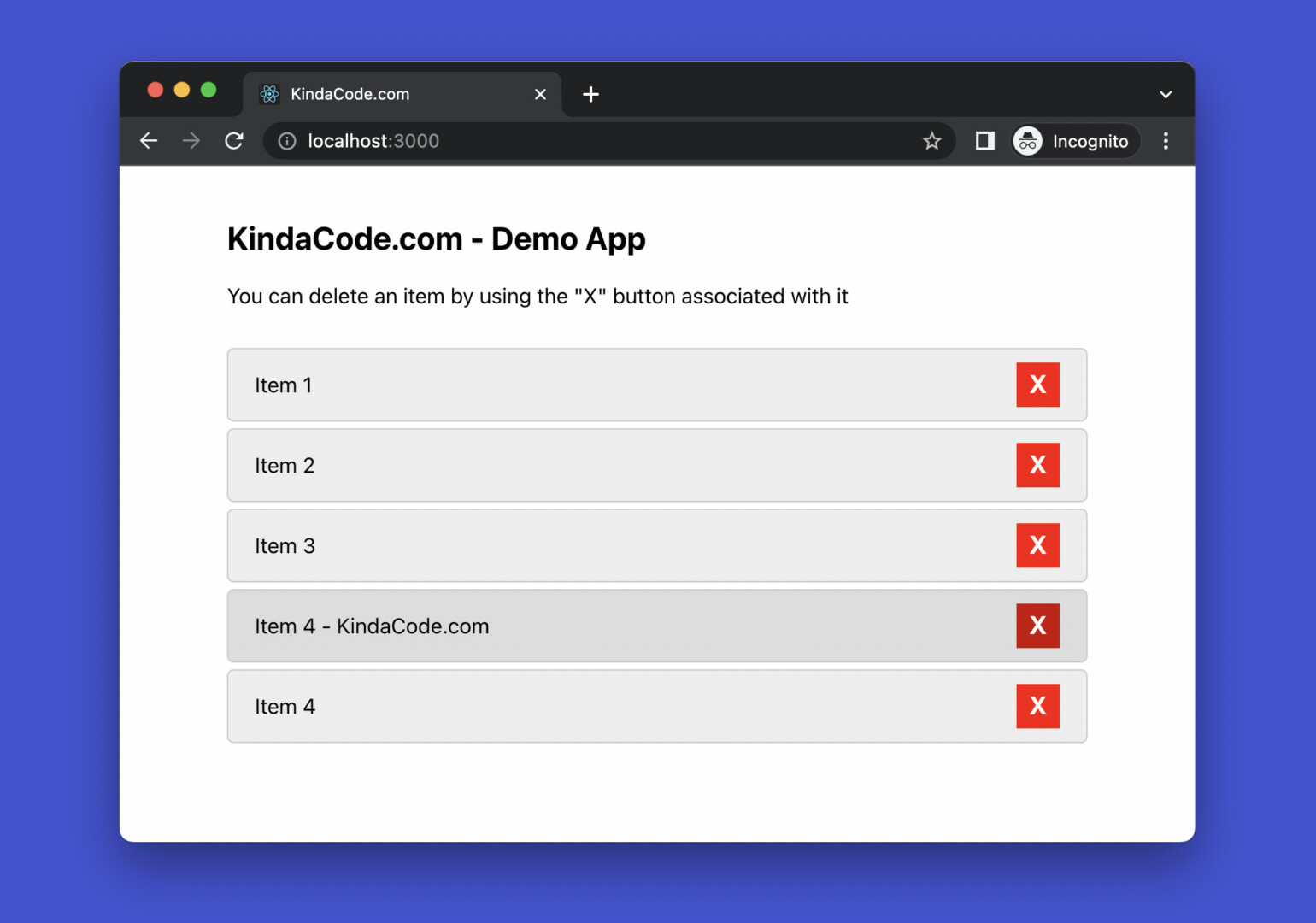
When developing web apps with React, there are innumerable cases where we have to render a list of things, such as a list of users, a list of tasks, a list of products, etc. In these situations, you might want to add a delete button to each item in the list so that people can discard specific ones that they no longer need.
This practical article walks you through a complete example of removing items from a list in React. We’ll use the useState hook and function components.
Table of Contents
A Quick Note
Let’s say we have an array of objects and each object has a property called id. To remove the object associated with a given id, we can use the Array.filter() method:
const updatedList = items.filter((item) => item.id !== id);
Let’s see how we’ll apply this in React.
