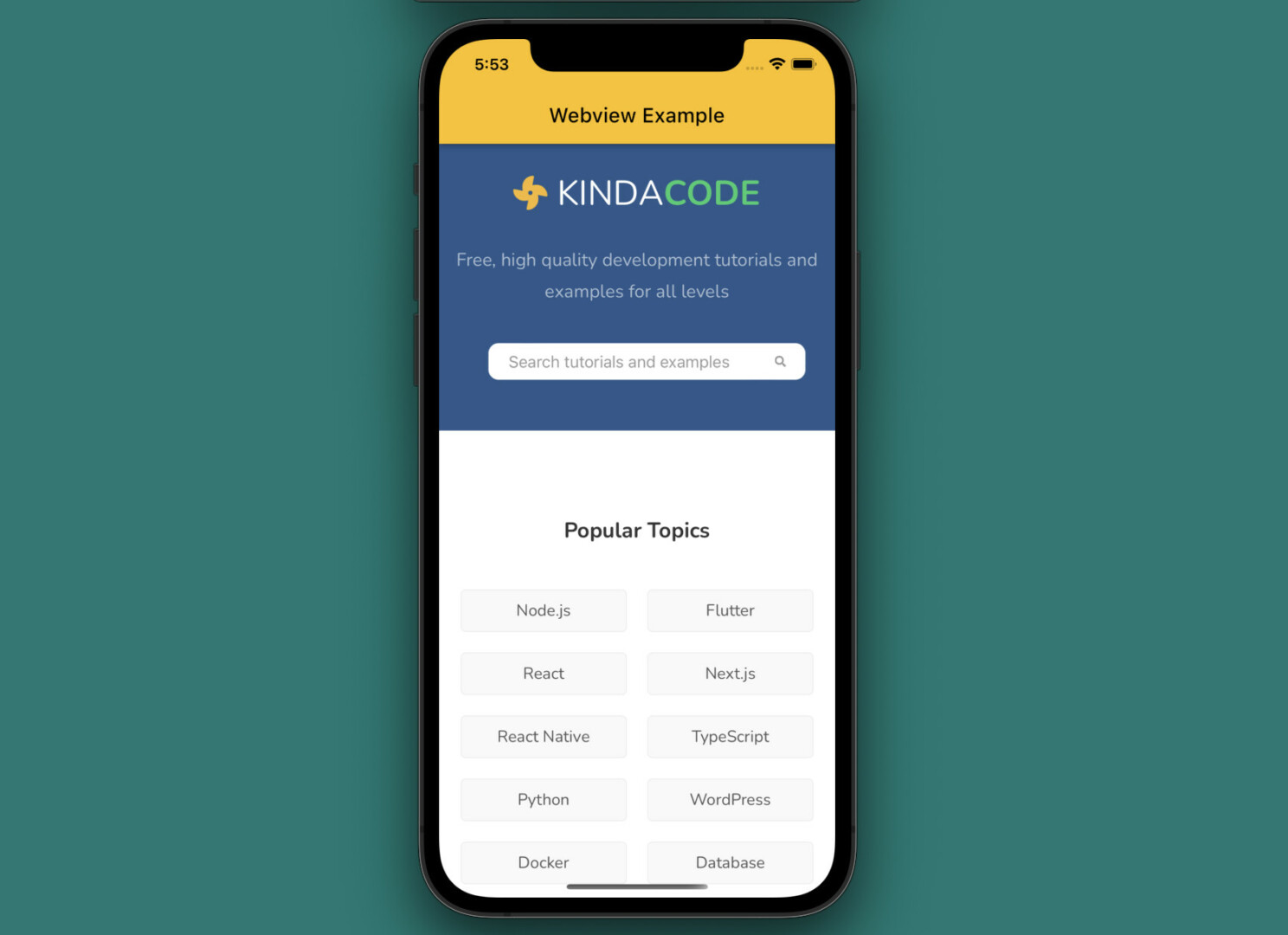
There are several packages that can help you implement a web view in your Flutter application. In this article, we’ll use webview_flutter, the most popular plugin for this kind of stuff.
Table of Contents
Install The Plugin
Run the following command to add the flutter_webview plugin to your project:
flutter pub add webview_flutter
This is an official plugin made by the Flutter team, and it is updated quite regularly (this tutorial uses the latest version of the plugin).
