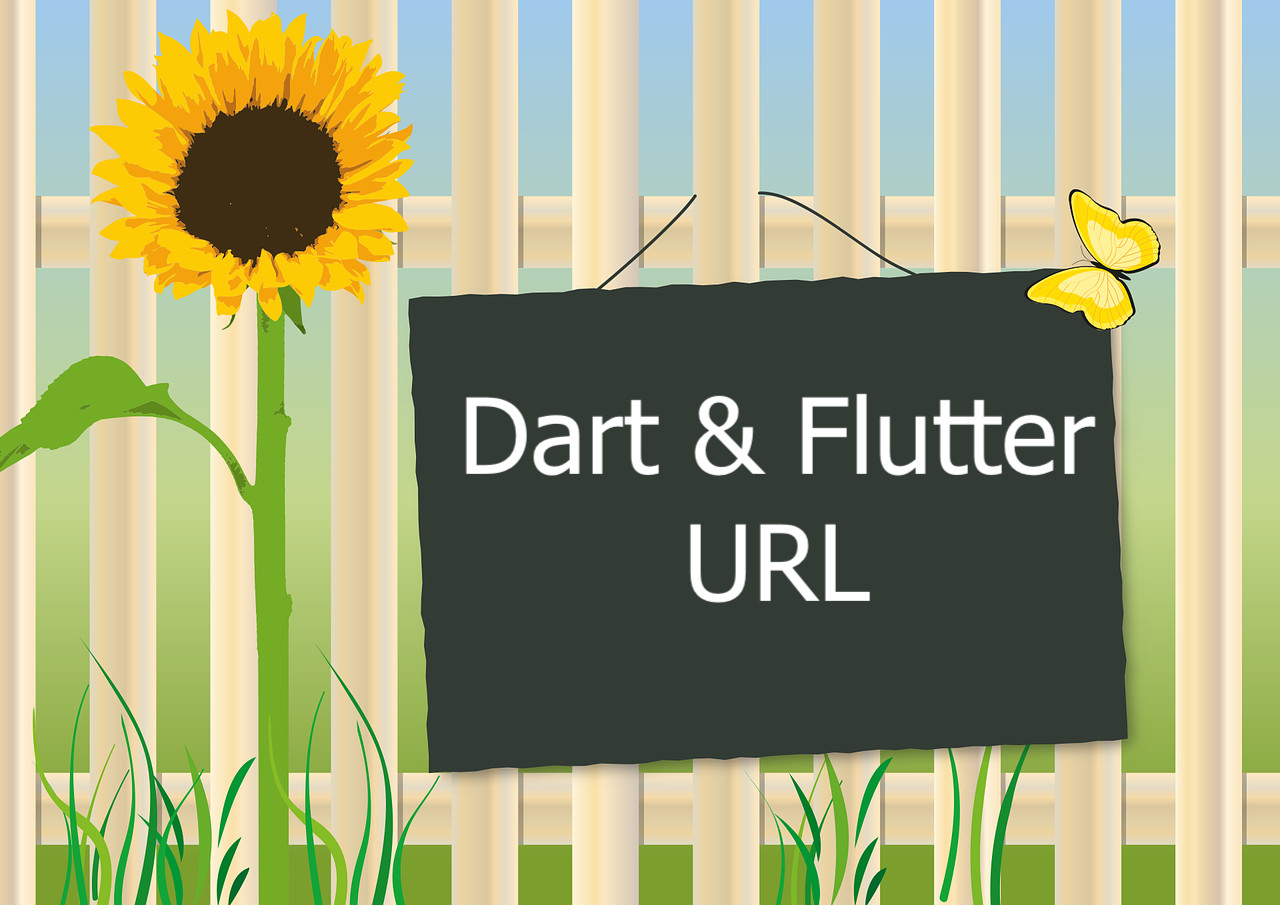
In Dart, you can extract useful information from a given URL string by following the steps below:
1. Construct a Uri object from your URL string:
const url = "...";
const uri = Uri.parse(url);
2. Now, you can get what you want through various properties of the Uri object. Below are the most commonly used:
- origin: The base URL (it looks like this: protocol://host:port)
- host: The host part of the URL (a domain, an IP address, etc)
- scheme: The protocol (http, https, etc)
- port: The port number
- path: The path
- pathSegments: The URI path split into its segments
- query: The query string
- queryParameters: The query is split into a map for easy access
You can find more details about the Uri class in the official docs.
Example:
// main.dart
void main() {
// an ordinary URL
const urlOne = 'https://www.kindacode.com/some-category/some-path/';
final uriOne = Uri.parse(urlOne);
print(uriOne.origin); // https://www.kindacode.com
print(uriOne.host); // www.kindacode.com
print(uriOne.scheme); // https
print(uriOne.port); // 443
print(uriOne.path); // /some-category/some-path/
print(uriOne.pathSegments); // [some-category, some-path]
print(uriOne.query); // null
print(uriOne.queryParameters); // {}
// a URL with query paramters
const urlTwo =
'https://test.kindacode.com/one-two-three?search=flutter&sort=asc';
final uriTwo = Uri.parse(urlTwo);
print(uriTwo.origin); // https://test.kindacode.com
print(uriTwo.host); // test.kindacode.com
print(uriTwo.scheme);
print(uriTwo.port); // 443
print(uriTwo.path); // /one-two-three
print(uriTwo.pathSegments); // [one-two-three]
print(uriTwo.query); // search=flutter&sort=asc
print(uriTwo.queryParameters); // {search: flutter, sort: asc}
}
That’s it. Further reading:
- Dart: Sorting Entries of a Map by Its Values
- How to Subtract two Dates in Flutter & Dart
- Using GetX to make GET/POST requests in Flutter
- Flutter: Creating a Fullscreen Modal with Search Form
- Flutter: Showing a Context Menu on Long Press
- TabBar, TabBarView, and TabPageSelector in Flutter
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.