The steps below show you how to display local images in Flutter:
1. Create a folder in your project root directory and name it “images” (the name doesn’t matter, you can name it “assets” or whatever name that makes sense).
2. Copy all of the images you will use in your application to the “images” folder:
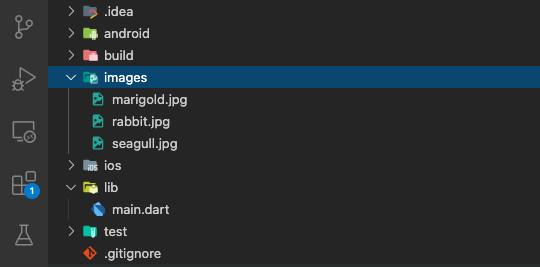
3. Open the pubspec.yaml file and add an “assets” section with the “images” directory inside the “flutter” section, like so:
flutter:
assets:
- images/
Note: Specify the directory name with the “/” character at the end to identify images required by your app. For convenience, you don’t have to declare all the image names but keep in mind that only files located directly in the directory are included. To add files located in subdirectories, create an entry per directory.
4. Using images
To use local images in Flutter, we use the Image widget like so:
Image.asset('image-path')
Full Example:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Flutter Example',
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Flutter Example'),
),
body: Column(
// Make sure you write the correct image paths
children: [
// simple
Image.asset('images/marigold.jpg'),
// add some options
Image.asset(
'images/rabbit.jpg',
width: 200,
height: 200,
)
]),
);
}
}
Screenshot:
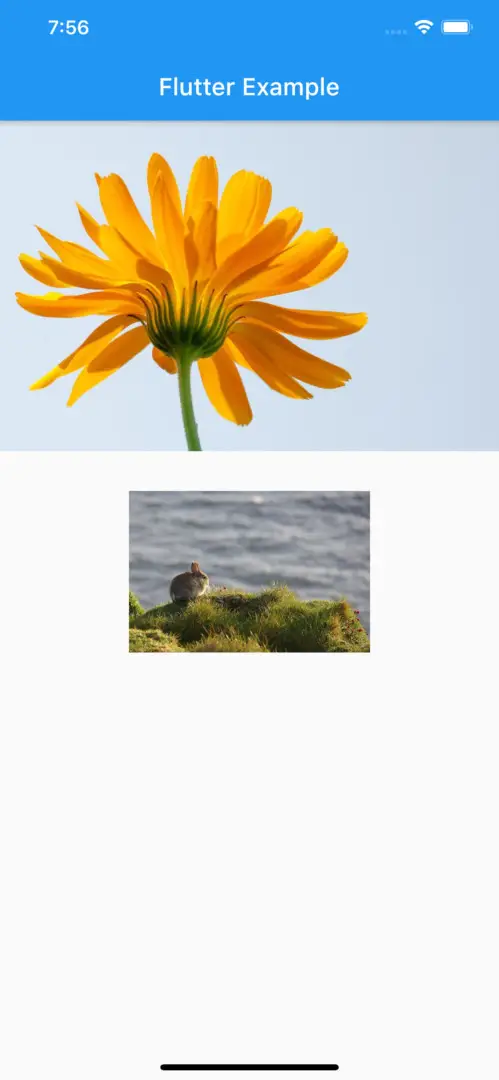
Note: After adding new images or editing the pubspec.yaml file, you should completely restart your app. A hot reload (r) or a hot restart (R) isn’t enough.
Further reading:
- Create a Custom NumPad (Number Keyboard) in Flutter
- Flutter & Hive Database: CRUD Example
- Flutter: Firebase Remote Config example
- Creating Masonry Layout in Flutter with Staggered Grid View
- Flutter: Creating Custom Back Buttons
- Hero Widget in Flutter: Tutorial & Example
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.