In Flutter, a button is disabled if both its onPressed and onLongPress callbacks are null. This concise article shows you how to disable a button after it has been pressed (this will be useful in cases where you want the user to be able to interact with a button only once).
Overview
The steps:
1. Create a variable called _isPressed to hold the button condition. In the beginning, _isPressed = false (make sure you’re using a stateful widget).
2. If _isPressed = false, set the button’s onPressed property to a callback function, otherwise, set onPressed to null:
onPressed: _isPressed == false ? _myCallback : null,
Because the onLongPress property is null by default, you don’t have to do anything with it in this case.
3. When the button is pressed, call:
setState(() {
_isPressed = true;
});
These steps can be hard to imagine for developers who haven’t worked with Flutter for long. Consider the following complete example for better understanding.
Example
App preview:
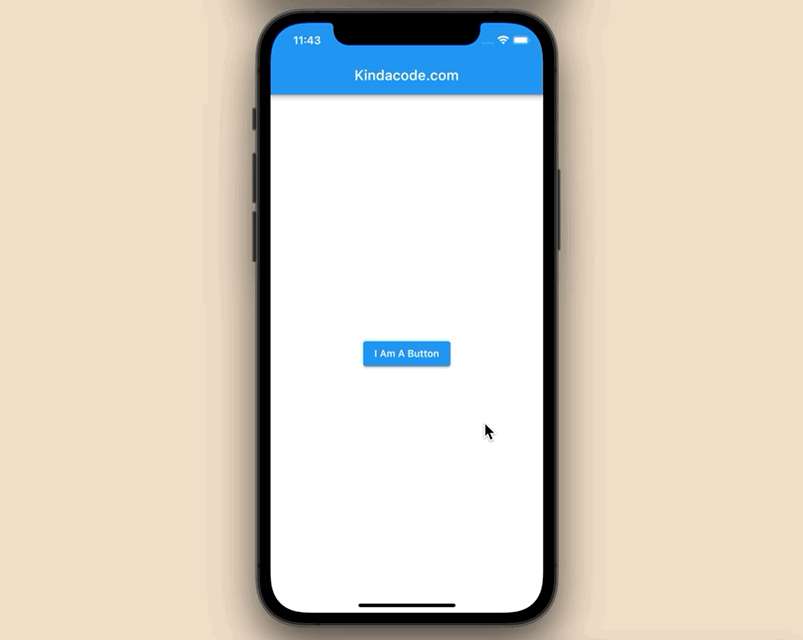
The code:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Hide the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
// This variable determines whether the button is disable or not
bool _isPressed = false;
// This function is called when the button gets pressed
void _myCallback() {
setState(() {
_isPressed = true;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Center(
child: ElevatedButton(
onPressed: _isPressed == false ? _myCallback : null,
child: const Text('I Am A Button'),
)));
}
}
That’s it. Further reading:
- Flutter: Creating Custom Back Buttons
- Working with ElevatedButton in Flutter
- Flutter: Text with Read More / Read Less Buttons
- Flutter: Create a Button with a Loading Indicator Inside
- Working with OutlinedButton in Flutter
- 4 Ways to Create Full-Width Buttons in Flutter
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.