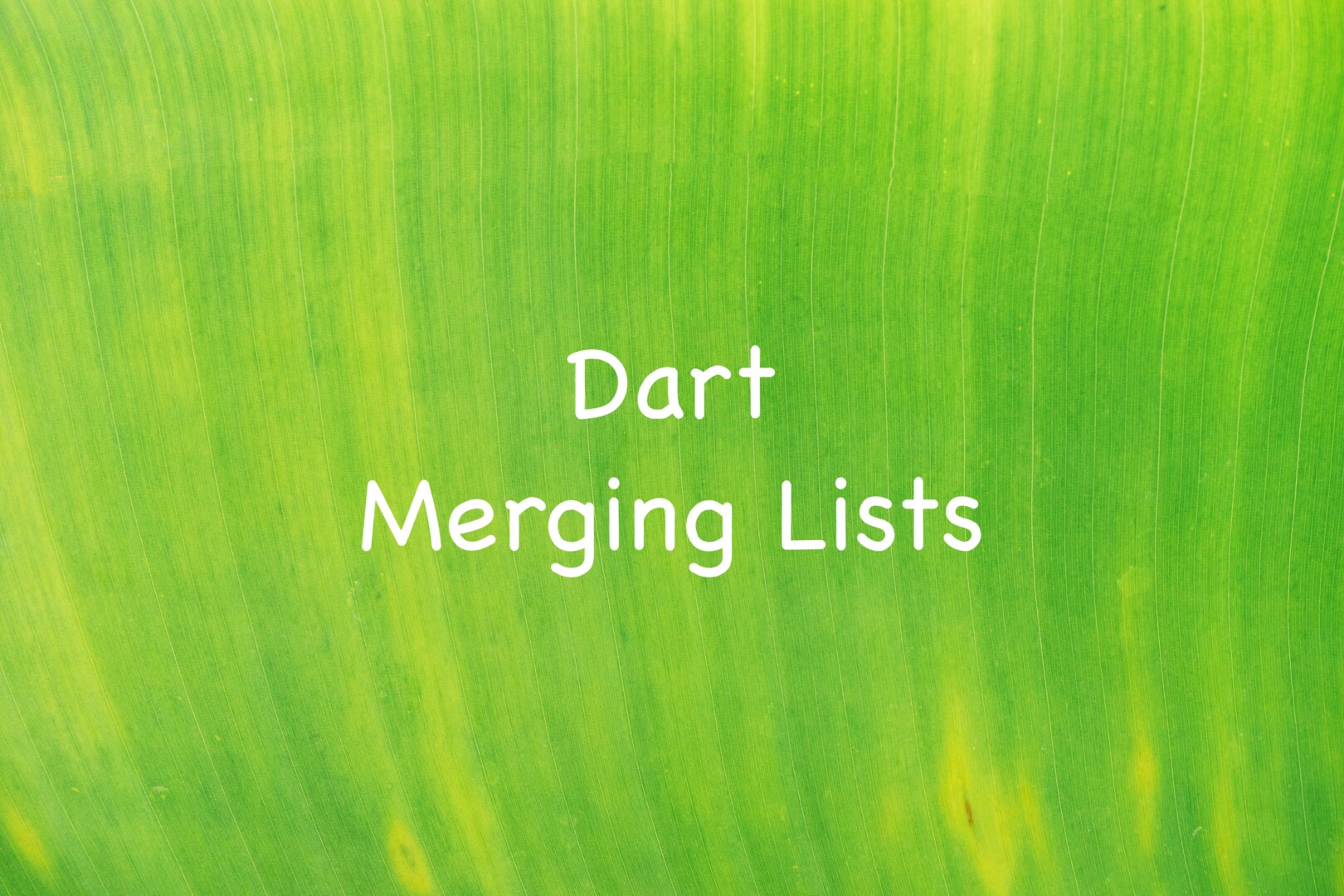
This article shows you a couple of different ways to join 2 lists in Dart. You will learn each method through an example.
Using Addition (+) Operator
Example:
void main() {
final List list1 = [1, 2, 3];
final List list2 = [4, 5, 6];
final List list3 = list1 + list2;
print(list3);
}
Output:
[1, 2, 3, 4, 5, 6]
In my opinion, this approach is the most intuitive.
Using List addAll() method
The List class of Dart provides the addAll() method that helps you easily join 2 lists together.
Example:
void main() {
List listA = [1, 2, 3];
List listB = [4, 5, 6];
listA.addAll(listB);
print(listA);
List<String> listC = ['Dog', 'Cat'];
List<String> listD = ['Bear', 'Tiger'];
listC.addAll(listD);
print(listC);
}
Output:
[1, 2, 3, 4, 5, 6]
[Dog, Cat, Bear, Tiger]
Using Spread Operator
This approach is very concise.
Example:
void main() {
final List list1 = ['A', 'B', 'C'];
final List list2 = ['D', 'E', 'F'];
final List list3 = [...list1, ...list2];
print(list3);
}
Output:
[A, B, C, D, E, F]
Wrapping Up
We’ve walked through a few approaches to combining lists in Dart. This knowledge is very helpful when working with data-driven applications. If you’d like to explore more about Dart and Flutter, take a look at the following articles:
- Dart: Convert Class Instances (Objects) to Maps and Vice Versa
- How to Flatten a Nested List in Dart
- Flutter & Dart: 3 Ways to Generate Random Strings
- Using Chip widget in Flutter: Tutorial & Examples
- Flutter: SliverGrid example
- Flutter & Dart: How to Check if a String is Null/Empty
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.