This succinct article shows you how to parse and display XML data in Flutter.
Table of Contents
Overview
Like JSON, XML can be used to receive data from a web server. However, JSON is a data interchange format and only provides a data encoding specification, while XML is a language that uses strict semantics to specify custom markup languages and provides a lot more than data interchange.
In general, JSON is faster and easier to use. Nevertheless, XML is more powerful and can reduce software risk in large applications with puritanical data structure requirements.
To use XML in Flutter, we need to work through these steps:
- Fetch an XML document
- Use the XML DOM to loop through the document
- Extract values and store them in variables
A package named xml can help us save time and energy when dealing with XML data. Let’s build a complete sample app to get a better understanding.
Example
What are We Going To Build?
This demo renders a list of employees in a fictional company, including their names and salaries. The information is provided in XML format (in real life, this kind of data is usually fetched from an API or from a file):
<?xml version="1.0"?>
<employees>
<employee>
<name>Spiderman</name>
<salary>5000</salary>
</employee>
<employee>
<name>Dr. Strange</name>
<salary>6000</salary>
</employee>
<employee>
<name>Thanos</name>
<salary>7000</salary>
</employee>
<employee>
<name>Iron Man</name>
<salary>8000</salary>
</employee>
<employee>
<name>KindaCode.com</name>
<salary>0.5</salary>
</employee>
</employees>
App screenshot:
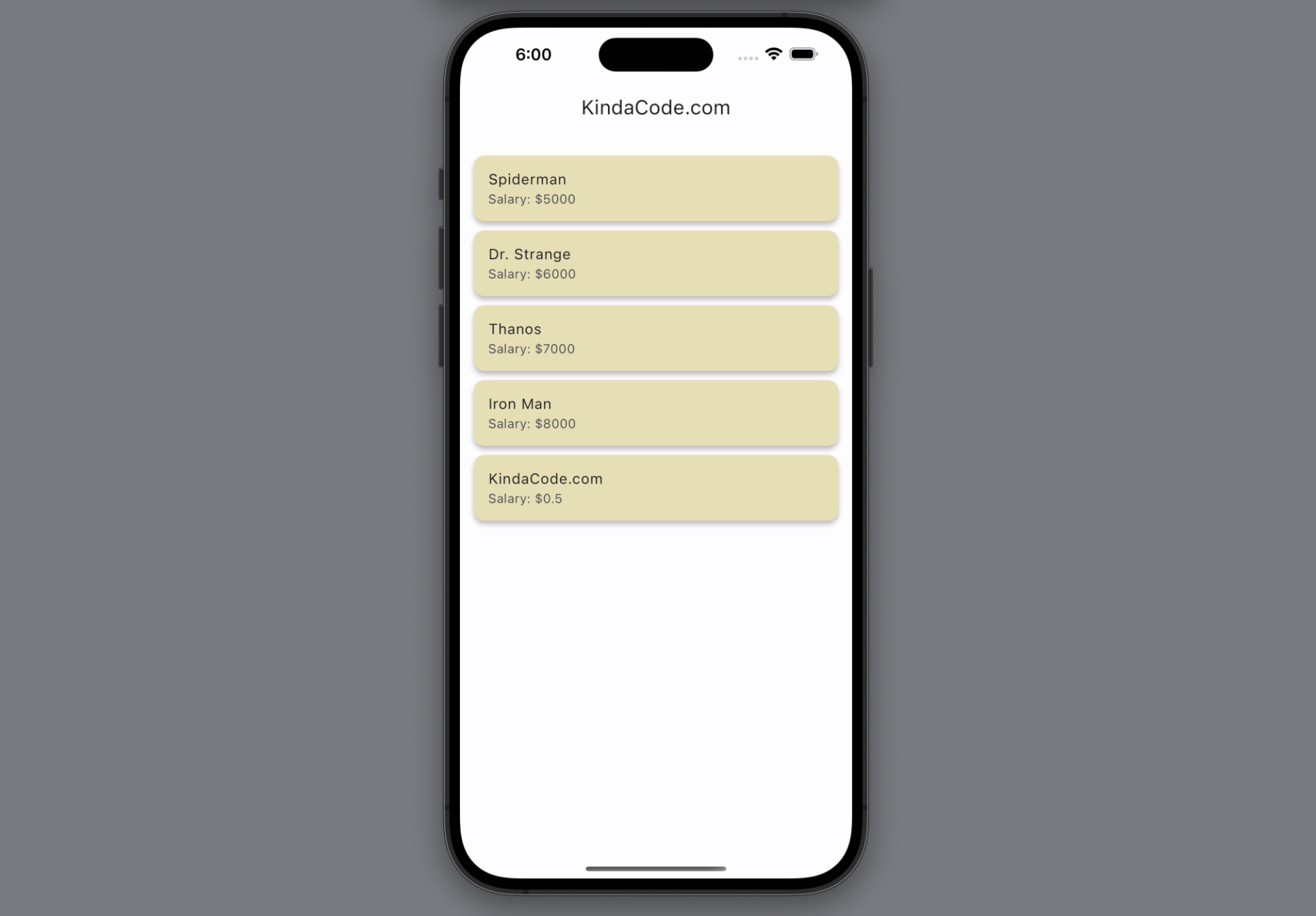
The Code
1. Install the package by running the command below:
flutter pub add xml
Then execute this:
flutter pub get
2. The full source code in main.dart
(with explanations):
// main.dart
import 'package:flutter/material.dart';
import 'package:xml/xml.dart' as xml;
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'KindaCode.com',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.blue),
useMaterial3: true),
home: const HomeScreen(),
);
}
}
class HomeScreen extends StatefulWidget {
const HomeScreen({Key? key}) : super(key: key);
@override
State<HomeScreen> createState() => _HomeScreenState();
}
class _HomeScreenState extends State<HomeScreen> {
// This list will be displayed in the ListView
List _employees = [];
// This function will be triggered when the app starts
void _loadData() async {
