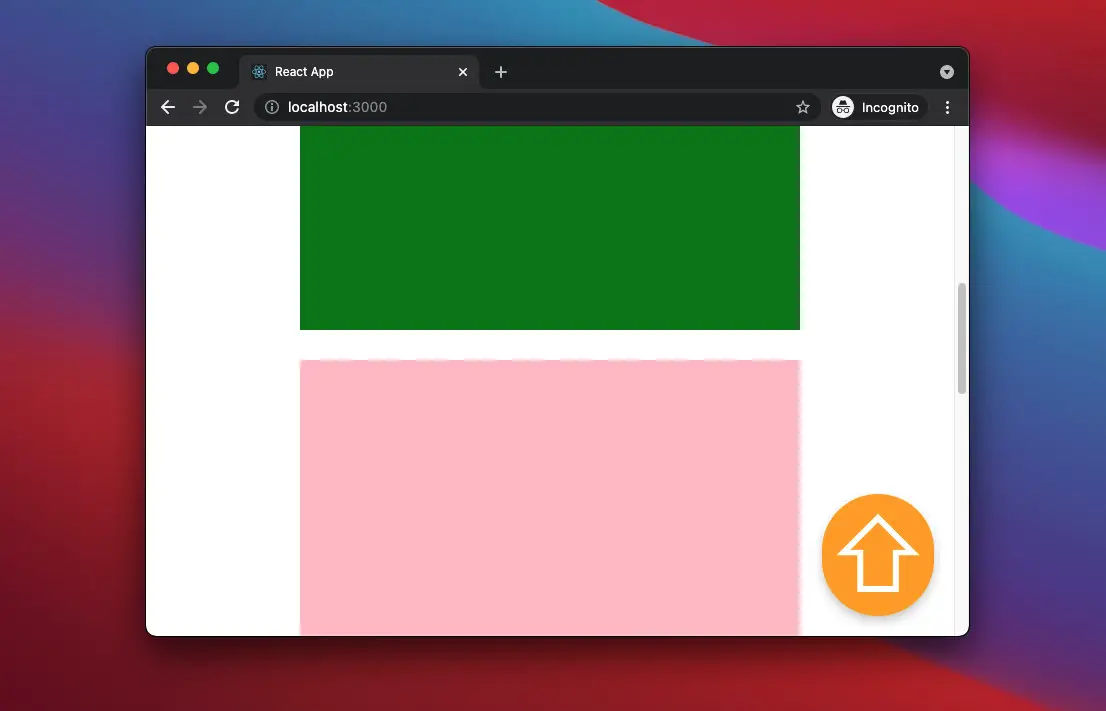
For long web pages with a lot of content, a back-to-top button will be very useful. This button will make it easy and convenient for the user to return to the top of the page once they have scrolled down some distance.
In this article, we are going to create a React back-to-top button from scratch without using any third-party libraries. What we use in the example below are the useState hook, useEffect hook, and the window object that is supported by all browsers that support Javascript.
Table of Contents
The Example
Preview
It doesn’t make lots of sense if the back-to-top button appears even when the user is at the top of the page. It should only show up when the user has scrolled down to a little bit.
A quick demo is worth more than a thousand words:
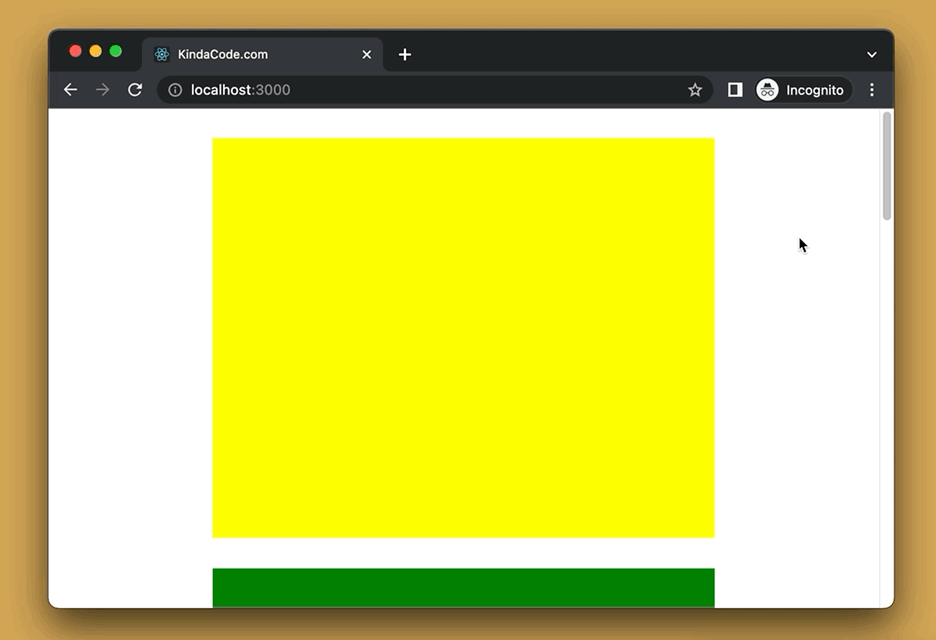
A quick note
To smoothly scroll the window to the top, we use:
window.scrollTo({
top: 0,
behavior: 'smooth' // for smoothly scrolling
});
The Code
1. Create a new React app and replace all of the default code in src/App.js with the following:
// App.js
// Kindacode.com
import { useEffect, useState } from "react";
import "./App.css";
const App = () => {
// The back-to-top button is hidden at the beginning
const [showButton, setShowButton] = useState(false);
useEffect(() => {
window.addEventListener("scroll", () => {
if (window.pageYOffset > 300) {
setShowButton(true);
} else {
setShowButton(false);
}
});
}, []);
// This function will scroll the window to the top
const scrollToTop = () => {
window.scrollTo({
top: 0,
behavior: 'smooth' // for smoothly scrolling
});
};
return (
<>
<div className="container">
<div className="box box--1"></div>
<div className="box box--2"></div>
<div className="box box--3"></div>
<div className="box box--4"></div>
<div className="box box--5"></div>
</div>
{showButton && (
<button onClick={scrollToTop} className="back-to-top">
⇧
</button>
)}
{/* ⇧ is used to create the upward arrow */}
</>
);
};
export default App;
2. Remove all of the unwanted code in App.css then add this:
.container {
width: 500px;
margin: auto;
text-align: center;
}
.back-to-top {
position: fixed;
bottom: 20px;
right: 20px;
font-size: 100px;
background: orange;
color: white;
cursor: pointer;
border-radius: 100px;
border: none;
box-shadow: 0 5px 10px #ccc;
}
.back-to-top:hover {
background: red;
}
/* Just some color boxes to make the page longer */
.box {
width: 100%;
height: 80vh;
margin: 30px 0;
}
.box--1 {
background: yellow;
}
.box--2 {
background: green;
}
.box--3 {
background: pink;
}
.box--4 {
background: grey;
}
.box--5 {
background: purple;
}
3. Run your project and check the result.
Conclusion
We’ve made a back-to-top button without using any third-party packages. From here, you can easily implement this useful button in other projects as needed. If you’d like to learn more new and interesting things about modern React, take a look at the following articles:
- React + TypeScript: Handling onFocus and onBlur events
- React & TypeScript: Using useRef hook example
- React + TypeScript: Using setTimeout() with Hooks
- Most popular React Component UI Libraries
- React: Create an Animated Side Navigation from Scratch
- Top 4 React form validation libraries
You can also check our React category page and React Native category page for the latest tutorials and examples.