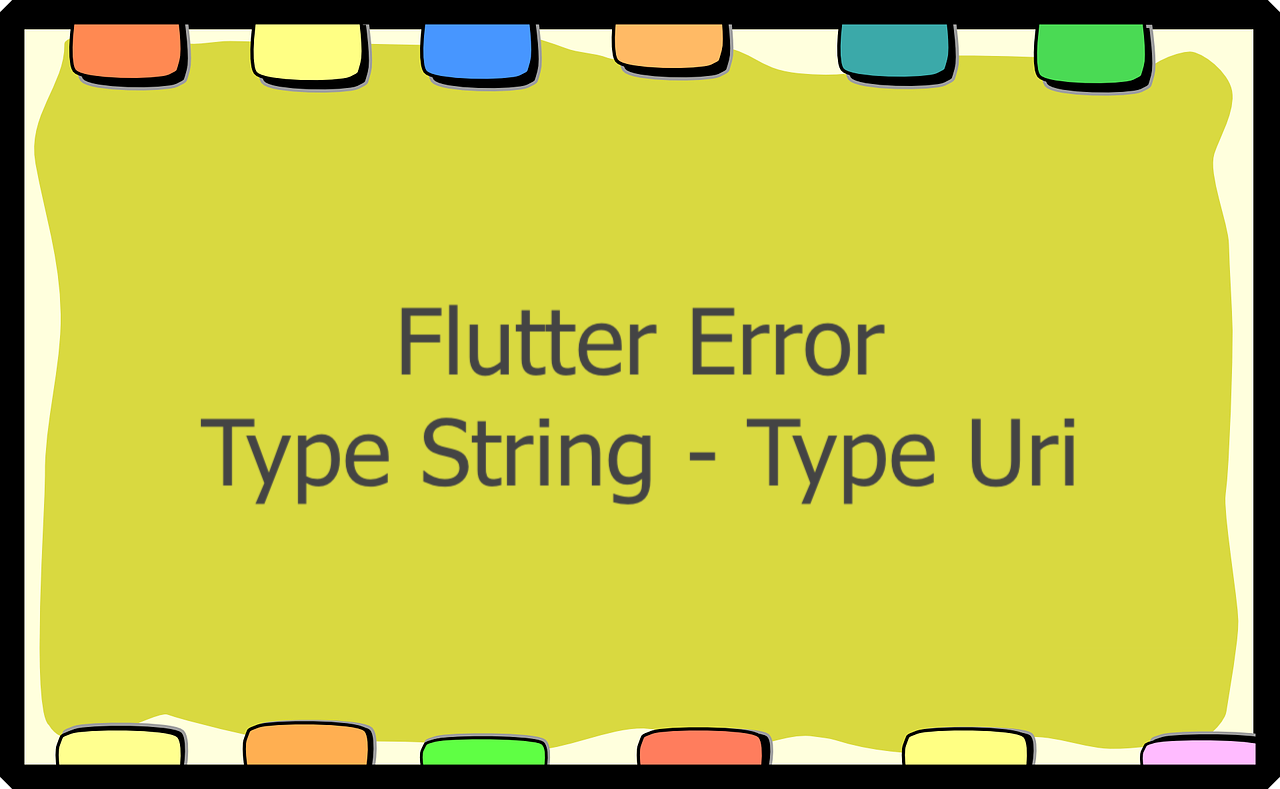
If you’re using the http package (version 0.13 or newer), you might run into the following error when sending HTTP requests:
The argument type 'String' can't be assigned to the parameter type 'Uri'
The cause of this error is that the functions provided by the http
package previously received arguments with both String
and Uri
types, but now it only accepts Uri
types.
Let’s Fix It
You can fix the mentioned issue with one line of code. By using the Uri.parse() method, you can create a new Uri
object by parsing a URI string.
Example:
import 'package:http/http.dart' as http;
/* ... */
void _sendPostRequest() async {
try {
const String apiEndpoint =
'https://api.kindacode.com/this-is-a-fiction-api-endpoint';
// Replace with your own api url
final Uri url = Uri.parse(apiEndpoint);
final response = await http.post(url);
// Do something with the response
print(response);
} catch (err) {
// Handling error
print(err);
}
}
Note that the Uri
class comes with dart:core
so you can use it right away.
Further reading:
- 2 Ways to Fetch Data from APIs in Flutter
- Best Libraries for Making HTTP Requests in Flutter
- Flutter and Firestore Database: CRUD example (null safety)
- Using GetX (Get) for Navigation and Routing in Flutter
- Using GetX (Get) for State Management in Flutter
- Flutter + Firebase Storage: Upload, Retrieve, and Delete files
You can also check out our Flutter category page, or Dart category page for the latest tutorials and examples.