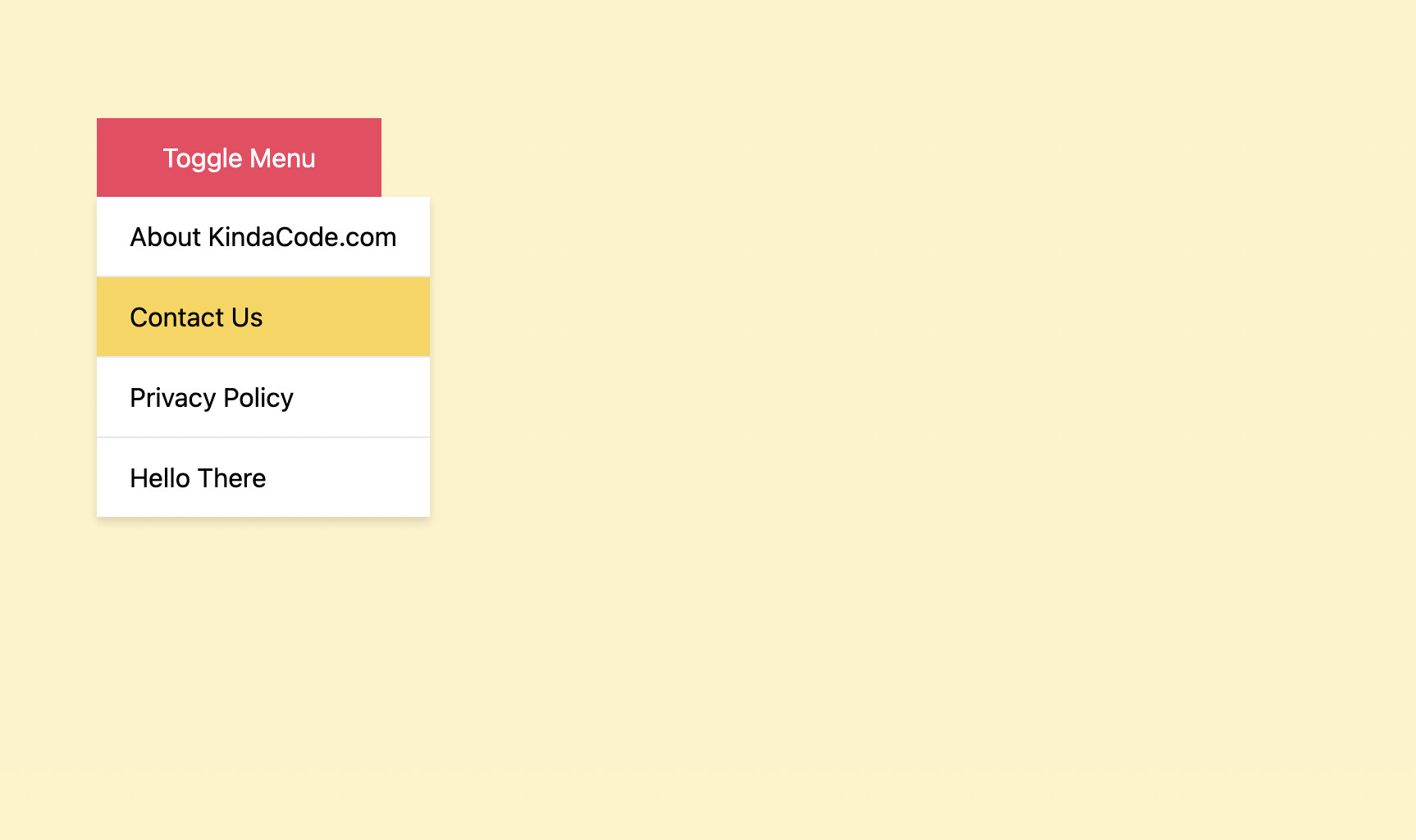
This short and straightforward guide shows you how to create a dropdown menu that can be opened or closed by mouse clicking (not mouse hovering). We’ll make it by using Tailwind CSS and a little vanilla Javascript (just a few lines).
Table of Contents
Example Preview
Our dropdown menu contains some links. In order to open the menu, you can click on the Toggle Menu button. To close the menu, there are two options:
- Use the Toggle Menu button
- Clicking outside of the menu area
Here’s how it works:
The Code
Here’s the code that produces the demo above:
<body class="p-20 bg-amber-100">
<div id="dropdown-wrapper" class="inline-block">
<button onclick="toggleMenu()"
class="px-10 py-3 bg-amber-500 hover:bg-amber-600 focus:bg-rose-500 text-white">
Toggle Menu</button>
<div id="menu" class="hidden flex flex-col bg-white drop-shadow-md">
<a class="px-5 py-3 hover:bg-amber-300 border-b border-gray-200" href="#">About KindaCode.com</a>
<a class="px-5 py-3 hover:bg-amber-300 border-b border-gray-200" href="#">Contact Us</a>
<a class="px-5 py-3 hover:bg-amber-300 border-b border-gray-200" href="#">Privacy Policy</a>
<a class="px-5 py-3 hover:bg-amber-300" href="#">Hello There</a>
</div>
</div>
<!-- Javascript code -->
<script>
var menu = document.getElementById("menu");
// open/close the menu when the user clicks on the button
function toggleMenu() {
if (menu.classList.contains('hidden')) {
menu.classList.remove('hidden');
} else {
menu.classList.add('hidden');
}
}
// close the menu when the user clicks outside of it
window.onclick = function (event) {
var dropdownWrapper = document.getElementById('dropdown-wrapper');
if (!dropdownWrapper.contains(event.target) && !menu.classList.contains('hidden')) {
menu.classList.add('hidden');
}
}
</script>
</body>
Explanations
In the beginning, the dropdown menu is hidden because we added the hidden utility class to it.
The toggleMenu() function is used to open and close the dropdown menu. It is called when the user clicks on the Toggle Menu button. To show the menu, we remove the hidden class from it:
menu.classList.remove('hidden');
To hide the menu, we add the hidden class back:
menu.classList.add('hidden');
To close the menu when the user clicks outside of it, we use this:
window.onclick = function (event) {
var dropdownWrapper = document.getElementById('dropdown-wrapper');
if (!dropdownWrapper.contains(event.target) && !menu.classList.contains('hidden')) {
menu.classList.add('hidden');
}
}
Conclusion
You’ve learned how to implement a clickable dropdown menu with Tailwind CSS and plain Javascript. If you’d like to explore more new and interesting things about modern frontend development, take a look at the following articles:
- Tailwind CSS: Create a Floating Input Label (Simplest Way)
- Tailwind CSS: Create an Animated & Closable Side Menu
- Text Overflow in Tailwind CSS (with Examples)
- Tailwind CSS: Create a Fixed/Sticky Footer Menu
- Tailwind CSS: How to Create a Fixed Top Menu Bar
- Tailwind CSS: How to Create Parallax Scrolling Effect
You can also check out our CSS category page for the latest tutorials and examples.