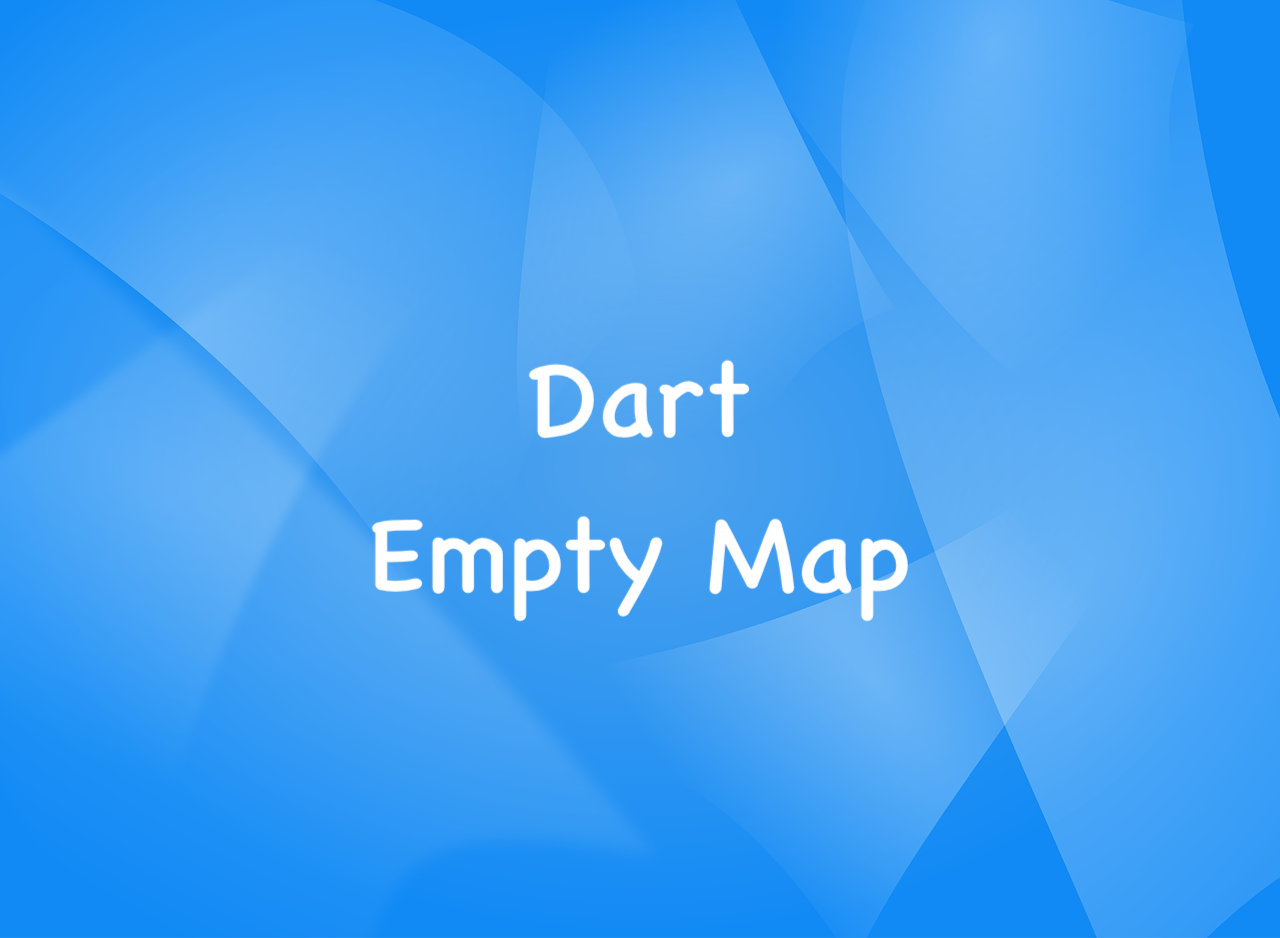
In Dart, you can check if a given map is empty or not in several ways. The most convenient approaches are to use the isEmpty, isNotEmpty, or length properties.
Example:
// main.dart
Map<String, dynamic> map1 = {'website': 'Kindacode.com', 'age': 6};
Map map2 = {};
Map map3 = {'key1': 'Value 1', 'key2': 'Value 2'};
void main() {
// Using isEmpty
if (map1.isEmpty) {
print('Map1 is empty');
} else {
print('Map1 is not empty');
}
// Using isNotEmpty
if (map2.isNotEmpty) {
print('Map2 is not empty');
} else {
print('Map2 is empty');
}
// Clear map3's entries
map3.clear();
// Using length property
if (map3.length == 0) {
print('Map3 is empty because it was cleared with the clear() method');
} else {
print('Map 3 is not empty');
}
}
Output:
Map1 is not empty
Map2 is empty
Map3 is empty because it was cleared with the clear() method
We’ve traversed an example that demonstrates more than one way to determine if a given map is empty or not. Keep going and learning by taking a look at the following articles:
- Dart: Checking if a Map contains a given Key/Value
- Dart: How to Add new Key/Value Pairs to a Map
- Dart: How to Update a Map
- Dart: How to remove specific Entries from a Map
- Dart & Flutter: 2 Ways to Count Words in a String
- Dart regex to validate US/CA phone numbers
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.