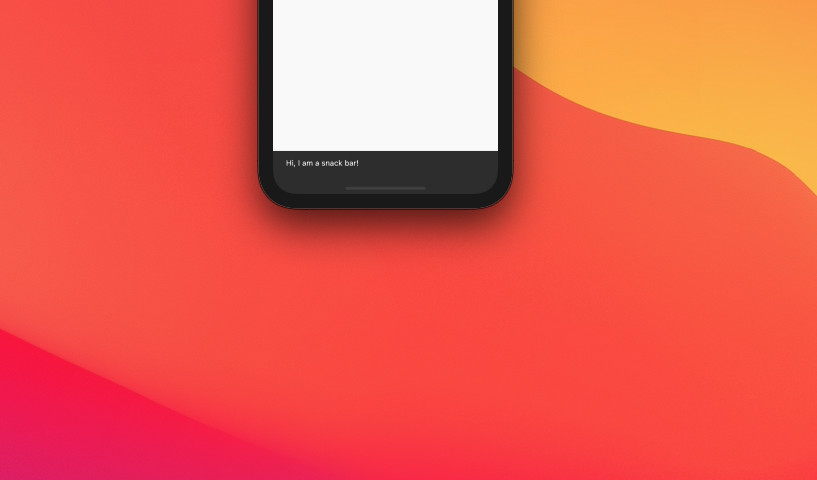
This article shows you how to use ScaffoldMessenger to display SnackBars in Flutter applications.
Overivew
ScaffoldMessenger provides APIs for displaying snack bars in a neat and simple way.
With the ScaffoldMessenger class, we can now call showSnackBar() inside or outside the build() function without worrying about context. Both implementations below are working fine.
1. Call showSnackBar() inside build():
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Kindacode.com'),
),
body: Center(
child: ElevatedButton(
onPressed: () {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: Text("Hi, I am a snack bar!"),
));
},
child: Text('Show SnackBar'),
),
),
);
}
2. Call showSnackBar() outside build():
void _show() {
ScaffoldMessenger.of(context).showSnackBar(SnackBar(
content: Text("Hi, I am a snack bar!"),
));
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Kindacode.com'),
),
body: Center(
child: ElevatedButton(
onPressed: _show,
child: Text('Show SnackBar'),
),
),
);
}
Complete Example
Preview
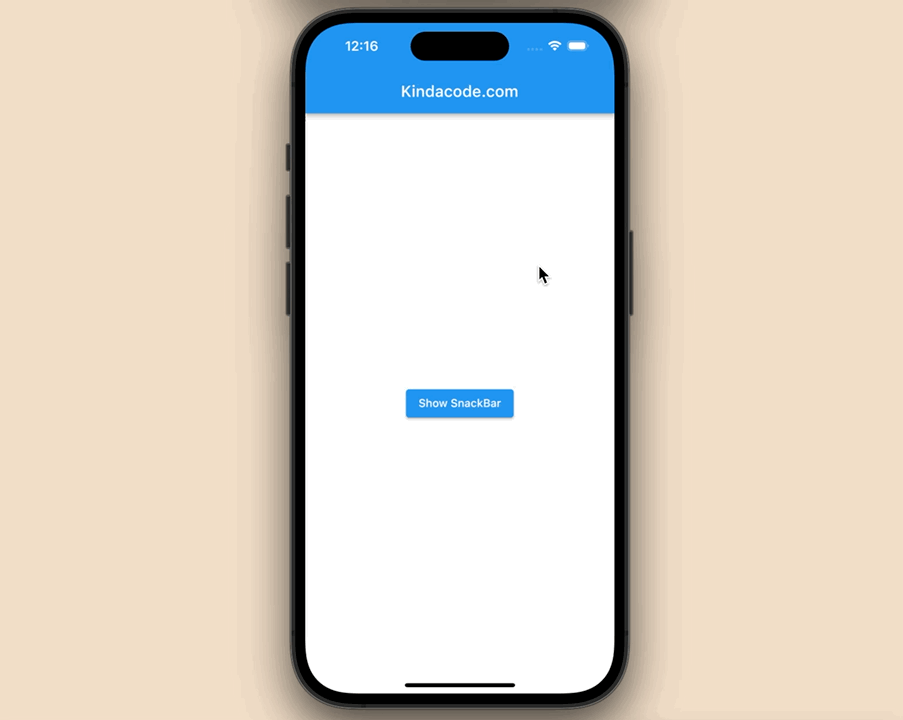
The full code
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
// Function to show the SnackBar
// This function will be called when the user clicks on the button
void _show() {
ScaffoldMessenger.of(context).showSnackBar(const SnackBar(
content: Text("Hi, I am a snack bar!"),
));
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Center(
child: ElevatedButton(
onPressed: _show,
child: const Text('Show SnackBar'),
),
),
);
}
}
The Old Days
Note: This section is for reference purposes or for those of you who are working on maintaining old code bases.
In past (Flutter 1.x), we used to display SnackBar with the 2 following approaches. The first one is using Scaffold.of(context).showSnacBar(/*…*/) like this:
Scaffold.of(context).showSnackBar(SnackBar(
content: Text('I am a snack bar!'),
));
The second way is showing SnackBar with a key, as shown below:
final GlobalKey<ScaffoldState> _scaffoldKey = GlobalKey();
void _show() {
_scaffoldKey.currentState.showSnackBar(SnackBar(
content: Text("Hello"),
));
}
Many Flutter newcomers are likely to encounter the error below when showing a snack bar with the old-fashioned approaches:
Scaffold.of() called with a context that does not contain a Scaffold
ScaffoldMessenger can help you get rid of this problem.
Conclusion
This article has walked you through a few aspects of using SnackBar in modern Flutter. If you would like to learn more exciting and new things about mobile development, take a look at the following articles:
- Flutter StreamBuilder examples
- Using AnimatedIcon in Flutter
- Example of sortable DataTable in Flutter
- Flutter: Make a “Scroll Back To Top” button
- React Native FlatList: Tutorial and Examples
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.