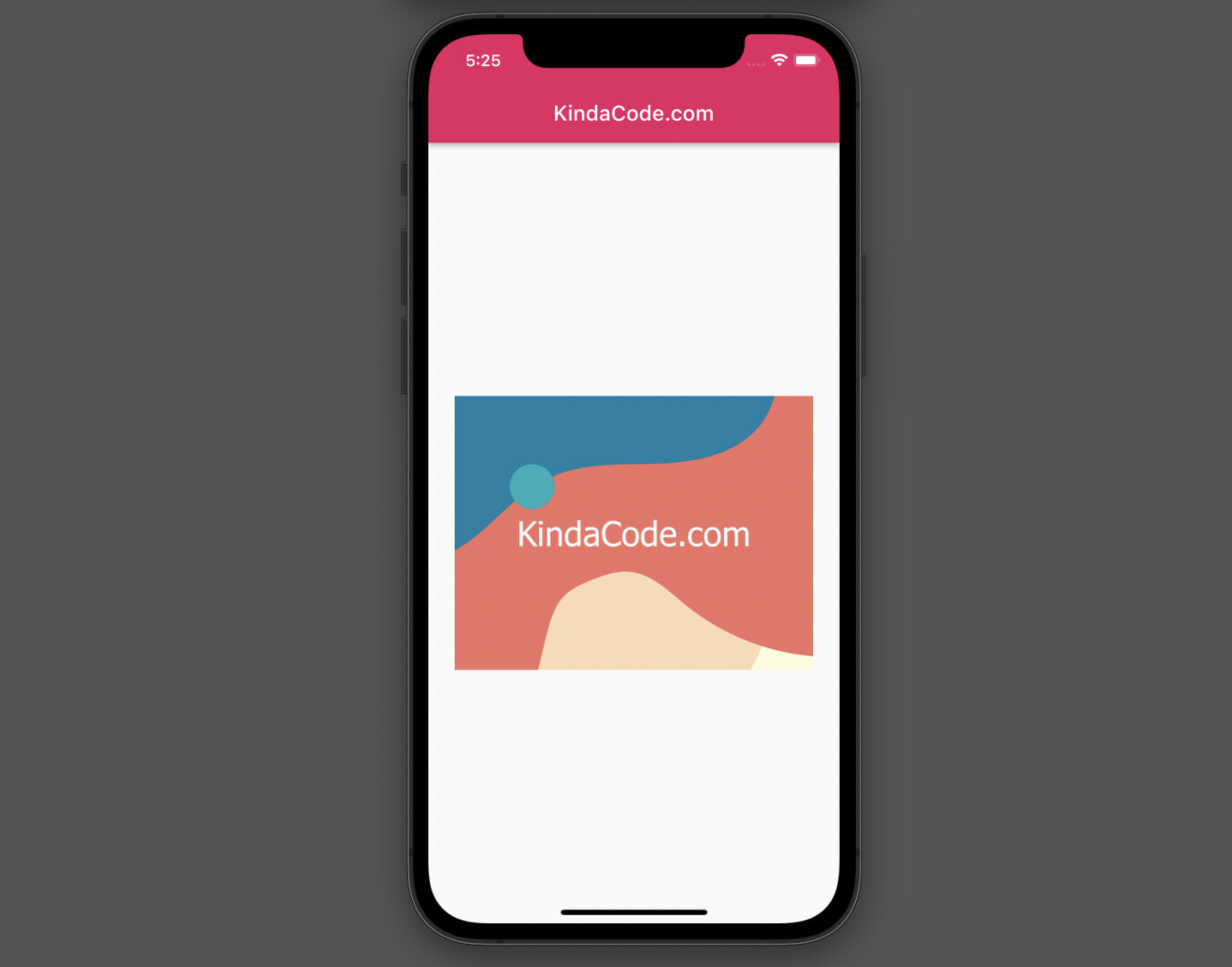
The complete code:
// main.dart
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'dart:typed_data';
void main() async {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'KindaCode.com',
theme: ThemeData(
primarySwatch: Colors.pink,
),
home: const HomeScreen(),
);
}
}
class HomeScreen extends StatefulWidget {
const HomeScreen({Key? key}) : super(key: key);
@override
State<HomeScreen> createState() => _HomeScreenState();
}
class _HomeScreenState extends State<HomeScreen> {
// this byte data will be used to render the image
Int8List? _bytes;
// get byte data from an image url
void _getBytes(imageUrl) async {
final ByteData data =
await NetworkAssetBundle(Uri.parse(imageUrl)).load(imageUrl);
setState(() {
_bytes = data.buffer.asInt8List();
// see how byte date of the image looks like
print(_bytes);
});
}
@override
void initState() {
// call the _getBytes() function
_getBytes(
'https://www.kindacode.com/wp-content/uploads/2022/07/KindaCode.jpg');
super.initState();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('KindaCode.com')),
body: Center(
child: _bytes == null
? const CircularProgressIndicator()
: Image.memory(
Uint8List.fromList(_bytes!),
width: 340,
height: 260,
fit: BoxFit.cover,
)),
);
}
}
Mastering a skill involves continuous learning and a lot of practicing. Keep your motivation and stay on track by taking a look at the following Flutter articles:
- Adding a Border to an Image in Flutter (3 Examples)
- Flutter: Caching Network Images for Big Performance gains
- Flutter: Reading Bytes from a Network Image
- How to Create Rounded ListTile in Flutter
- Flutter + Firebase Storage: Upload, Retrieve, and Delete files
- Flutter: How to Read and Write Text Files
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.