final temporaryList = [];
// in real life, this is usually fetched from an API or from an XML file
// In this example, we use this XML document to simulate the API response
const employeeXml = '''<?xml version="1.0"?>
<employees>
<employee>
<name>Spiderman</name>
<salary>5000</salary>
</employee>
<employee>
<name>Dr. Strange</name>
<salary>6000</salary>
</employee>
<employee>
<name>Thanos</name>
<salary>7000</salary>
</employee>
<employee>
<name>Iron Man</name>
<salary>8000</salary>
</employee>
<employee>
<name>KindaCode.com</name>
<salary>0.5</salary>
</employee>
</employees>''';
// Parse XML data
final document = xml.XmlDocument.parse(employeeXml);
final employeesNode = document.findElements('employees').first;
final employees = employeesNode.findElements('employee');
// loop through the document and extract values
for (final employee in employees) {
final name = employee.findElements('name').first.text;
final salary = employee.findElements('salary').first.text;
temporaryList.add({'name': name, 'salary': salary});
}
// Update the UI
setState(() {
_employees = temporaryList;
});
}
// Call the _loadData() function when the app starts
@override
void initState() {
super.initState();
_loadData();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('KindaCode.com')),
body: Padding(
padding: const EdgeInsets.symmetric(vertical: 20),
// list of employees
child: ListView.builder(
itemBuilder: (context, index) => Card(
key: ValueKey(_employees[index]['name']),
margin: const EdgeInsets.symmetric(vertical: 5, horizontal: 15),
color: Colors.amber.shade100,
elevation: 4,
child: ListTile(
title: Text(_employees[index]['name']),
subtitle: Text("Salary: \$${_employees[index]['salary']}"),
),
),
itemCount: _employees.length,
),
),
);
}
}
Final Words
You’ve learned how to parse and extract values from an XML document to present in a list view. You can modify the code to improve the app if you like.
Keep your motivation and continue exploring more new and interesting stuff in the world of Flutter by taking a look at the following articles:
- Flutter: Full-Screen Semi-Transparent Modal Dialog
- Flutter: Load and display content from CSV files
- Flutter: How to Read and Write Text Files
- How to encode/decode JSON in Flutter
- How to read data from local JSON files in Flutter
- 2 Ways to Fetch Data from APIs in Flutter
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.