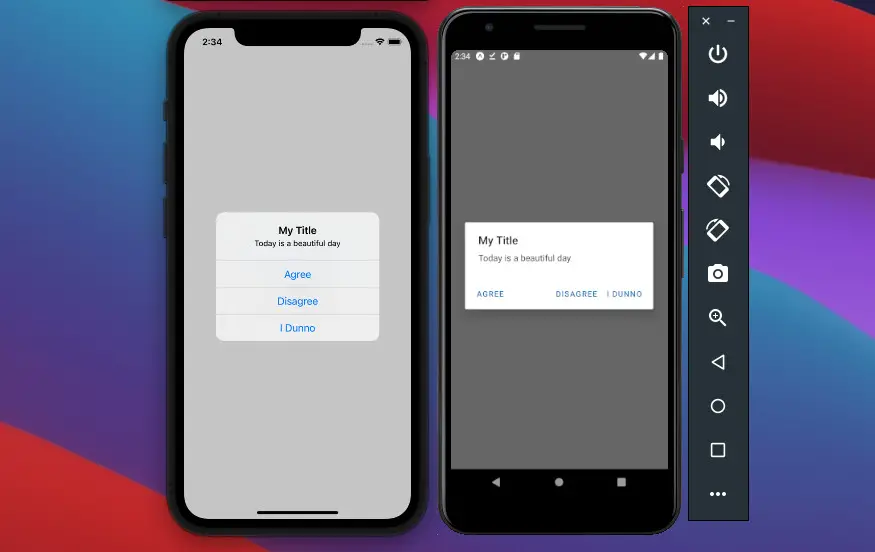
Alert is a built-in component in React Native that can launch a dialog with a title, message, and buttons. The example below will show you how to use it in practice.
Example
Preview
The sample app we are going to build has a button in the center of the screen. When the user presses this button, an alert dialog with some options will show up. The selected option will be displayed on the screen within a Text component (the alert dialog will disappear right after the decision is made).
Here’s how it work:
The Code
Create a new React Native project with React Native CLI or Expo, then replace the default code in App.js with the following:
import { useState } from "react";
import { StyleSheet, View, Alert, Button, Text } from "react-native";
const App = () => {
// this function will be called when the button is pressed
const showAlert = () => {
return Alert.alert(
// the title of the alert dialog
"My Title",
// the message you want to show up
"Today is a beautiful day",
// buttons
// On iOS there is no limit of how many buttons you can use, but on Android three is the maximum
// If you're developing an app for both platforms, don't use more than three buttons
[
// the first button
{
text: "Agree",
onPress: () => setChoice("Agree"),
},
// the second button
{
text: "Disagree",
onPress: () => setChoice("Disagree"),
},
// the third button
{
text: "I Dunno",
onPress: () => setChoice("I do not know"),
},
]
);
};
const [choice, setChoice] = useState("");
return (
<View style={styles.screen}>
<Button title="Show Alert Dialog" onPress={showAlert} />
<Text style={styles.text}>{choice}</Text>
</View>
);
};
// just some styles for our app
const styles = StyleSheet.create({
screen: {
flex: 1,
flexDirection: "column",
justifyContent: "center",
alignItems: "center",
},
text: {
marginTop: 30,
fontSize: 24,
},
});
export default App;
You can find more details about available props in the official docs (reactnative.dev).
Further reading:
- Using Switch component in React Native (with Hooks)
- How to Get the Window Width & Height in React Native
- React Native: Make a Button with a Loading Indicator inside
- Using AsyncStorage in React Native: CRUD Example
- How to create circle images in React Native
You can also check our React topic page and React Native topic page for the latest tutorials and examples.