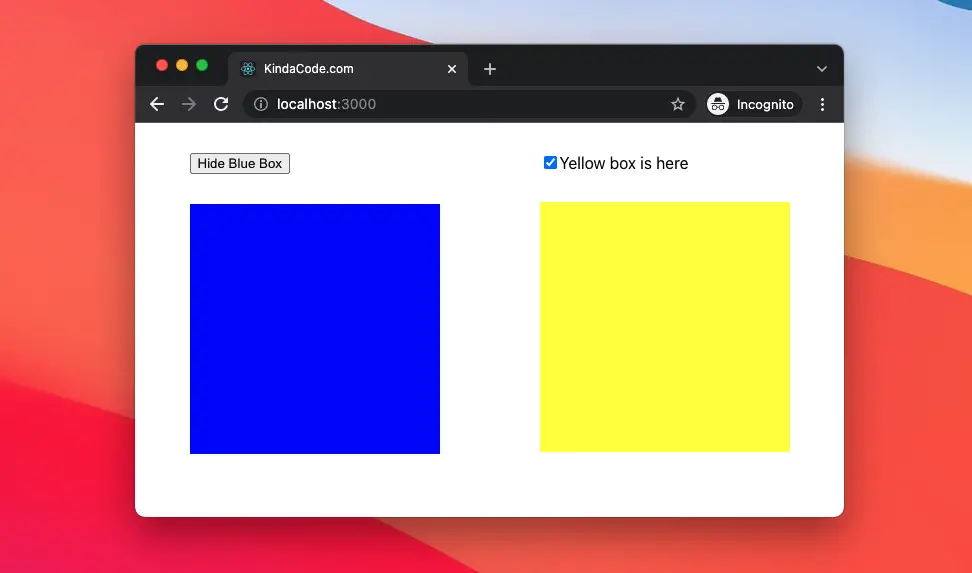
This article walks you through a complete example of programmatically showing and hiding components based on conditions in React. Without any further ado (such as singing about the history of React or how rapidly it evolves), let’s get our hands dirty.
Table of Contents
The Example
Preview
The app we are going to make contains a button, a checkbox, a blue square box, and a yellow square box.
- The button is used to control the presence of the blue box.
- The yellow box will show up when the checkbox is checked. Otherwise, it cannot be seen.
A demo is worth more than thousands of words:
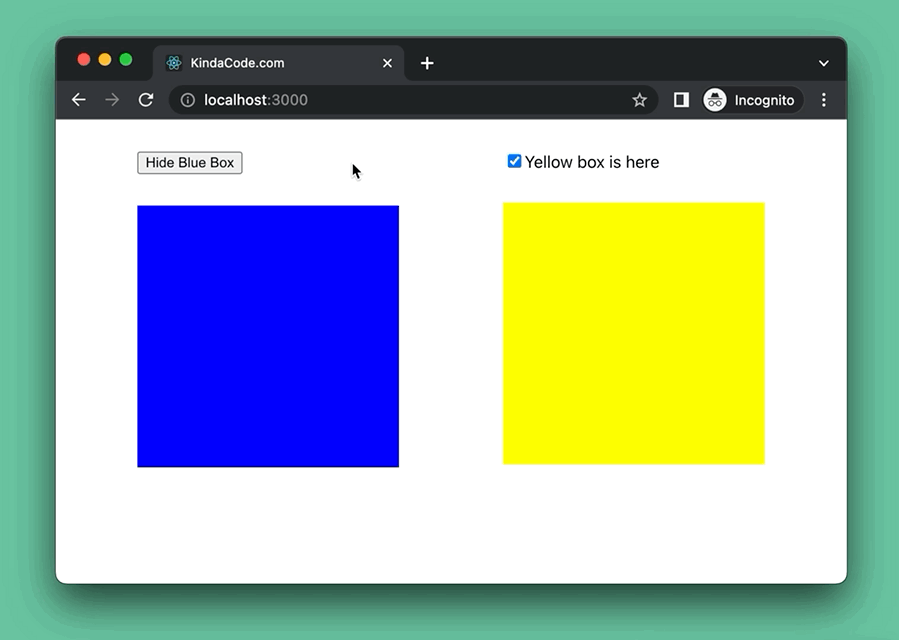
The Code
1. Create a new React project:
npx create-react-app my_app
2. Replace all of the unwanted code in src/App.js with the code below:
// App.tsx
// KindaCode.com
import React, { useState } from "react";
import "./App.css";
const App = () => {
const [iseBlueBoxShown, setIsBlueBoxShown] = useState(true);
const [isYellowBoxShown, setIsYellowBoxShown] = useState(true);
// This function is triggered when the button is clicked
const buttonHandler = () => {
setIsBlueBoxShown(!iseBlueBoxShown);
};
// This function is triggered when the checkbox changes
const checkboxHandler = () => {
setIsYellowBoxShown(!isYellowBoxShown);
};
return (
<div className="container">
<div>
<button onClick={buttonHandler}>
{iseBlueBoxShown ? "Hide Blue Box" : "Show Blue Box"}
</button>
{iseBlueBoxShown && <div className="blue-box"></div>}
</div>
<div>
<input
type="checkbox"
checked={isYellowBoxShown}
onChange={checkboxHandler}
/>
<span>
{isYellowBoxShown
? "Yellow box is here"
: "Yellow box has gone away"}
</span>
{isYellowBoxShown && <div className="yellow-box"></div>}
</div>
</div>
);
};
export default App;
3. Remove all the unwanted code in src/App.css and add this:
/*
App.css
KindaCode.com
*/
.container {
max-width: 600px;
margin: 30px auto;
display: flex;
justify-content: space-between;
}
.blue-box {
margin-top: 30px;
width: 250px;
height: 250px;
background: blue;
}
.yellow-box {
margin-top: 30px;
width: 250px;
height: 250px;
background: yellow;
}
Conclusion
Together we’ve examined an end-to-end example of showing and hiding components based on conditions. The concept is quite simple, but from here, you can go much further and build huge and complex things. If you’d like to explore more new and fascinating stuff in the modern React world, then take a look at the following articles:
- Best Libraries for Formatting Date and Time in React
- React + TypeScript: Working with Props and Types of Props
- 2 Ways to Use Custom Port in React (create-react-app)
- React + TypeScript: Re-render a Component on Window Resize
- React + TypeScript: Image onLoad & onError Events
- React: Create an Animated Side Navigation from Scratch
You can also check our React category page and React Native category page for the latest tutorials and examples.