In Flutter, the ErrorWidget widget is used to render an error message when a build method falls. You can easily implement an ErrorWidget by adding ErrorWidget.builder to the main() function like this:
void main() {
ErrorWidget.builder = (FlutterErrorDetails details) {
return <your widget>;
};
return runApp(MyApp());
}
For more clarity, please see the example below.
Example
In this example, we’ll create a fake error by doing this:
@override
void initState() {
super.initState();
throw ("Hi there. I am an error");
}
Screenshots
Without WidgetError, you’ll see a red screen with yellow text when the error occurs. By using WidgetError, you can display anything you want when your Flutter app runs into a problem:
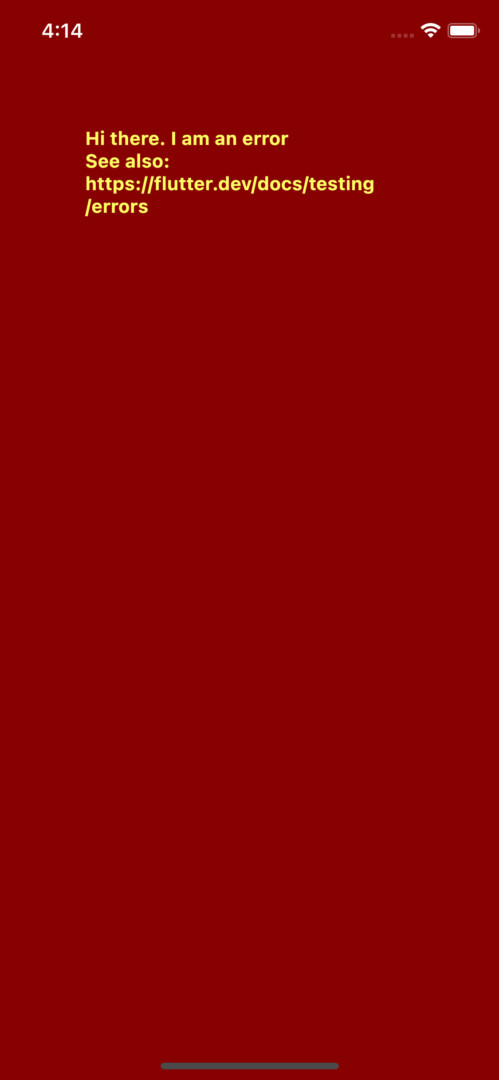
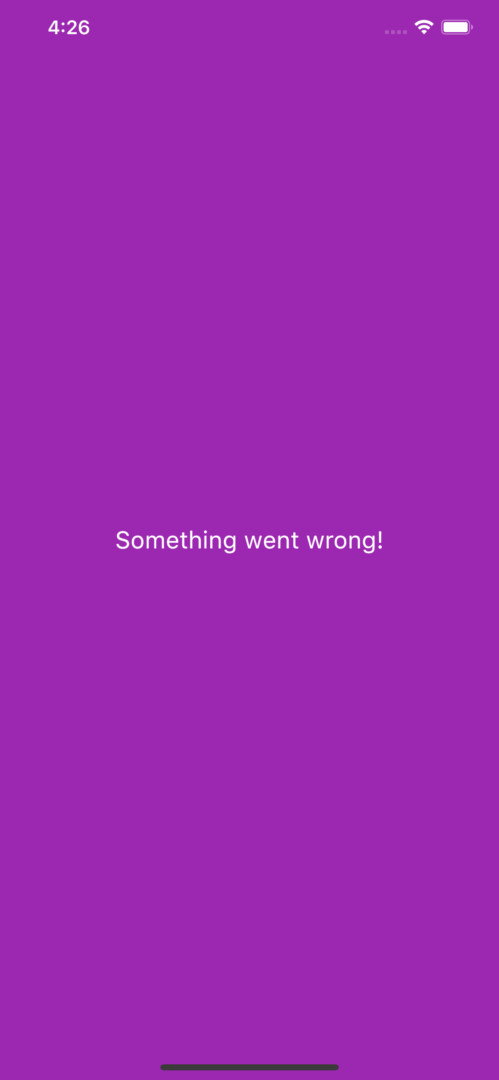
The console will display additional information about the error as default.
The complete code:
// main.dart
import 'package:flutter/material.dart';
void main() {
ErrorWidget.builder = (FlutterErrorDetails details) {
return Material(
child: Container(
color: Colors.purple,
alignment: Alignment.center,
child: const Text(
'Something went wrong!',
style: TextStyle(fontSize: 20, color: Colors.white),
),
),
);
};
return runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
@override
void initState() {
super.initState();
// throw a custom error
throw ("Hi there. I am an error");
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: const Center());
}
}
Afterword
We’ve created a small app that demonstrates an elegant way to handle errors in Flutter. At this point, you should have a better understanding of the ErrorWidget. If you’d like to explore more new and interesting stuff about Flutter and Dart, take a look at the following articles:
- How to make an image carousel in Flutter
- How to implement Star Rating in Flutter
- Flutter: Making a Tic Tac Toe Game from Scratch
- Flutter & SQLite: CRUD Example
- Flutter and Firestore Database: CRUD example
- Flutter: CupertinoPicker Example
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.