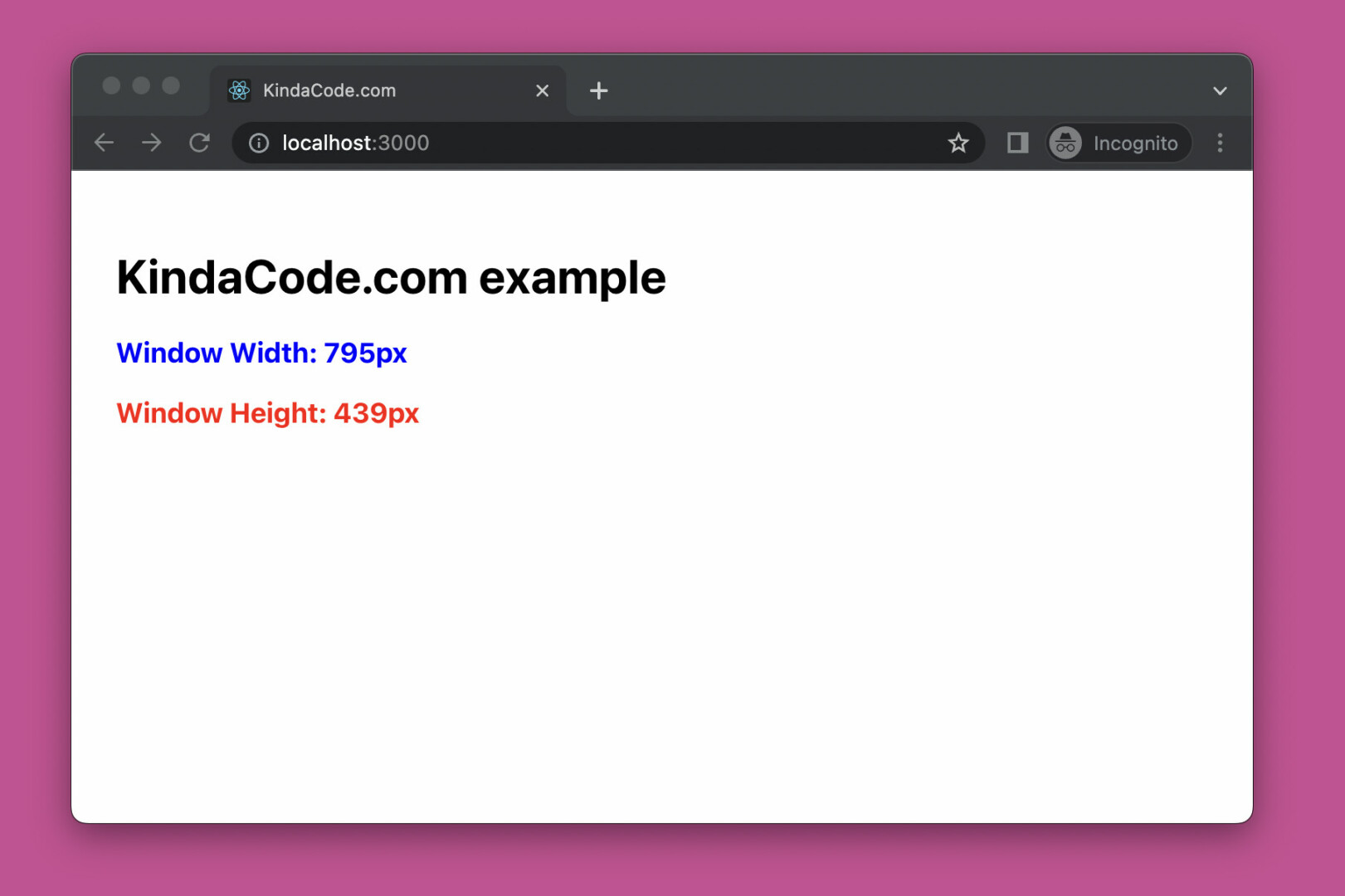
This concise and straightforward article shows you how to get the width and height of the viewport (window) in React. Without any further ado, let’s unveil things that matter.
Table of Contents
Overview
In React and any other frontend technologies that use Javascript, you can use the window object without importing any things. This object provides 2 properties that can help us read the width and height of the viewport:
- window.innerWidth
- window.innerHeight
However, the size of the window is not consistent. The complete example below demonstrates how to update the width and height we receive each time the browser window is resized by the user.
Full Example
Preview
The idea of this demo project is really simple. We just get the width and height of the viewport and then display them on the screen.
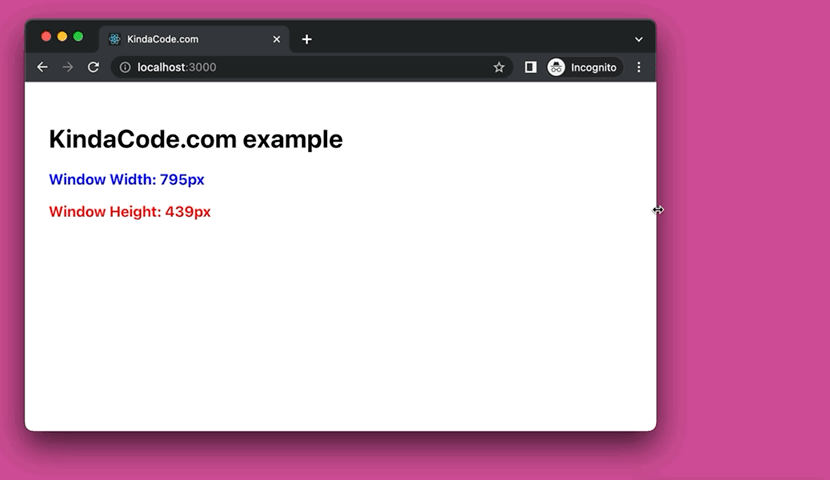
The Code
Here’s the full source code in the App.js file with explanations:
// KindaCode.com
// src/App.js
import { useState, useEffect } from 'react';
function App() {
// the size of the window in the begginning
const [windowSize, setWindowSize] = useState({
width: window.innerWidth,
height: window.innerHeight,
});
// we use the useEffect hook to listen to the window resize event
useEffect(() => {
window.onresize = () => {
setWindowSize({
width: window.innerWidth,
height: window.innerHeight,
});
};
}, []);
return (
<div
style={{
padding: 30,
}}
>
<h1>KindaCode.com example</h1>
{/* Display the results */}
<h3 style={{ color: 'blue' }}>Window Width: {windowSize.width}px</h3>
<h3 style={{ color: 'red' }}>Window Height: {windowSize.height}px</h3>
</div>
);
}
export default App;
Conclusion
You’ve learned how to obtain information about the size of the window. This knowledge is very helpful, especially when you develop a responsive and adaptive web app. If you’d like to explore more new and exciting stuff about modern React, take a look at the following articles:
- React: Show a Loading Dialog (without 3rd libraries)
- React Router: useParams & useSearchParams Hooks
- React: Get the Position (X & Y) of an Element
- How to Use Styled JSX in React: A Deep Dive
- How to Use Tailwind CSS in React
- React: How to Create a Reorderable List from Scratch
You can also check our React category page and React Native category page for the latest tutorials and examples.