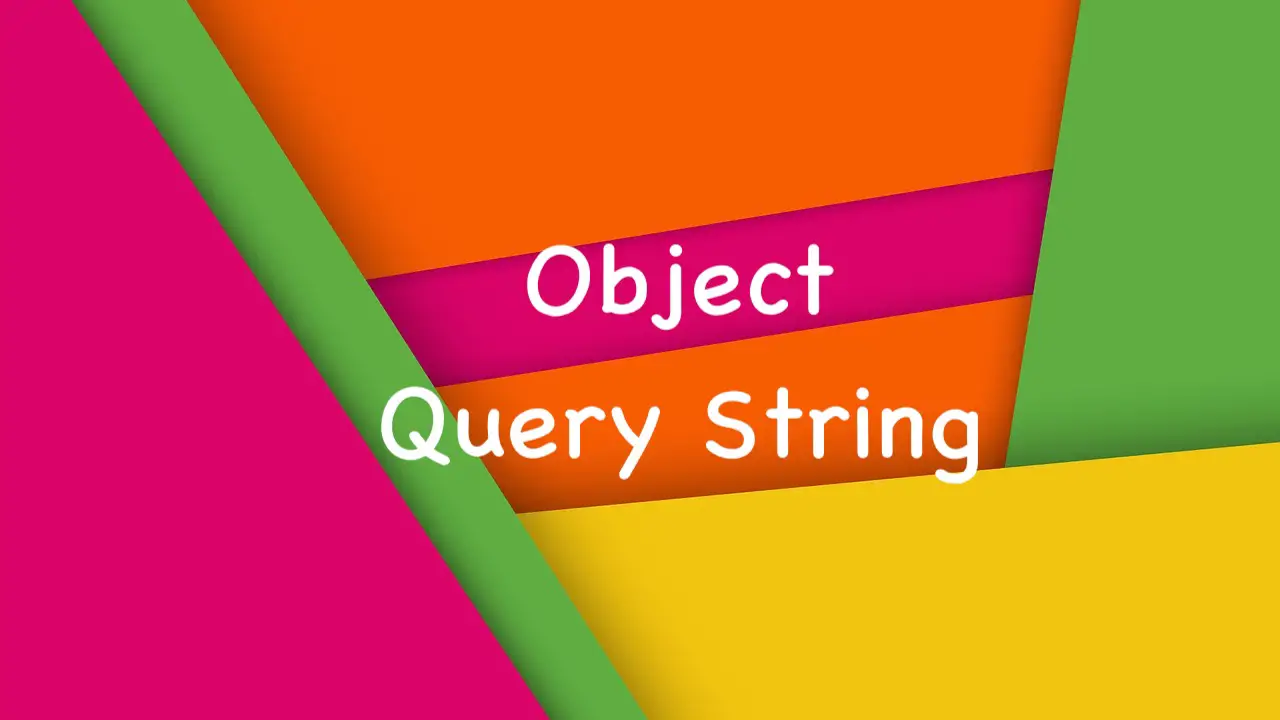
This article shows you a couple of different ways to convert (stringify) objects into query strings in Node.js.
Using URLSearchParams API
The URLSearchParams class comes with the URL module and is available on the global object so that you can use it without “require” or “import”.
Example:
// kindacode.com
const params = {
category: 'book',
language: 'en',
page: 12,
perPage: 10,
order: 'price',
orderBy: 'desc',
author: ['John Doe', 'Jane Dee']
}
const qs = new URLSearchParams(params);
console.log(qs.toString())
Output:
category=book&language=en&page=12&perPage=10&order=price&orderBy=desc&author=John+Doe%2CJane+Dee
Using querystring module
querystring is a built-in module of Node.js that can help us get the job done.
Example:
// kindacode.com
import queryString from 'querystring';
// for Commonjs, use this:
// const queryString = require('queryString');
const obj = {
name: 'John Doe',
age: 36,
skill: 'Node.js'
}
const qs = queryString.stringify(obj)
console.log(qs)
Output:
name=John%20Doe&age=36&skill=Node.js
The querystring API works fine and is still maintained but it is considered legacy. The official documentation recommends using URLSearchParams (the first approach) instead.
Writing your own function
With a few lines of code, we can write a function to stringify an object.
Example:
// kindacode.com
// define the functio
const obj2qs = (inputObj) =>
Object.entries(inputObj)
.map((i) => [i[0], encodeURIComponent(i[1])].join("="))
.join("&");
// Try it
const obj = {
x: 100,
y: 'yyyyy',
z: 'ZzZz'
}
const result = obj2qs(obj)
console.log(result)
Output:
x=100&y=yyyyy&z=ZzZz
Using a third-party library
There’re several open-source packages that can easily stringify an object in Node.js. A popular name is querystringify.
Install it by running:
npm install querystringify --save
Example:
import querystringify from 'querystringify';
// for CommonJS, use this:
// const querystringify = require('querystringify');
const obj = {
foo: 'abc',
bar: '123'
}
const qs = querystringify.stringify(obj)
console.log(qs);
Output:
foo=abc&bar=123
Conclusion
You’ve learned more than one approach to turning objects into query strings in Node.js. Keep the ball rolling and continue exploring more interesting stuff by taking a look at the following articles:
- Node.js: Get File Name and Extension from Path/URL
- Node.js: Listing Files in a Folder
- Node.js: Ways to Create a Directory If It Doesn’t Exist
- Using Axios to download images and videos in Node.js
- Top Node.js Open Source Headless CMS
- Best Node.js frameworks to build backend APIs
You can also check out our Node.js category page for the latest tutorials and examples.