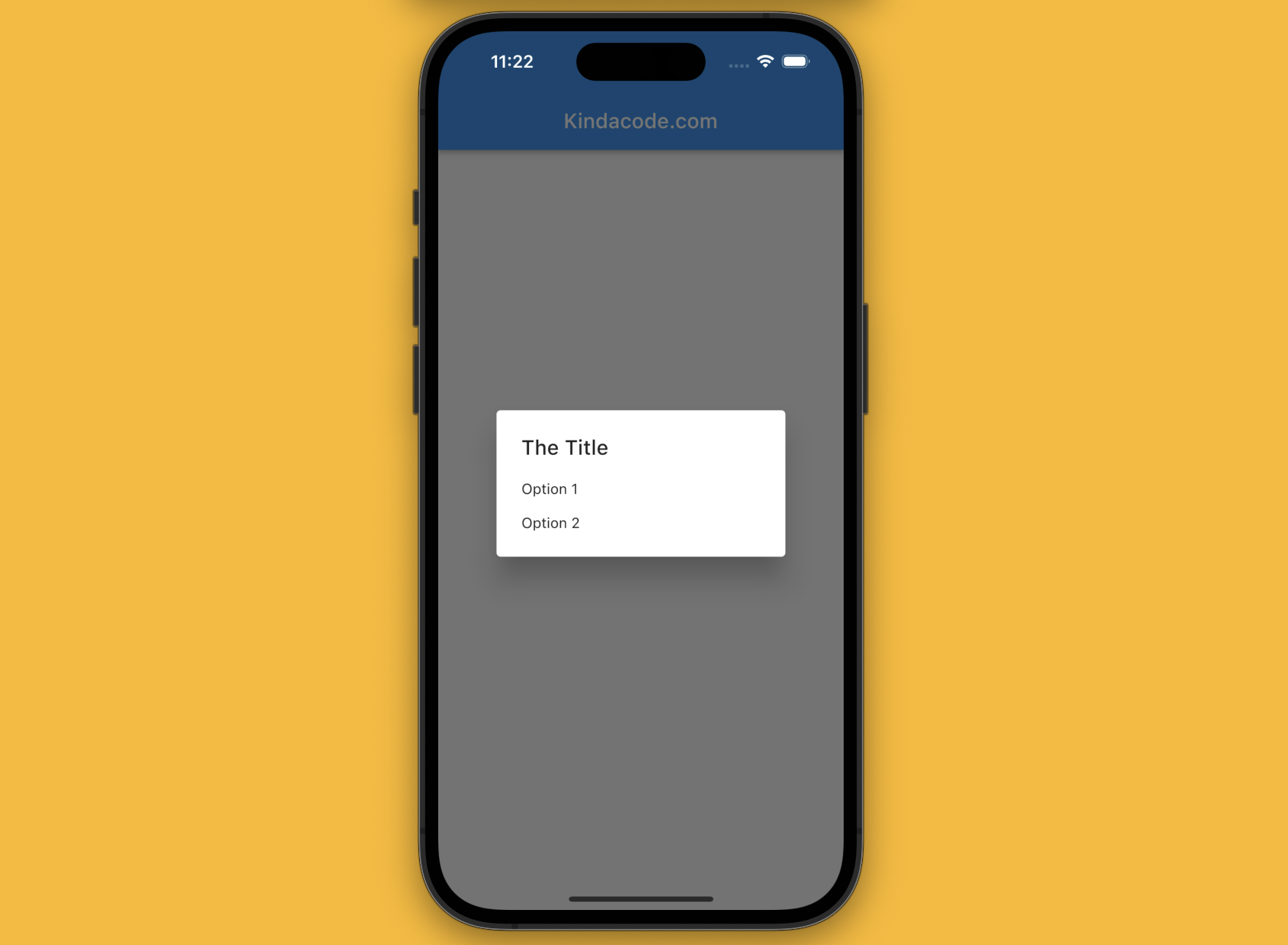
Table of Contents
Summary
This is a short and straight-to-the-point guide to the SimpleDialog widget (that renders a basic material dialog with some options) in Flutter.
In general, the SimpleDialog widget isn’t often used directly. Instead, it’s shown by using the showDialog function:
showDialog(context: ctx, builder: (_) {
return SimpleDialog(
title: const Text('The Title'),
children: [
SimpleDialogOption(
child: ...
onPressed: ...
),
],
);
},);
To close the dialog, just use this:
Navigator.of(context).pop()
Example
The small app we are going to build contains a text button in the center of the screen. When the user presses the button, a simple dialog will show up with two options: Option 1 and Option 2. When an option is selected, some text will be printed out in the terminal window (you can do anything else you like).
App preview
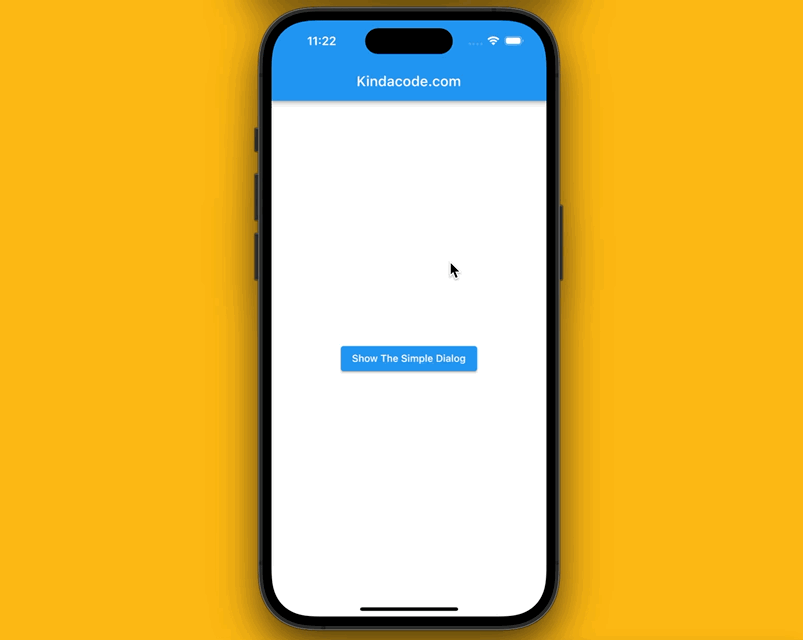
The complete code
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Hide the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
theme: ThemeData.light(),
home: const HomePage(),
);
}
}
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
// The point of this guide is here
void _show(BuildContext ctx) {
showDialog(
context: ctx,
builder: (_) {
return SimpleDialog(
title: const Text('The Title'),
children: [
SimpleDialogOption(
child: const Text('Option 1'),
onPressed: () {
// Do something
debugPrint('You have selected the option 1');
Navigator.of(ctx).pop();
},
),
SimpleDialogOption(
child: const Text('Option 2'),
onPressed: () {
// Do something
debugPrint('You have selected the option 2');
Navigator.of(ctx).pop();
},
)
],
);
},
);
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Center(
child: ElevatedButton(
child: const Text('Show The Simple Dialog'),
onPressed: () => _show(context),
),
),
);
}
}
Hope this helps. Further reading:
- How to Make a Confirm Dialog in Flutter
- Flutter: Bottom Sheet examples
- 2 Ways to Create Flipping Card Animation in Flutter
- Flutter: How to Make Spinning Animation without Plugins
- Flutter: How to Draw a Heart with CustomPaint
- Using GetX (Get) for Navigation and Routing in Flutter
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.