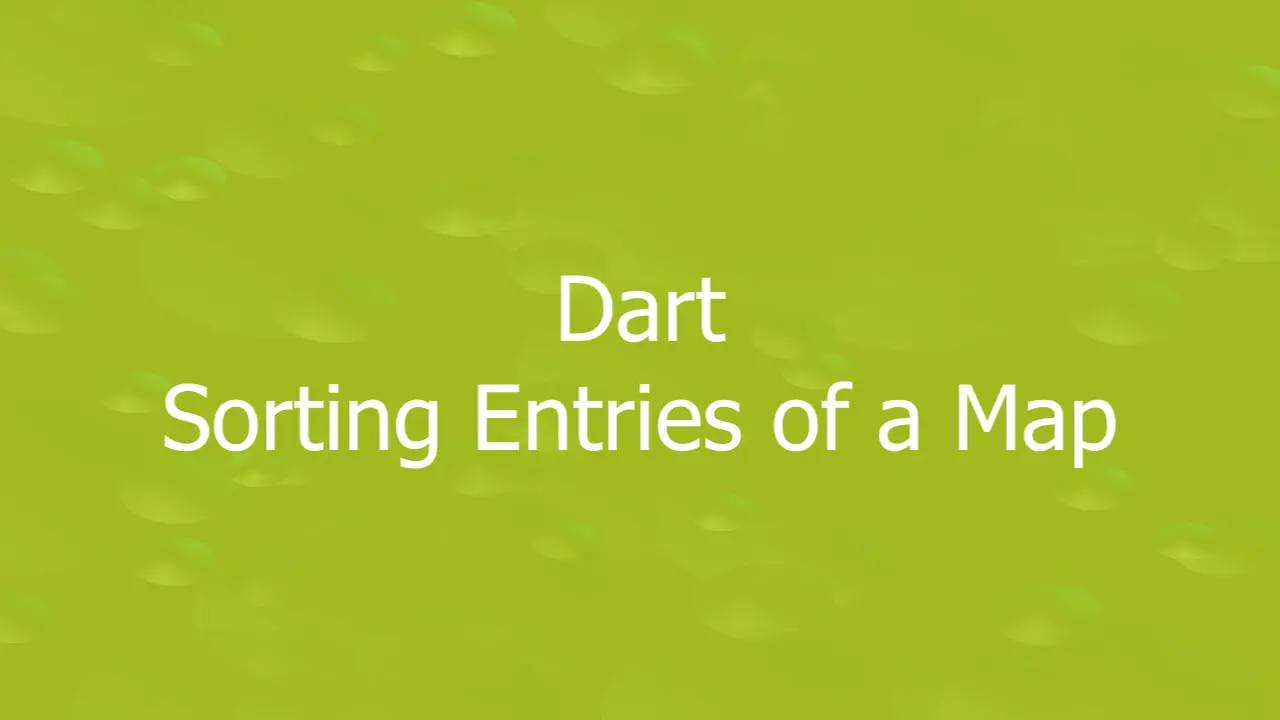
Imagine your boss gives you a Dart map like so:
final Map<String, int> myMap = {
'a': 10,
'b': 20,
'c': 15,
'd': 5,
'e': 14
};
And then, he asks you to sort the map in the ascending/descending order of the map’s values. How do you solve this problem to make him happy? Well, the solution is quite simple. Let’s see the two examples below.
Sorting the map in ascending order of its values
The code:
// KindaCode.com
void main() {
final Map<String, int> myMap = {'a': 10, 'b': 20, 'c': 15, 'd': 5, 'e': 14};
// sort the map in the ascending order of values
// turn the map into a list of entries
List<MapEntry<String, int>> mapEntries = myMap.entries.toList();
// sort the list
mapEntries.sort((a, b) => a.value.compareTo(b.value));
// turn the list back into a map
final Map<String, int> sortedMapAsc = Map.fromEntries(mapEntries);
// print the result
print(sortedMapAsc);
}
Output:
{d: 5, a: 10, e: 14, c: 15, b: 20}
Sorting the map in descending order of its values
The code is almost the same as the preceding example except that we change this line:
mapEntries.sort((a, b) => a.value.compareTo(b.value));
To this:
mapEntries.sort((a, b) => b.value.compareTo(a.value));
Here’s the full code:
// KindaCode.com
void main() {
final Map<String, int> myMap = {'a': 10, 'b': 20, 'c': 15, 'd': 5, 'e': 14};
// sort the map in the ascending order of values
// turn the map into a list of entries
List<MapEntry<String, int>> mapEntries = myMap.entries.toList();
// sort the list in descending order of values
mapEntries.sort((a, b) => b.value.compareTo(a.value));
// turn the list back into a map
final Map<String, int> sortedMapAsc = Map.fromEntries(mapEntries);
// print the result
print(sortedMapAsc);
}
Output:
{b: 20, c: 15, e: 14, a: 10, d: 5}
That’s if. Further reading:
- Flutter & Dart: Displaying Large Numbers with Digit Grouping
- Prevent VS Code from Auto Formatting Flutter/Dart Code
- Flutter & Dart: 3 Ways to Generate Random Strings
- Flutter Autocomplete example
- How to create a Filter/Search ListView in Flutter
- Using Font Awesome Icons in Flutter
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.