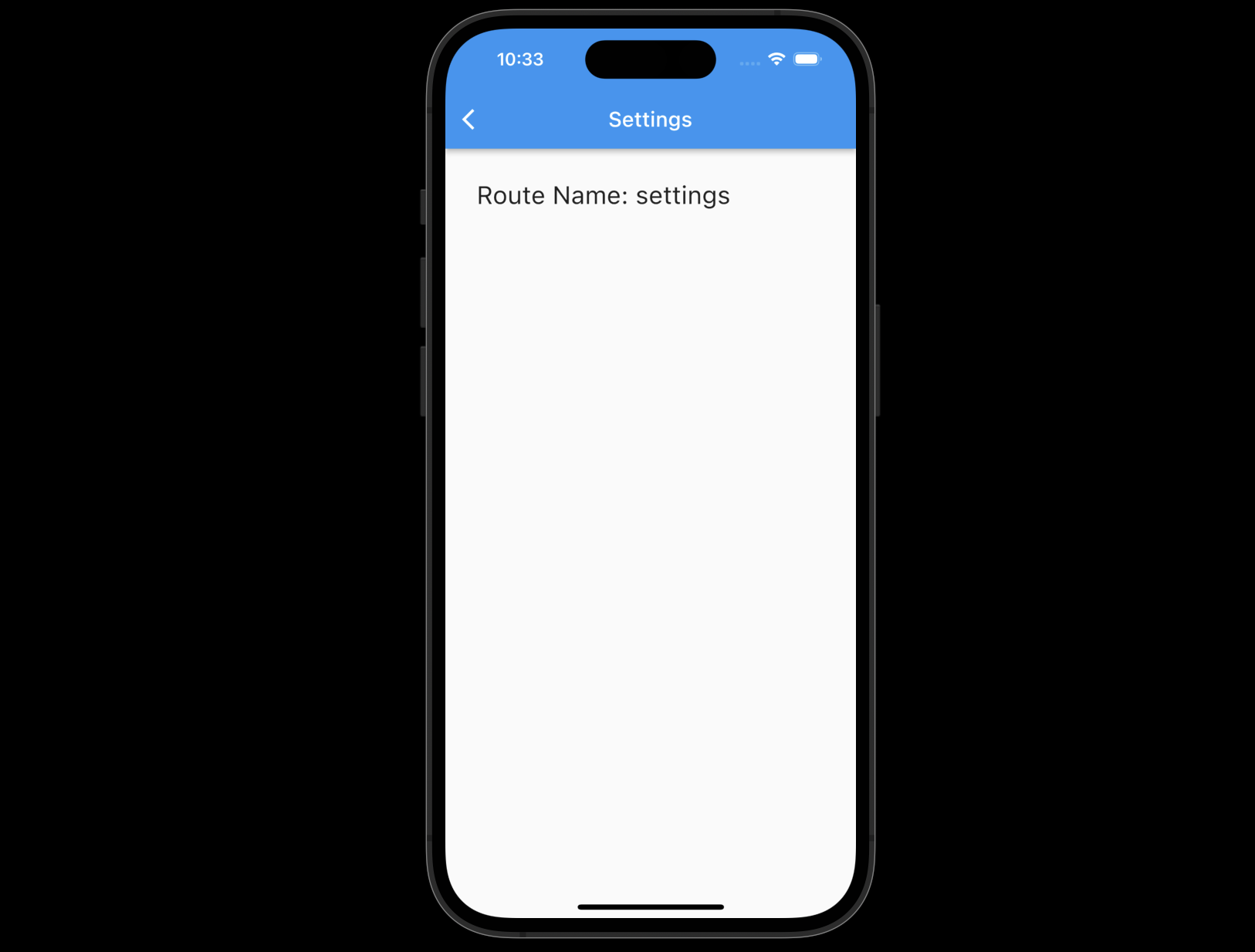
Table of Contents
Overview
If you register the routes in your Flutter app like this:
return MaterialApp(
home: HomePage(),
routes: {
'page-a': (BuildContext ctx) => PageA(),
'page-b': (BuildContext ctx) => PageB(),
'page-c': (BuildContext ctx) => PageC(),
},
)
Then you can retrieve the route name of the current page that is being seen by using this:
ModalRoute.of(context).settings.name
Notes:
- If you navigate to other pages by using pushNamed(), pushNamedAndRemoveUntil(), or pushReplacementNamed(), you’ll get the exact declared route names.
- If you use push(), pushAndRemoveUntil(), or pushReplacement() for navigating to other pages, the results will be null.
If you get confused, see the complete example below for more clarity.
Example
Preview
The tiny app we’re going to make has 2 screens: Home and Settings. On each screen, we’re going to display the corresponding route name.
Here’s how it works:
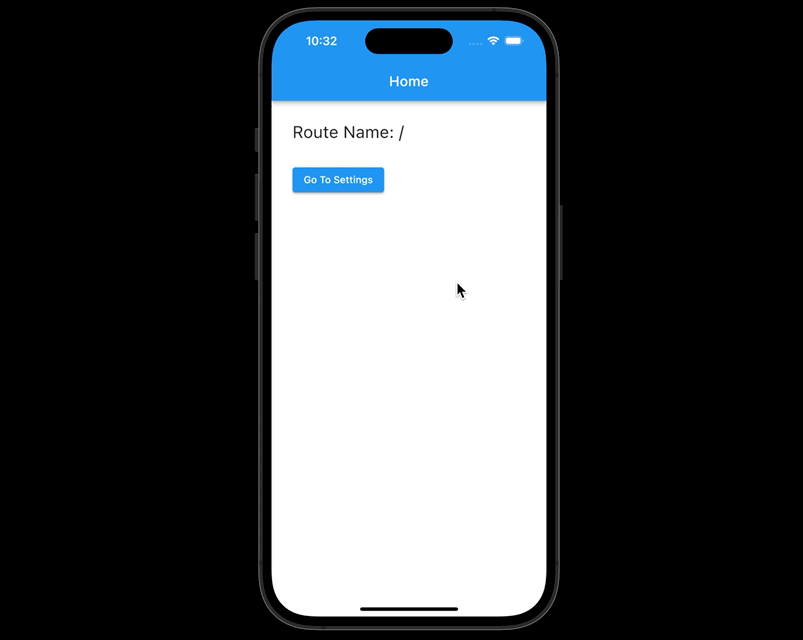
The full code
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// hide the debug banner
debugShowCheckedModeBanner: false,
title: "Kindacode.com",
home: const HomePage(),
routes: {
'settings': (BuildContext ctx) => const SettingsPage(),
},
);
}
}
// Home Page
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Home'),
),
body: Padding(
padding: const EdgeInsets.all(30),
child: Column(children: [
Text("Route Name: ${ModalRoute.of(context)?.settings.name}"),
const SizedBox(
height: 30,
),
TextButton(
onPressed: () {
Navigator.of(context).pushNamed('settings');
},
child: const Text('Go To Settings'))
]),
),
);
}
}
// Settings Page
class SettingsPage extends StatelessWidget {
const SettingsPage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Settings'),
),
body: Center(
child: Text("Route Name: ${ModalRoute.of(context)?.settings.name}"),
),
);
}
}
For simplicity’s sake, I put everything in a single Dart file. If you want to follow good practice and keep your code organized, you can put each class in a separate file.
Futher reading:
- Using GetX (Get) for Navigation and Routing in Flutter
- How to Create a Sortable ListView in Flutter
- Working with Cupertino Bottom Tab Bar in Flutter
- Flutter: BottomNavigationBar example
- Ways to Format DateTime in Flutter
- Flutter and Firestore Database: CRUD example
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.