This practical article walks you through a complete example that demonstrates how to implement a complex dialog in Unity by using the built-in EditorUtility.DisplayDialogComplex static function. Without any further ado, let’s get our hands dirty.
Table of Contents
A Quick Note
The DisplayDialogComplex static function of the EditorUtility class can take up to 5 parameters:
- title (String): the title of the dialog
- message (String): the content of the dialog
- ok (String): the text on the dialog function chosen button
- cancel (String): the text on the dialog cancel button
- alt (String): the text on the alternative purpose button
The Example
Screenshot:
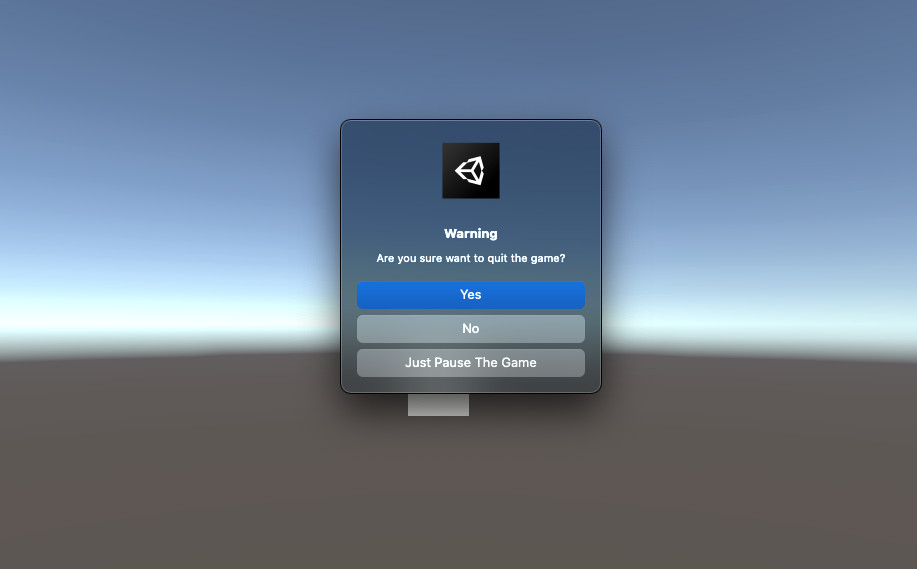
The code:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEditor;
public class ComplexDialog : MonoBehaviour
{
void Start()
{
// Call the ShowDialog function after 3 seconds
Invoke("ShowDialog", 3.0f);
}
void ShowDialog()
{
int selectedOption = EditorUtility.DisplayDialogComplex(
title: "Warning",
message: "Are you sure want to quit the game?",
ok: "Yes",
cancel: "No",
alt: "Just Pause The Game"
);
if (selectedOption == 0)
{
Debug.Log("You chose to quit the game");
}
else if (selectedOption == 1)
{
Debug.Log("You chose to continue playing the game");
}
else if (selectedOption == 2)
{
Debug.Log("You chose to pause the game. You can resume it anytime");
}
}
}
Conclusion
We’ve examined an example of implementing a complex dialog in Unity. If you’d like to explore more interesting stuff about game development, take a look at the following articles:
- Unity – How to Show a Confirmation Dialog
- Unity – How to Run a Function after a Delay
- Unity – Programmatically Enable/Disable a Script Component
- Unity – Programmatically Count the Scenes in Build Settings
- C#: How to Convert a Numeric String to Int/Double
You can also check out our C Sharp and Unity 3d topic page to see the latest tutorials and examples.