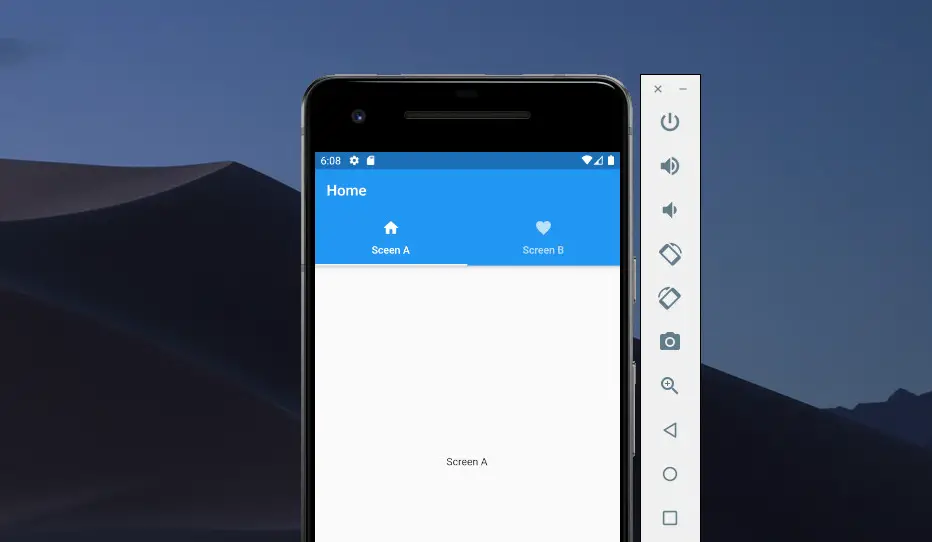
This short article shows you how to add a TabBar to the bottom area of an AppBar in Flutter with DefaultTabController, TabBar, and Tab.
Table of Contents
Example
Preview
We’ll make a small Flutter app that contains 2 screens: ScreenA and ScreenB. The user can use the TabBar, that locates at the bottom of the AppBar, to navigate between the screens.
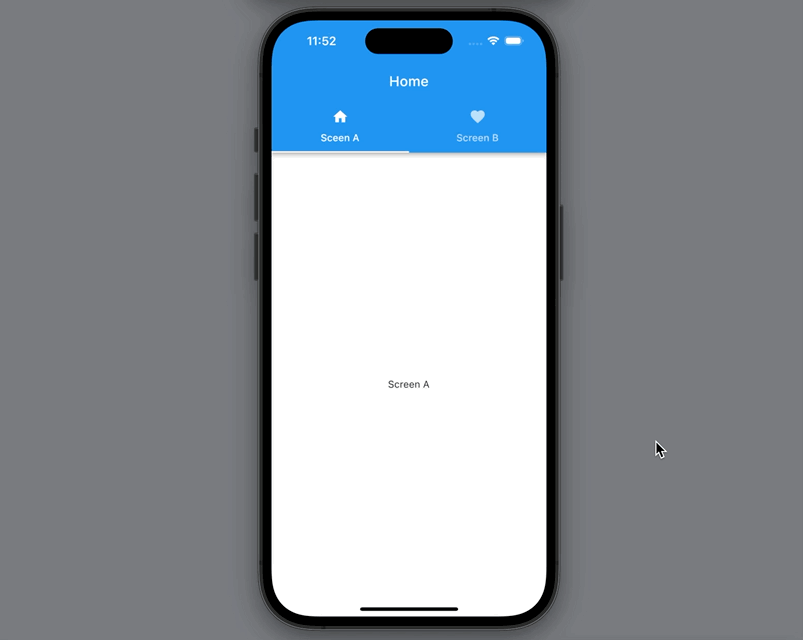
Writing Code
1. Create a new Flutter project:
flutter create my_app
2. In the lib folder, create 2 new files: screen_a.dart and screen_b.dart. Here’s the structure:
.
├── main.dart
├── screen_a.dart
└── screen_b.dart
3. Add the following to screen_a.dart to create ScreenA:
// screen_a.dart
import 'package:flutter/material.dart';
class ScreenA extends StatelessWidget {
const ScreenA({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const Center(child: Text('Screen A'));
}
}
4. Add the following to screen_b.dart to create ScreenB:
// screen_b.dart
import 'package:flutter/material.dart';
class ScreenB extends StatelessWidget {
const ScreenB({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const Center(child: Text('Screen B'));
}
}
5. Remove all the default code in main.dart and add this:
// main.dart
import 'package:flutter/material.dart';
import './screen_a.dart';
import './screen_b.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Hide the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
theme: ThemeData.light(),
home: const TabsScreen(),
);
}
}
// Focus this section
class TabsScreen extends StatelessWidget {
const TabsScreen({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return DefaultTabController(
length: 2,
child: Scaffold(
appBar: AppBar(
title: const Text('Home'),
bottom: const TabBar(
tabs: [
Tab(icon: Icon(Icons.home), text: 'Sceen A'),
Tab(icon: Icon(Icons.favorite), text: 'Screen B')
],
),
),
body: const TabBarView(
children: [ScreenA(), ScreenB()],
),
),
);
}
}
Hope this helps.
Conclusion
We’ve walked through an end-to-end example of implementing a tab bar to the bottom of an app bar. If you’d like to explore more new and exciting stuff about modern Flutter development, take a look at the following articles:
- Flutter: Global Styles for AppBar using AppBarTheme
- Flutter: 2 Ways to Create an Onboarding Flow (Intro Slider)
- Flutter SliverAppBar Example (with Explanations)
- Flutter: How to center the appBar title on Android
- Flutter: Hide the AppBar in landscape mode
- Creating Masonry Layout in Flutter with Staggered Grid View
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.