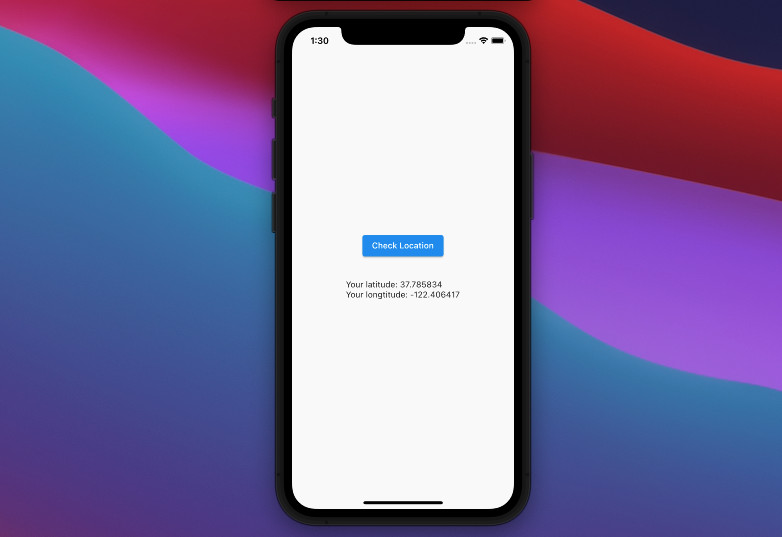
This is a short guide to getting the user’s current location in Flutter by using a plugin called location.
Installation
1. Add location and its latest version to the dependencies section in your pubspec.yaml file by running the command below:
flutter pub add location
2. Perform the following to make sure the package was pulled to your project:
flutter pub get
3. Import the plugin into your Dart code:
import 'package:location/location.dart';
We’re not done and have to add some special setup.
iOS permission setup
Add the following to the dict section in your <project-root>/ios/Runner/Info.plist:
<key>NSLocationWhenInUseUsageDescription</key>
<string>Some description</string>
Here’s mine:
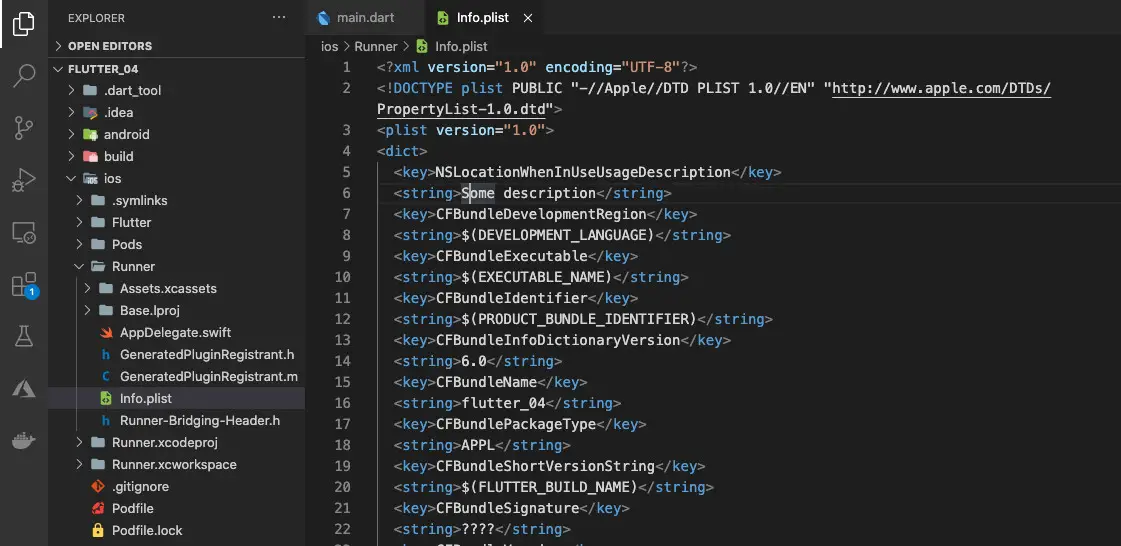
Android permission setup
No extra setup is required if you’re using Flutter 1.12 or beyond. However, if your app needs to access the location in background mode, you have to place the following permissions in your AndroidManifest.xml:
<uses-permission android:name="android.permission.FOREGROUND_SERVICE" />
<uses-permission android:name="android.permission.ACCESS_BACKGROUND_LOCATION"/>
The Complete Example
App Preview
This tiny sample app contains a button in the center of the screen. When this button gets pressed, the current longitude and latitude will be returned (for the first time use, a dialog asking permission will appear. If you refuse, this dialog will never show again unless you delete the app and reinstall it).