Implementation
Import webview_flutter into your Dart code:
import 'package:webview_flutter/webview_flutter.dart';
Then create a webview controller:
final _controller = WebViewController()
..setJavaScriptMode(JavaScriptMode.unrestricted)
..setNavigationDelegate(
NavigationDelegate(
onProgress: (int progress) {
// print the loading progress to the console
// you can use this value to show a progress bar if you want
debugPrint("Loading: $progress%");
},
onPageStarted: (String url) {},
onPageFinished: (String url) {},
onWebResourceError: (WebResourceError error) {},
onNavigationRequest: (NavigationRequest request) {
return NavigationDecision.navigate;
},
),
)
..loadRequest(Uri.parse("https://www.kindacode.com"));
Finally, use the controller with the WebViewWidget as shown below:
WebViewWidget(
controller: _controller,
)
The Complete Example
import 'package:flutter/material.dart';
// don't forget this line
import 'package:webview_flutter/webview_flutter.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
theme: ThemeData(primarySwatch: Colors.amber),
title: "Kindacode.comr",
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
MyHomePage({Key? key}) : super(key: key);
// Create a webview controller
final _controller = WebViewController()
..setJavaScriptMode(JavaScriptMode.unrestricted)
..setNavigationDelegate(
NavigationDelegate(
onProgress: (int progress) {
// print the loading progress to the console
// you can use this value to show a progress bar if you want
debugPrint("Loading: $progress%");
},
onPageStarted: (String url) {},
onPageFinished: (String url) {},
onWebResourceError: (WebResourceError error) {},
onNavigationRequest: (NavigationRequest request) {
return NavigationDecision.navigate;
},
),
)
..loadRequest(Uri.parse("https://www.kindacode.com"));
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Webview Example'),
),
body: SizedBox(
width: double.infinity,
// the most important part of this example
child: WebViewWidget(
controller: _controller,
)),
);
}
}
And here’s the result:
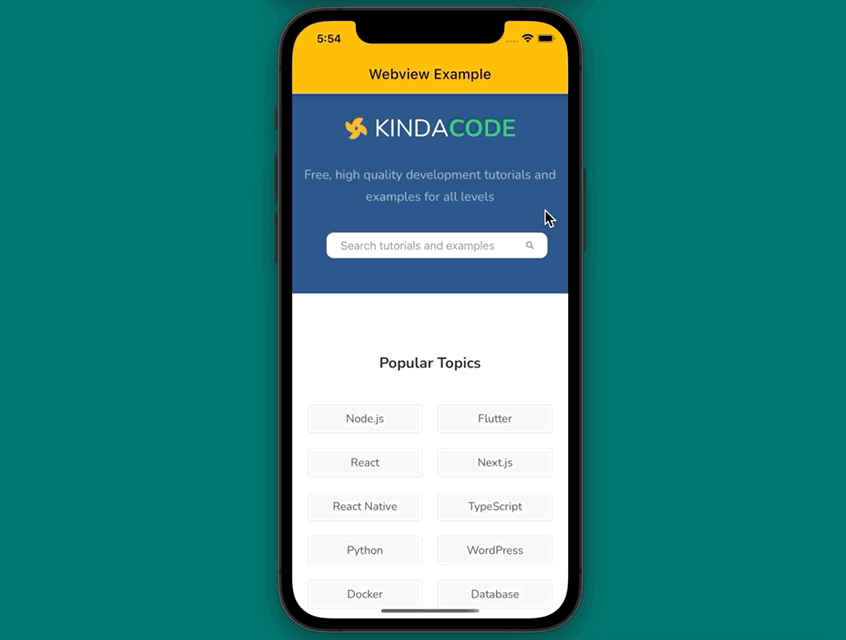
You you have a look at your console, you will see some output like this:
flutter: Loading: 10%
flutter: Loading: 30%
flutter: Loading: 76%
flutter: Loading: 100%
Conclusion
You’ve gone through a complete example of implementing a web view in an app. If you’d like to learn more new and interesting stuff about Flutter, take a look at the following articles:
- Displaying Subscripts and Superscripts in Flutter
- Flutter: 5 Ways to Add a Drop Shadow to a Widget
- Flutter + Firebase Storage: Upload, Retrieve, and Delete files
- Flutter: Make Text Clickable like Hyperlinks on the Web
- How to Programmatically Take Screenshots in Flutter
- Example of sortable DataTable in Flutter
You can also check out our Flutter category page, or Dart category page for the latest tutorials and examples.