In Flutter, you can access variables from the stateful widget class with the widget keyword like this:
class ChildPage extends StatefulWidget {
final String someText;
const ChildPage({Key? key, required this.someText}) : super(key: key);
@override
State<ChildPage> createState() => _ChildPageState();
}
class _ChildPageState extends State<ChildPage> {
@override
Widget build(BuildContext context) {
return Center(
child: Text(widget.someText),
);
}
}
But in some cases, you may need to get the variables from the stateful widget before Widget build(BuildContext context) runs. How to do so? The solution is to use the initState() method:
class ChildPage extends StatefulWidget {
final String someText;
const ChildPage({Key? key, required this.someText}) : super(key: key);
@override
State<ChildPage> createState() => _ChildPageState();
}
class _ChildPageState extends State<ChildPage> {
late String displayText;
@override
void initState() {
displayText = widget.someText + " " + "Some extra text!";
super.initState();
}
@override
Widget build(BuildContext context) {
return Center(
child: Text(displayText),
);
}
}
Complete Example
In this example, we will pass some text from a widget (MyHomePage) to a stateful widget (ChildPage).
Screenshot:
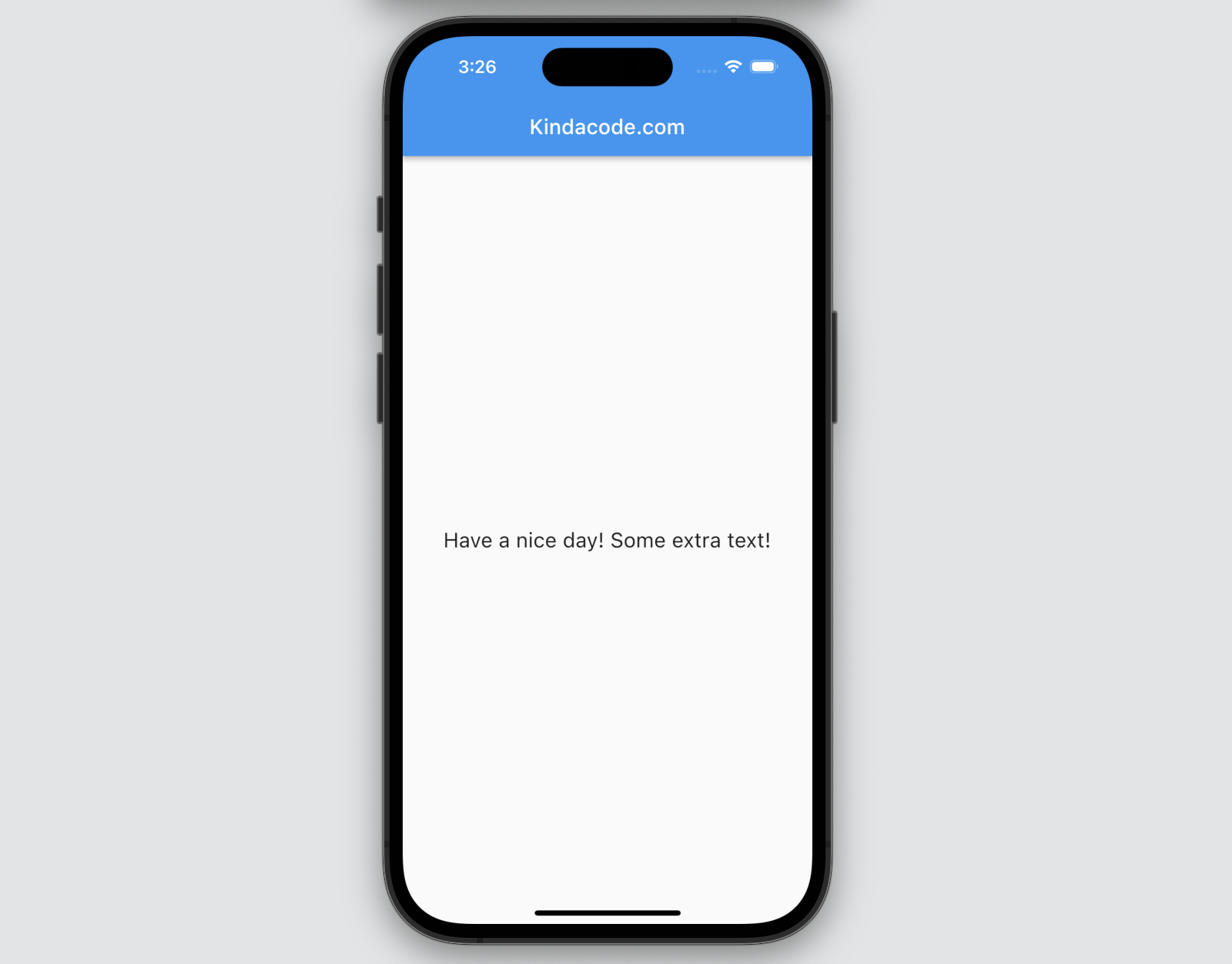
Here’s the full code in main.dart accompanied by explanations in the comments:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Hide the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: const ChildPage(
// Pass the text to the state class
someText: 'Have a nice day!',
),
);
}
}
class ChildPage extends StatefulWidget {
// This is the text that will be passed to the state class
final String someText;
const ChildPage({Key? key, required this.someText}) : super(key: key);
@override
State<ChildPage> createState() => _ChildPageState();
}
class _ChildPageState extends State<ChildPage> {
// This is the text that will be displayed
late String displayText;
@override
void initState() {
// Initialize the displayText variable
// with the variable "someText" from the ChildPage class
displayText = "${widget.someText} Some extra text!";
super.initState();
}
@override
Widget build(BuildContext context) {
return Center(
// Display the text
child: Text(
displayText,
style: const TextStyle(fontSize: 20),
),
);
}
}
Hope this helps. Further reading:
- Flutter: Columns with Percentage Widths
- Flutter: Make Text Clickable like Hyperlinks on the Web
- How to Add a Drop Shadow to Text in Flutter
- Flutter: Dismiss Keyboard when Tap Outside Text Field
- Flutter TextField: Styling labelText, hintText, and errorText
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.