This short post shows you how to change the status bar color (and control its text and icon brightness) in Flutter.
Table of Contents
iOS
On iOS, the background color of the status bar is the same as the background color of the AppBar
widget:
Scaffold(
appBar: AppBar(
backgroundColor: Colors.indigo,
title: const Text('Kindacode.com'),
),
;
Screenshot:
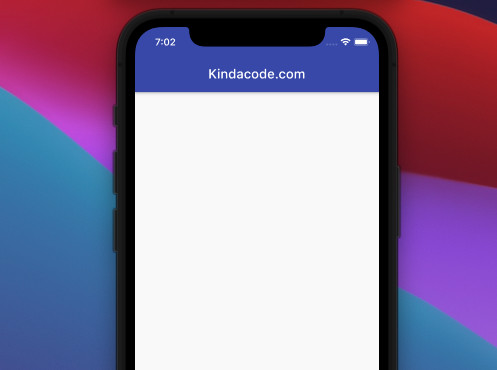
If you wrap the Scaffold
widget within a SafeArea
widget, the status bar will turn dark no matter what color the AppBar
is:
Widget build(BuildContext context) {
return SafeArea(
child: Scaffold(
appBar: AppBar(
backgroundColor: Colors.indigo,
title: const Text('Kindacode.com'),
),
),
);
}
Screenshot:
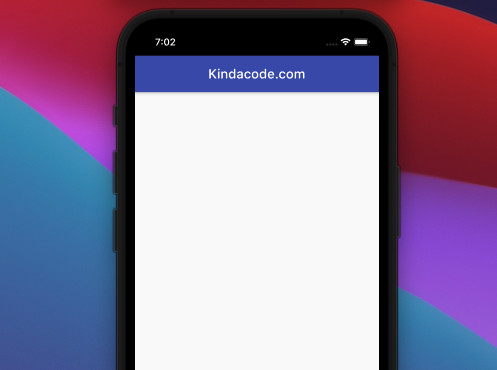
Android
On Android, you can set the color of the status bar separately from the color of AppBar
by using SystemChrome.setSystemUIOverlayStyle
like so:
// you have to import this
import 'package:flutter/services.dart';
void main() {
SystemChrome.setSystemUIOverlayStyle(
const SystemUiOverlayStyle(statusBarColor: Colors.amber));
runApp(const MyApp());
}
Text and Icons Brightness
You can control the brightness of the status bar’s text and icons (time, wifi, battery, etc) by using the systemOverlayStyle
parameter:
// text and icons will be light color
systemOverlayStyle: SystemUiOverlayStyle.dark,
// text and icons will be dark color
systemOverlayStyle: SystemUiOverlayStyle.light,
Complete Example
Screenshot:
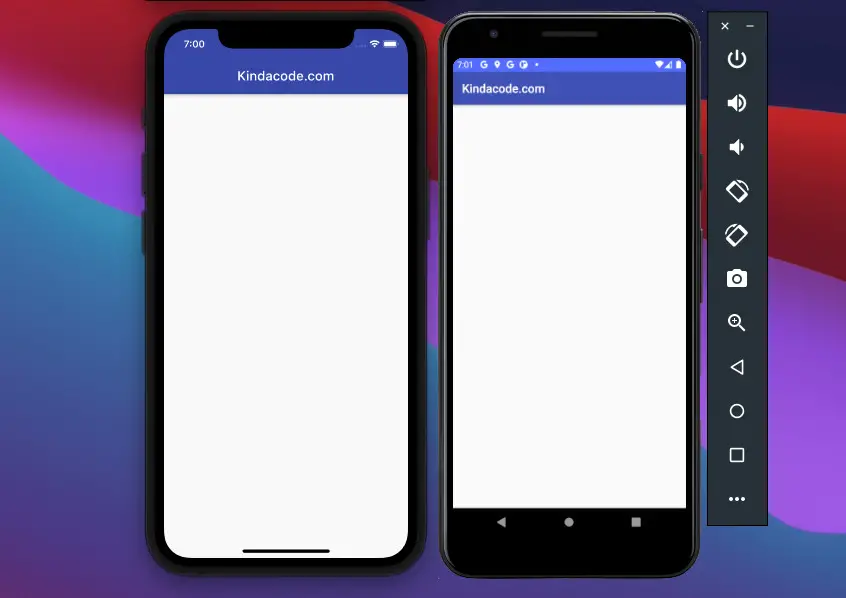
The full code:
// main.dart
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
void main() {
// set the status bar color (Android)
SystemChrome.setSystemUIOverlayStyle(
const SystemUiOverlayStyle(statusBarColor: Colors.indigoAccent));
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage());
}
}
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: Colors.indigo,
title: const Text('Kindacode.com'),
systemOverlayStyle: SystemUiOverlayStyle.light,
),
body: const Center(),
);
}
}
What’s Next?
Continue exploring more about Flutter by taking a look at the following tutorials:
- Flutter: Customize the Android System Navigation Bar
- Flutter: Hiding the Status Bar on iOS and Android
- Flutter: Global Styles for AppBar using AppBarTheme
- Example of CupertinoSliverNavigationBar in Flutter
- Flutter SliverAppBar Example (with Explanations)
- Flutter: Creating a Custom Number Stepper Input
- Flutter & Hive Database: CRUD Example (updated)
You can also check out our Flutter category page, or Dart category page for the latest tutorials and examples.