In order to add a border to a ListTile widget in Flutter, you can assign its shape property to RoundedRectangleBorder, BeveledRectangleBorder, or StadiumBorder. You have control over the thickness, color, and radius of the border. Let’s see the example below for more clarity.
Screenshot:
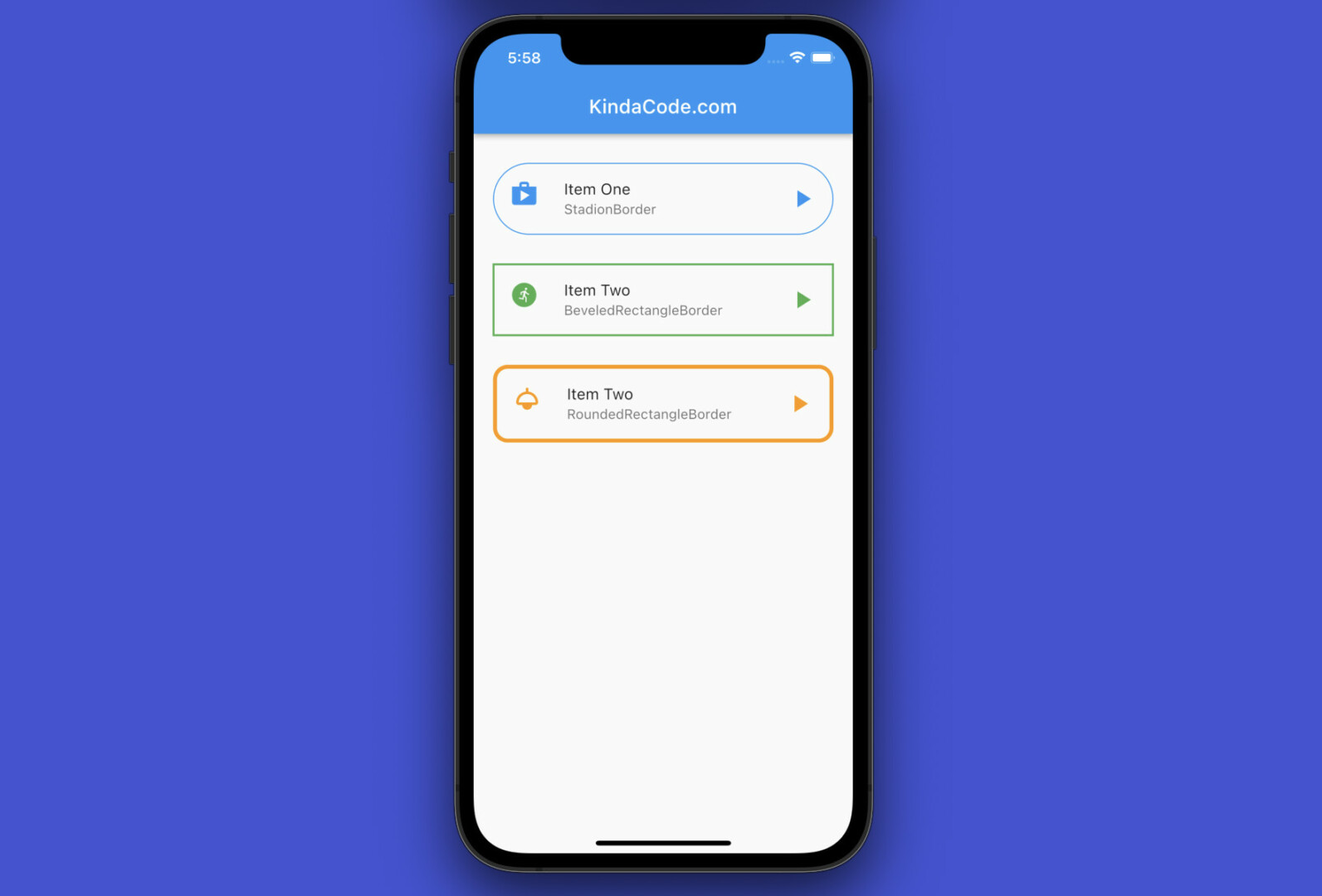
The code:
Scaffold(
appBar: AppBar(title: const Text('KindaCode.com')),
body: Padding(
padding: const EdgeInsets.symmetric(vertical: 30, horizontal: 20),
// implement add ListView that contains multiple ListTiles
child: ListView(
children: [
// using StadionBorder
const ListTile(
shape: StadiumBorder(
side: BorderSide(color: Colors.blue, width: 1),
),
iconColor: Colors.blue,
leading: Icon(
Icons.shop,
size: 30,
),
title: Text('Item One'),
subtitle: Text('StadionBorder'),
trailing: Icon(
Icons.play_arrow,
size: 30,
),
),
const SizedBox(
height: 30,
),
// Using BeveledRectangleBorder
const ListTile(
shape: BeveledRectangleBorder(
side: BorderSide(color: Colors.green, width: 1),
),
iconColor: Colors.green,
leading: Icon(
Icons.run_circle,
size: 30,
),
title: Text('Item Two'),
subtitle: Text('BeveledRectangleBorder'),
trailing: Icon(
Icons.play_arrow,
size: 30,
),
),
const SizedBox(
height: 30,
),
// Using RoundedRectangleBorder
ListTile(
shape: RoundedRectangleBorder(
side: const BorderSide(color: Colors.orange, width: 4),
borderRadius: BorderRadius.circular(15),
),
iconColor: Colors.orange,
leading: const Icon(
Icons.light,
size: 30,
),
title: const Text('Item Two'),
subtitle: const Text('RoundedRectangleBorder'),
trailing: const Icon(
Icons.play_arrow,
size: 30,
),
),
],
),
),
);
That’s it. Further reading:
- Flutter: Creating a Fullscreen Modal with Search Form
- Flutter: Best Packages to Create Cool Bottom App Bars
- How to Subtract two Dates in Flutter & Dart
- Flutter: Stream.periodic example
- Flutter + Firebase Storage: Upload, Retrieve, and Delete files
- Flutter and Firestore Database: CRUD example
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.