To set an image background for a Container widget in Flutter, we set its decoration property like this:
decoration: const BoxDecoration(
image: DecorationImage(
image: NetworkImage(/*...*/),
fit: BoxFit.cover,
repeat: ImageRepeat.noRepeat,
),
),
You can use either NetworkImage or AssetImage. If you have the plan to use AssetImage, you need to declare the images you are going to use in your pubspec.yaml file. If you aren’t familiar with this stuff, see the article using Local Images in Flutter.
Complete Example
Preview:
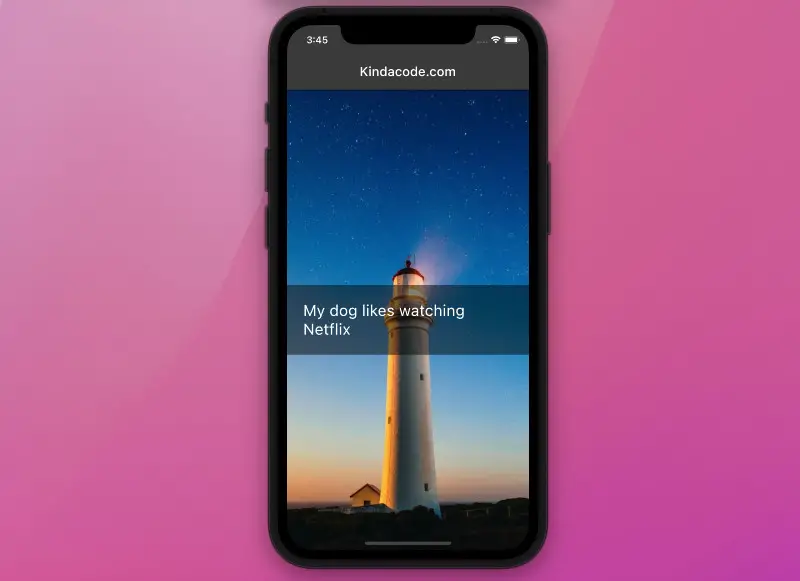
The beautiful background here is from Pixabay, a wonderful site to get free images.
The full code in main.dart:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Hide the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
theme: ThemeData.dark(),
home: const HomePage(),
);
}
}
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Center(
child: Container(
alignment: Alignment.center,
decoration: const BoxDecoration(
image: DecorationImage(
image: NetworkImage(
'https://www.kindacode.com/wp-content/uploads/2022/02/beach.jpeg'),
fit: BoxFit.cover,
repeat: ImageRepeat.noRepeat,
),
),
child: Container(
padding: const EdgeInsets.all(25),
color: Colors.black45,
child: const Text(
'My dog likes watching Netflix',
style: TextStyle(color: Colors.white, fontSize: 24),
)),
),
),
);
}
}
Hope this helps. Further reading:
- Flutter image loading builder example
- Flutter: Caching Network Images for Big Performance gains
- Flutter: Reading Bytes from a Network Image
- Flutter: Adding a Gradient Border to a Container (2 Examples)
- Example of using AnimatedContainer in Flutter
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.