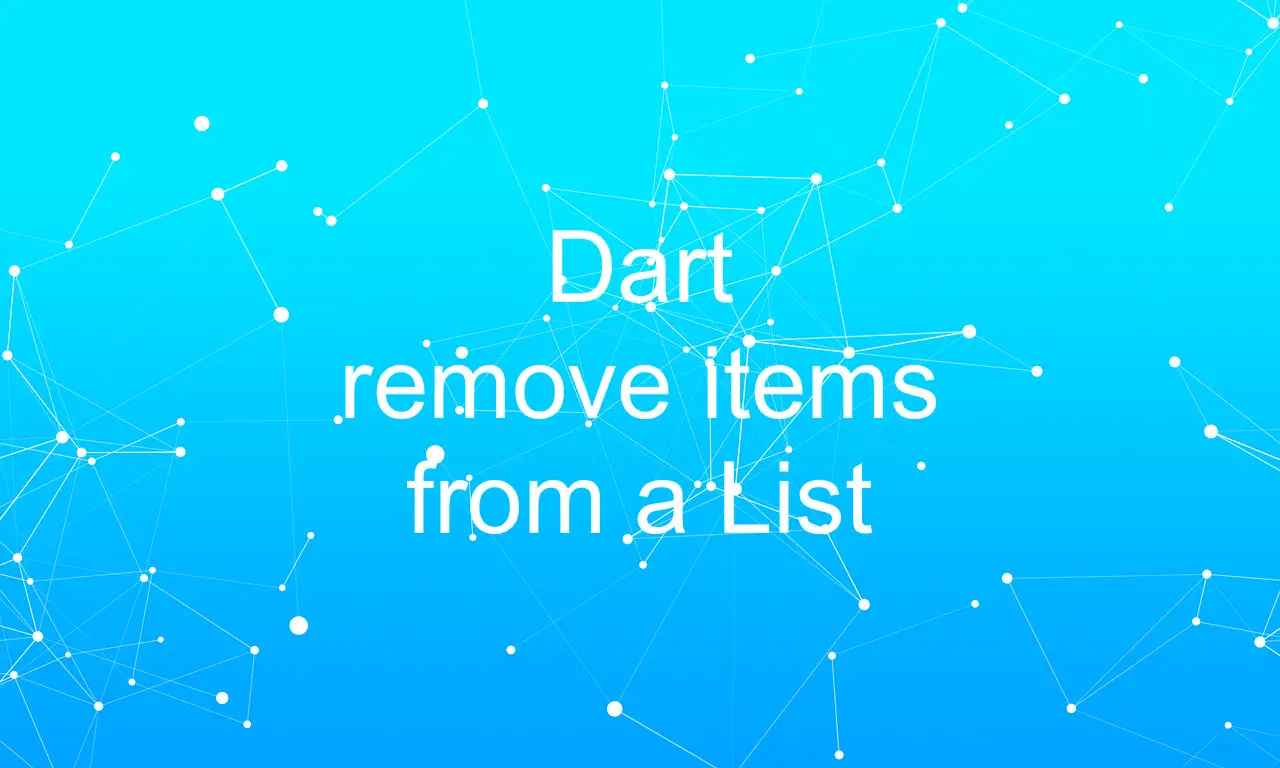
One of the most common tasks you encounter while working with a list in Dart is removing its items. Similar to other popular programming languages, Dart has some built-in list methods that help us get this matter done quickly.
List.remove()
This method is used to remove the first occurrence of a value from a list. It returns true if that value was in the list and returns false otherwise.
Example:
final myList = ['a', 'b', 'c', 'd', 'e', 'f', 'd', 'b'];
void main(){
myList.remove('b');
print(myList);
}
Output:
[a, c, d, e, f, d, b]
List.removeWhere()
This method is used to remove all items from a list that satisfy one or many conditions.
Example
This example removes people who are under 18 from a given list:
final List<Map<String, dynamic>> people = [
{'name': 'John', 'age': 30},
{'name': 'Bob', 'age': 50},
{'name': 'Tom', 'age': 14},
{'name': 'Donald', 'age': 3}
];
void main() {
people.removeWhere((person) => person['age'] <= 18);
print(people);
}
Output:
[{name: John, age: 30}, {name: Bob, age: 50}]
List.removeAt()
This method is used to remove an item at the position of an index from a list.
Example
This program removes the third person from a list of people (the index of the first person is 0):
final List<Map<String, dynamic>> people = [
{'name': 'John', 'age': 30},
{'name': 'Bob', 'age': 50},
{'name': 'Tom', 'age': 14},
{'name': 'Donald', 'age': 3}
];
void main() {
people.removeAt(2);
print(people);
}
Output:
[{name: John, age: 30}, {name: Bob, age: 50}, {name: Donald, age: 3}]
List.removeLast()
This method pops and returns the last item of a list.
Example
This program removes the last person from the list:
final List<Map<String, dynamic>> people = [
{'name': 'John', 'age': 30},
{'name': 'Bob', 'age': 50},
{'name': 'Tom', 'age': 14},
{'name': 'Donald', 'age': 3}
];
void main() {
people.removeLast();
print(people);
}
Output:
[{name: John, age: 30}, {name: Bob, age: 50}, {name: Tom, age: 14}]
List.removeRange()
This method removes the items in the range start inclusive to end exclusive:
List.removeRange(int start, int end)
Example
This program removes elements whose indices are from 2 to 5:
final myList = ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h'];
void main(){
myList.removeRange(2, 6);
print(myList);
}
Output:
[a, b, g, h]
Conclusion
Congratulations! At this point, you have learned 5 different methods to remove items from a list in Dart and can begin using them to build complicated Flutter projects.
You can also read about swiping to remove items from a ListView – highlighting selected items in a ListView – implementing horizontal ListView – Flutter: ListView Pagination (Load More) example to get a better sense of the list and ListView in Flutter.
In order to see the latest tutorials and examples, take a look at our Flutter topic page and Dart topic page. Happy coding.