The Example
App Preview
The demo we’re going to make renders a list of items. Each item goes with an X button (with a red background color), which can be used to delete the corresponding item.
Here’s how it works:
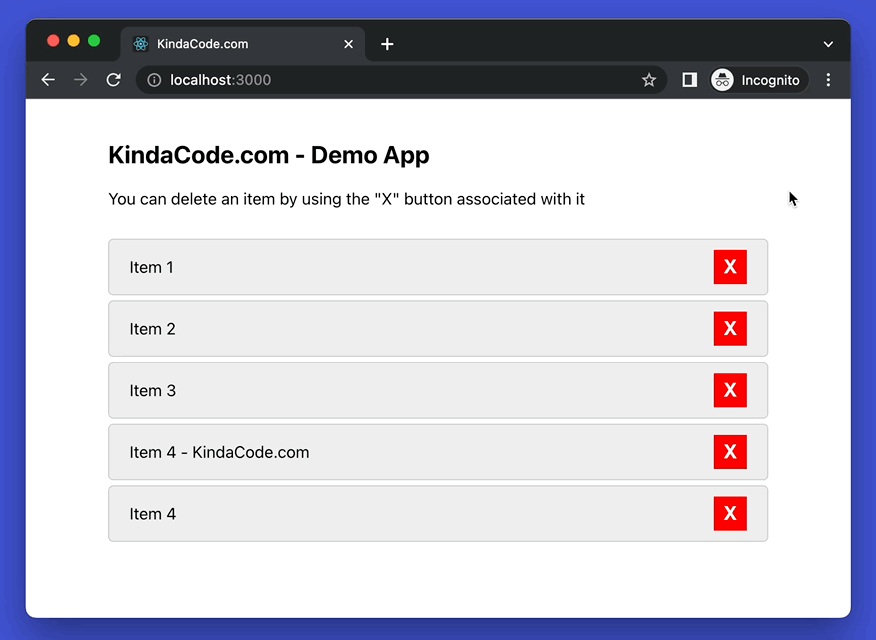
The Steps
1. Create a new React project:
npx create-react-app kindacode-list-items example
2. The full source code in src/App.js:
// KindaCode.com
// src/App.js
import { useState } from 'react';
import './App.css';
function App() {
// Declare the state variable, which we'll call "items"
const [items, setItems] = useState([
{ id: 1, name: 'Item 1' },
{ id: 2, name: 'Item 2' },
{ id: 3, name: 'Item 3' },
{ id: 5, name: 'Item 4 - KindaCode.com' },
{ id: 4, name: 'Item 4' },
]);
// Delete the item with the given id
// This function will be called when an "X" button is clicked
const removeItem = (id) => {
const updatedList = items.filter((item) => item.id !== id);
setItems(updatedList);
};
return (
<div className='container'>
<h2>KindaCode.com - Demo App</h2>
<p>You can delete an item by using the "X" button associated with it</p>
{/* Implement the list */}
<div className='list'>
{items.map((item) => (
<div className='item' key={item.id}>
<span className='item-name'>{item.name}</span>
<button
className='delete-button'
onClick={() => removeItem(item.id)}
>
X
</button>
</div>
))}
</div>
</div>
);
}
export default App;
3. The CSS code for src/App.js:
/* KindaCode.com */
/* src/App.css */
.container {
width: 80%;
margin: 40px auto;
}
.list {
margin-top: 30px;
}
.item {
margin: 5px 0px;
padding: 10px 20px;
display: flex;
justify-content: space-between;
align-items: center;
background: #eee;
border: 1px solid #ccc;
border-radius: 5px;
}
.item:hover {
background: #ddd;
}
.item-name {
font-size: 16px;
}
.delete-button {
padding: 6px 10px;
background: red;
color: #fff;
font-weight: bold;
font-size: 19px;
border: none;
cursor: pointer;
}
.delete-button:hover {
background: #c00;
}
4. Launch the app:
npm start
Afterword
We’ve examined an end-to-end, thorough example that demonstrates how to eliminate items from a list. This is the foundation of knowledge that is indispensable when constructing modern web apps.
If you would like to explore more new and exciting stuff in React development, take a look at the following articles:
- React: Programmatically Scroll to Bottom/Top of a Div
- React + TypeScript: Making a Custom Context Menu
- React + TypeScript: Making a Reading Progress Indicator
- React + TypeScript: Image onLoad & onError Events
- React: Update Arrays and Objects with the useState Hook
- React Router: How to Highlight Active Link
You can also check our React category page and React Native category page for the latest tutorials and examples.