Here’s how it works on Android (it takes a few seconds to fetch the latitude and the longitude):
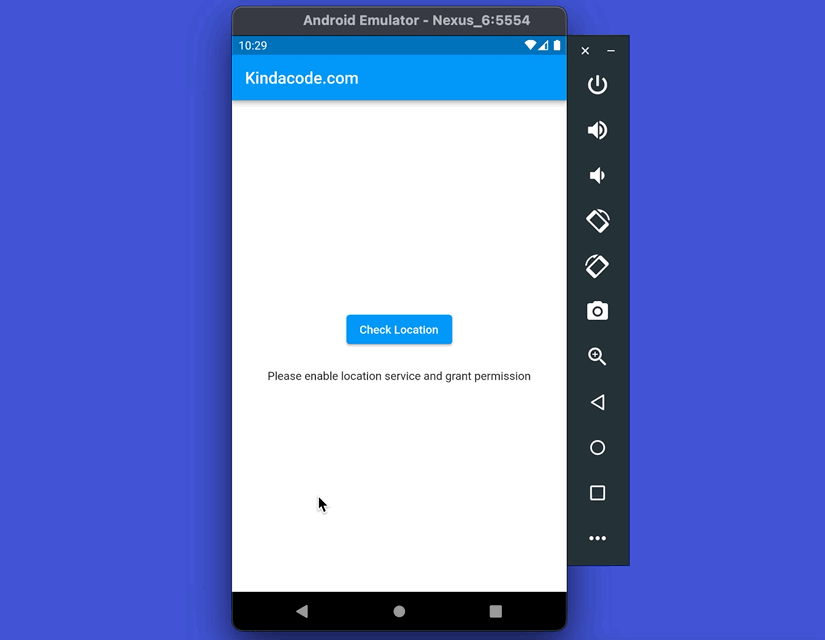
The Code
The full source code in main.dart with explanations:
// main.dart
import 'package:flutter/material.dart';
import 'package:location/location.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
/*
serviceEnabled and permissionGranted are used
to check if location service is enable and permission is granted
*/
late bool _serviceEnabled;
late PermissionStatus _permissionGranted;
LocationData? _userLocation;
// This function will get user location
Future<void> _getUserLocation() async {
Location location = Location();
// Check if location service is enable
_serviceEnabled = await location.serviceEnabled();
if (!_serviceEnabled) {
_serviceEnabled = await location.requestService();
if (!_serviceEnabled) {
return;
}
}
// Check if permission is granted
_permissionGranted = await location.hasPermission();
if (_permissionGranted == PermissionStatus.denied) {
_permissionGranted = await location.requestPermission();
if (_permissionGranted != PermissionStatus.granted) {
return;
}
}
final locationData = await location.getLocation();
setState(() {
_userLocation = locationData;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Center(
child: Padding(
padding: const EdgeInsets.all(20),
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
ElevatedButton(
onPressed: _getUserLocation,
child: const Text('Check Location')),
const SizedBox(height: 25),
// Display latitude & longtitude
_userLocation != null
? Wrap(
children: [
Text('Your latitude: ${_userLocation?.latitude}'),
const SizedBox(width: 10),
Text('Your longtitude: ${_userLocation?.longitude}')
],
)
: const Text(
'Please enable location service and grant permission')
],
),
),
),
);
}
}
Conclusion
You’ve learned how to retrieve the longitude and latitude of the current user. If you’d like to explore more amazing and new things about modern mobile development with Flutter, take a look at the following articles:
- Flutter: SliverGrid example
- Flutter and Firestore Database: CRUD example
- Flutter: Firebase Remote Config example
- Flutter: Convert UTC Time to Local Time and Vice Versa
- How to implement Star Rating in Flutter
- Flutter: Creating a Custom Number Stepper Input
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.