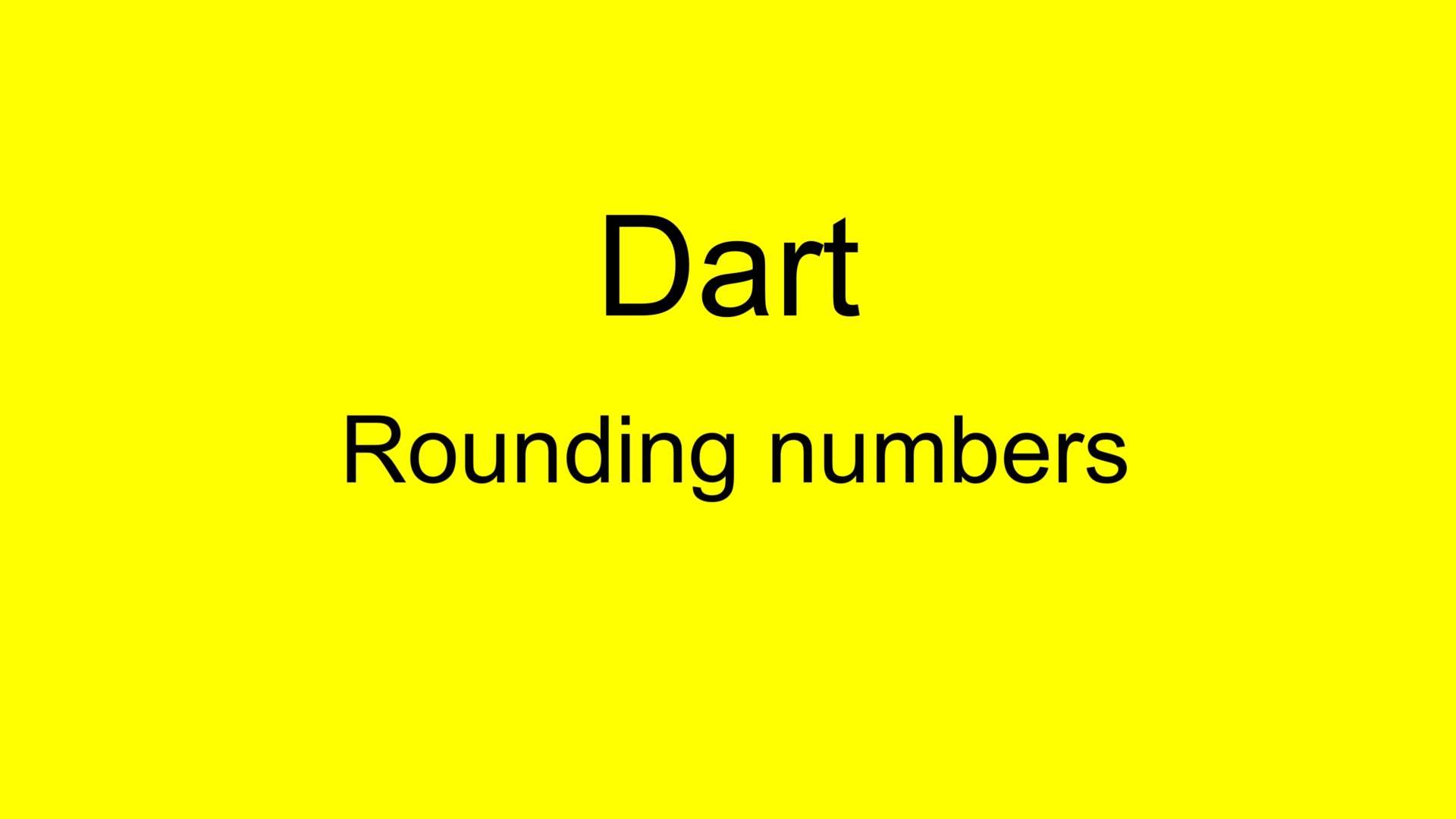
In order to round a float number to an integer in Dart, we can use the round() method. This method returns the closest integer to the input number. If cannot determine the closest integer (e.g. 0.5, -2.5, 3.5, etc), rounds away from zero.
Example:
import 'package:flutter/foundation.dart';
void main() {
var x = 10.3333;
if (kDebugMode) {
print(x.round());
}
var y = -1.45;
if (kDebugMode) {
print(y.round());
}
var z = 4.55;
if (kDebugMode) {
print(z.round());
}
var t = 1.5;
if (kDebugMode) {
print(t.round());
}
}
Output:
10
-1
5
2
If you provide an input number that is not finite (NaN or an infinity), the round() method will throw an UnsupportedError exception.
Further reading:
- Dart: Convert Class Instances (Objects) to Maps and Vice Versa
- How to Flatten a Nested List in Dart
- Inheritance in Dart: A Quick Example
- Flutter & Dart: 3 Ways to Generate Random Strings
- Flutter & Dart: Displaying Large Numbers with Digit Grouping
- How to Reverse a String in Dart (3 Approaches)
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.