
A switch (also called a toggle) is an interactive component that lets users select between a pair of opposing values (e.g. on/off, true/false, light/dark) and has different appearances to distinguish the choice. This article shows you how to create an iOS-style switch in Flutter by using the CupertinoSwitch
widget.
Overview
To use the CupertinoSwitch widget, you must import the Cupertino library:
import 'package:flutter/cupertino.dart';
The CupertinoSwitch widget requires 2 parameters:
value
(bool): The state of the widgetonChanged
: This callback function is triggered when the user changes the switch
Other parameters are optional.
Constructor:
CupertinoSwitch({
Key? key,
required bool value,
required ValueChanged<bool>? onChanged,
Color? activeColor,
Color? trackColor,
Color? thumbColor,
bool? applyTheme,
Color? focusColor,
FocusNode? focusNode,
ValueChanged<bool>? onFocusChange,
bool autofocus = false,
DragStartBehavior dragStartBehavior = DragStartBehavior.start
})
Example
App Preview
The tiny app we’re going to make contains 2 switches. The first one, whose active color is green (this is the default color), controls the existence of the green box. The second switch, whose active color is blue, controls the existence of the blue box.
Here’s how it works:
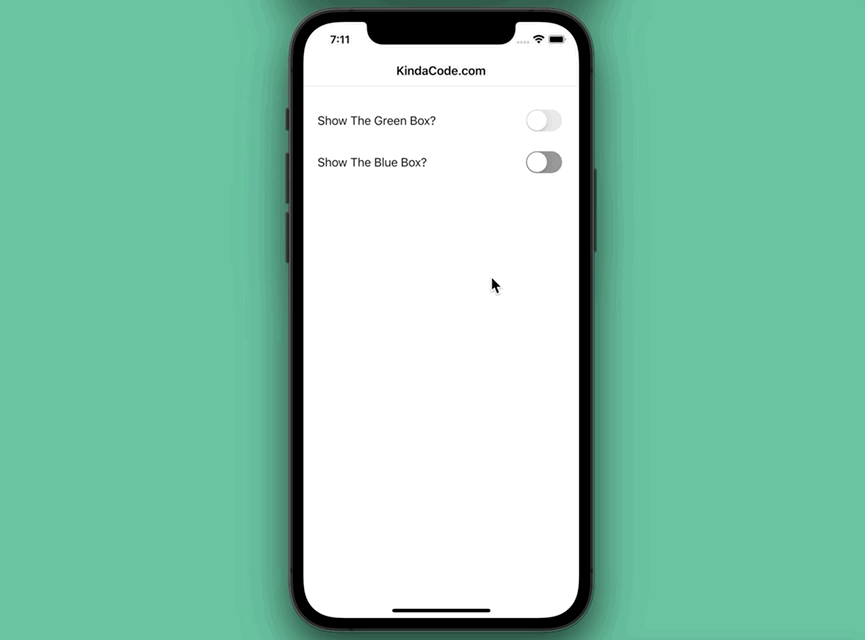
The Code
The full source code in main.dart
with explanations in the comments:
// main.dart
import 'package:flutter/cupertino.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const CupertinoApp(
// hide the debug banner
debugShowCheckedModeBanner: false,
title: "Kindacode.com",
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
// This variable stores the value of the first switch
// and controls the visibility of the green box
bool _showGreenBox = false;
// This variable stores the value of the second switch
// and controls the visibility of the blue box
bool _showBlueBox = false;
@override
Widget build(BuildContext context) {
return CupertinoPageScaffold(
navigationBar: const CupertinoNavigationBar(
middle: Text("KindaCode.com"),
),
child: Padding(
padding: const EdgeInsets.all(20),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
const SizedBox(
height: 100,
),
Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
const Text('Show The Green Box?'),
// This switch controls the existence of the green box
CupertinoSwitch(
value: _showGreenBox,
onChanged: (value) {
setState(() {
_showGreenBox = value;
});
},
),
],
),
const SizedBox(
height: 20,
),
Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
const Text('Show The Blue Box?'),
// this switch controls the existence of the blue box
CupertinoSwitch(
activeColor: CupertinoColors.activeBlue,
trackColor: CupertinoColors.inactiveGray,
thumbColor: CupertinoColors.white,
value: _showBlueBox,
onChanged: (value) {
setState(() {
_showBlueBox = value;
});
},
),
],
),
const SizedBox(
height: 30,
),
// The Green Box
if (_showGreenBox)
Container(
width: double.infinity,
height: 200,
color: CupertinoColors.activeGreen,
),
// The Blue Box
if (_showBlueBox)
Container(
margin: const EdgeInsets.only(top: 30),
width: double.infinity,
height: 200,
color: CupertinoColors.activeBlue,
),
],
),
),
);
}
}
Afterword
You’ve learned the fundamentals of the CupertinoSwitch
widget. You might need to use it a lot if you have a plan to develop an app that focuses on iOS users. Flutter is awesome and there are many things to learn about. Continue gaining more experience and strengthen your skills by taking a look at the following articles:
- Working with ListWheelScrollView in Flutter (2 Examples)
- Using GetX (Get) for Navigation and Routing in Flutter
- Using GetX (Get) for State Management in Flutter
- Flutter and Firestore Database: CRUD example
- Flutter + Firebase Storage: Upload, Retrieve, and Delete files
- How to create selectable text in Flutter
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.